Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/fxnai/fxnios
Run Python functions in iOS apps. Register at https://fxn.ai
https://github.com/fxnai/fxnios
Last synced: about 2 months ago
JSON representation
Run Python functions in iOS apps. Register at https://fxn.ai
- Host: GitHub
- URL: https://github.com/fxnai/fxnios
- Owner: fxnai
- License: apache-2.0
- Created: 2023-10-22T02:12:41.000Z (about 1 year ago)
- Default Branch: main
- Last Pushed: 2024-11-14T14:02:25.000Z (about 2 months ago)
- Last Synced: 2024-11-14T15:18:53.178Z (about 2 months ago)
- Language: Swift
- Homepage: https://docs.fxn.ai
- Size: 88.9 KB
- Stars: 0
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
- Code of conduct: CODE_OF_CONDUCT.md
Awesome Lists containing this project
README
# Function for iOS
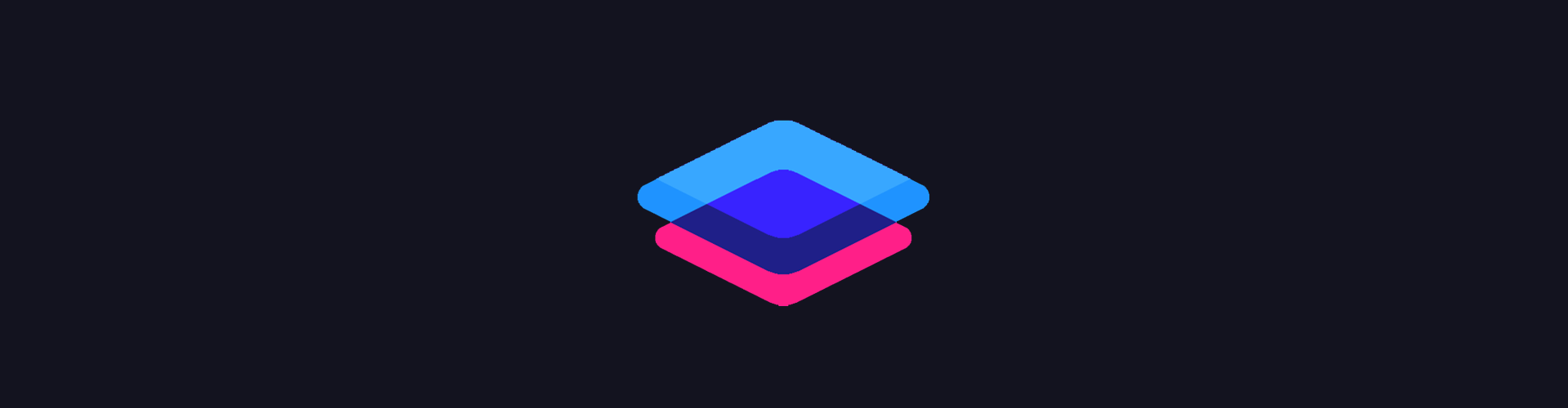
[](https://fxn.ai/community)
Run Python functions (a.k.a "predictors") locally in your iOS apps, with
full GPU acceleration and zero dependencies.> [!TIP]
> [Join our waitlist](https://fxn.ai/waitlist) to bring your custom Python functions and run them on-device across Android, iOS, macOS, Linux, web, and Windows.> [!NOTE]
> Function requires iOS 15+.## Installing Function
Function is distributed as a SwiftPM package, and can be added as a dependency in an Xcode project or in a SwiftPM package:### In Xcode
In your project editor, open the `Package Dependencies` tab, search
for https://github.com/fxnai/fxnios.git and add the package to your project:
### In Swift Package Manager
Add the following dependency to your `Package.swift` file:
```swift
let package = Package(
name: "MyAwesomeApp",
dependencies: [
.package(url: "https://github.com/fxnai/fxnios.git", from: "0.0.1"),
],
targets: [
.target(name: "MyAwesomeApp", dependencies: ["FunctionSwift"]),
]
)
```## Retrieving your Access Key
Before creating predictions, you will need to [create a Function account](https://fxn.ai).
Once you do, generate an access key: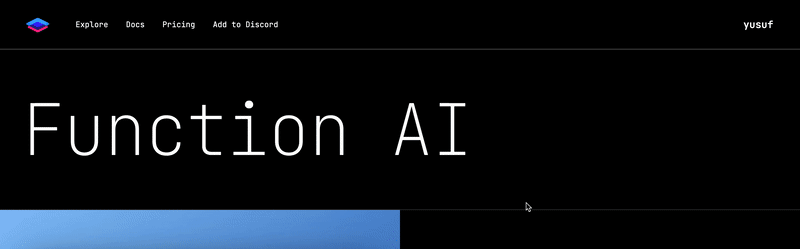
Next, create an `fxn.xcconfig` build configuration file and enter your access key:
```bash
# Function access key
FXN_ACCESS_KEY=""
```> [!CAUTION]
> Make sure that your `fxn.xcconfig` file is excluded from source control by adding it to your `.gitignore` file. **Never share your access key**.## Making a Prediction
First, create a Function client:
```swift
import FunctionSwift// 💥 Create a Function client
let fxn = Function(accessKey: "...")
```
Then make a prediction:
```swift
// 🔥 Make a prediction
let prediction = try await fxn.predictions.create(
tag: "@fxn/greeting",
inputs: ["name": "Sam"]
)
```
Finally, use the prediction results:
```swift
// 🚀 Use the results
print("Prediction result: \(prediction.results![0]!)")
```## Embedding Predictors
Function normally works by downloading and executing prediction functions at runtime. But because iOS requires
strict sandboxing, you must download and embed predictors at build-time instead.
First, create an `fxn.config.swift` file at the root of your target directory:
```swift
import FunctionSwiftlet config = Function.Configuration(
// add all predictor tags to be embedded here
tags: [
"@fxn/greeting"
]
)
```Next, right click on your project and run the `Embed Predictors` command on your app target:

Function will download the prediction function as a dynamic framework, then configure Xcode to embed the framework into your app bundle.
> [!NOTE]
> The `Embed Predictors` script requires internet and file system access to download and embed the prediction function into your Xcode project.> [!IMPORTANT]
> After embedding, Xcode might prompt you to either reload the project from disk or keep the current version in memory. **Always reload your project from disk**.## Useful Links
- [Discover predictors to use in your apps](https://fxn.ai/explore).
- [Join our Discord community](https://discord.gg/fxn).
- [Check out our docs](https://docs.fxn.ai).
- Learn more about us [on our blog](https://blog.fxn.ai).
- Reach out to us at [[email protected]](mailto:[email protected]).Function is a product of [NatML Inc](https://github.com/natmlx).