https://github.com/freshOS/Router-deprecated
🛣 Simple Navigation for iOS - ⚠️ Deprecated
https://github.com/freshOS/Router-deprecated
freshos microframework navigation router routing swift viewcontroller
Last synced: 5 months ago
JSON representation
🛣 Simple Navigation for iOS - ⚠️ Deprecated
- Host: GitHub
- URL: https://github.com/freshOS/Router-deprecated
- Owner: freshOS
- License: mit
- Created: 2017-02-27T07:04:22.000Z (about 8 years ago)
- Default Branch: master
- Last Pushed: 2021-10-21T06:56:49.000Z (over 3 years ago)
- Last Synced: 2024-12-01T19:34:40.709Z (5 months ago)
- Topics: freshos, microframework, navigation, router, routing, swift, viewcontroller
- Language: Swift
- Homepage:
- Size: 2.83 MB
- Stars: 448
- Watchers: 15
- Forks: 26
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
- Code of conduct: CODE_OF_CONDUCT.md
Awesome Lists containing this project
- awesome-ios - Router - Simple Navigation for iOS. (App Routing)
README

⚠️ Deprecated and no longer supported. Use at your own risks.
This has been used extensively and after weighing up pros and cons, we now favour the use of native Flow Controllers or Coordinators as explained [here](https://www.hackingwithswift.com/articles/71/how-to-use-the-coordinator-pattern-in-ios-apps).
The native route (pun intended) should always be favoured and we believe less dependencies is something we should always strive for.# Router
[](https://developer.apple.com/swift)

[](https://github.com/Carthage/Carthage)
[](https://app.bitrise.io/app/11b4cd282c1d70b3)
[](https://github.com/freshOS/then/blob/master/LICENSE)
[Reason](#why) - [Get Started](#get-started) - [Installation](#installation)
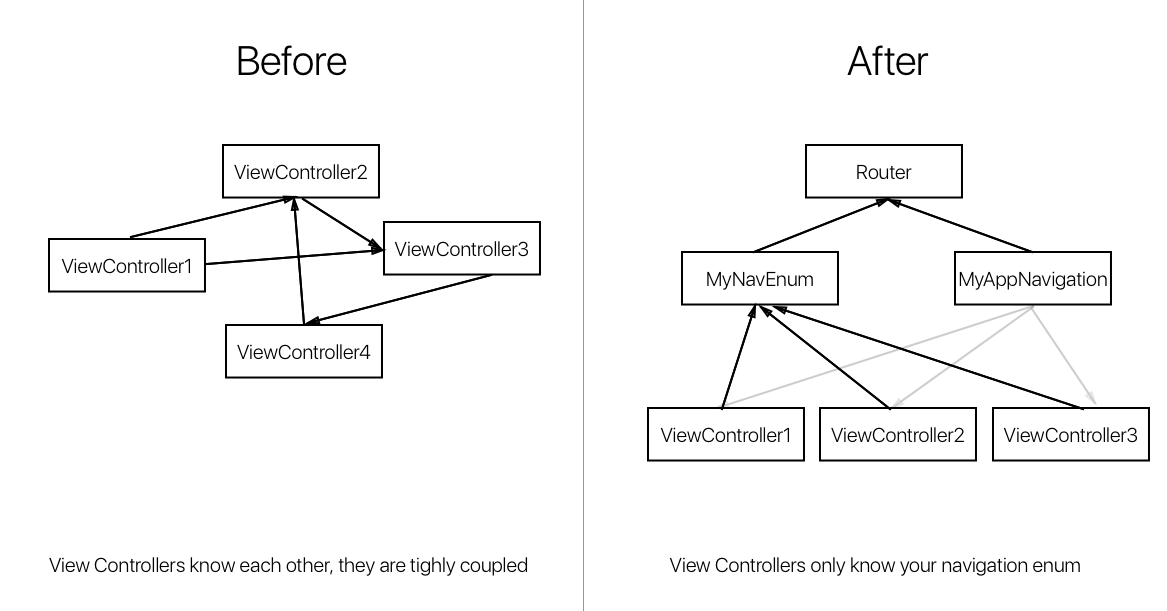
## Why
Because **classic App Navigation** introduces **tight coupling** between ViewControllers.
Complex Apps navigation can look like a **gigantic spider web**.Besides the fact that **Navigation responsibility is split** among ViewControllers, modifying a ViewController can cascade recompiles and produce **slow compile times.**
## How
By using a Navigation `enum` to navigate we decouple ViewControllers between them. Aka they don't know each other anymore. So modifying `VCA` won't trigger `VCB` to recompile anymore \o/```swift
// navigationController?.pushViewController(AboutViewController(), animated: true)
navigate(.about)
```Navigation code is now encapsulated in a `AppNavigation` object.
## Benefits
- [x] Decouples ViewControllers
- [x] Makes navigation Testable
- [x] Faster compile times## Get started
### 1 - Declare your Navigation enum
```swift
enum MyNavigation: Navigation {
case about
case profile(Person)
}
```
Swift enum can take params!
Awesome for us because that's how we will pass data between ViewControllers :)### 2 - Declare your App Navigation
```swift
struct MyAppNavigation: AppNavigation {func viewcontrollerForNavigation(navigation: Navigation) -> UIViewController {
if let navigation = navigation as? MyNavigation {
switch navigation {
case .about:
return AboutViewController()
case .profile(let p):
return ProfileViewController(person: p)
}
}
return UIViewController()
}func navigate(_ navigation: Navigation, from: UIViewController, to: UIViewController) {
from.navigationController?.pushViewController(to, animated: true)
}
}
```A cool thing is that the swift compiler will produce an error if a navigation
case is not handled ! Which would'nt be the case with string URLs by the way ;)### 3 - Register your navigation on App Launch
In `AppDelegate.swift`, before everything :
```swift
Router.default.setupAppNavigation(appNavigation: MyAppNavigation())
```### 4 - Replace navigations in your View Controllers
You can now call nagivations from you view controllers :
```swift
navigate(MyNavigation.about)
```Bridge `Navigation` with your own enum type, here `MyNavigation` so that we don't have to type our own.
```swift
extension UIViewController {func navigate(_ navigation: MyNavigation) {
navigate(navigation as Navigation)
}
}
```
You can now write :
```swift
navigate(.about)
```### Bonus - Tracking
Another cool thing about decoupling navigation is that you can now extract traking code from view Controllers as well. You can be notified by the router whenever a navigation happened.```swift
Router.default.didNavigate { navigation in
// Plug Analytics for instance
GoogleAnalitcs.trackPage(navigation)
}```
## Shave off compilation times
There is a nasty bug in Swift 3 compiler where the compiler rebuilds files even though they haven't changed.
This is documented here : https://forums.developer.apple.com/thread/62737?tstart=0Due to this bug, the compilation can go like this :
Change `ViewController1` -> `Build`
-> Compiles `ViewController1`, referenced in `MyAppNavigation` so
`MyAppNavigation` gets recompiled. `MyAppNavigation` is referenced in `AppDelegate` which gets recompiled which references ...
`App` -> `ViewController2` -> `ViewController3` -> `ViewControllerX` you get the point.
Before you know it the entire App gets rebuilt :/A good this is that most of the app coupling usually comes from navigation. which Router decouples.
We can stop this nonsense until this gets fixed in a future release of Xcode.
Router can help us manage this issue by injecting our AppNavigation implementation at runtime.In your `AppDelegate.swift`
```swift
// Inject your AppNavigation at runtime to avoid recompilation of AppDelegate :)
Router.default.setupAppNavigation(appNavigation: appNavigationFromString("YourAppName.MyAppNavigation"))
```And make sure your `AppNavigation` implementation is now a `class` that is `RuntimeInjectable`
```swift
class MyAppNavigation: RuntimeInjectable, AppNavigation {
```## Installation
#### Carthage
```
github "freshOS/Router"
```
#### Manually
Simply Copy and Paste `Router.swift` files in your Xcode Project :)#### As A Framework
Grab this repository and build the Framework target on the example project. Then Link against this framework.### Backers
Like the project? Offer coffee or support us with a monthly donation and help us continue our activities :)### Sponsors
Become a sponsor and get your logo on our README on Github with a link to your site :)