Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/heavy-duty/znap
Performance-first Rust Framework to build APIs compatible with the Solana Actions Spec.
https://github.com/heavy-duty/znap
Last synced: 15 days ago
JSON representation
Performance-first Rust Framework to build APIs compatible with the Solana Actions Spec.
- Host: GitHub
- URL: https://github.com/heavy-duty/znap
- Owner: heavy-duty
- License: apache-2.0
- Created: 2024-06-13T19:56:43.000Z (6 months ago)
- Default Branch: master
- Last Pushed: 2024-10-29T20:27:43.000Z (about 1 month ago)
- Last Synced: 2024-10-29T22:47:39.433Z (about 1 month ago)
- Language: Rust
- Homepage:
- Size: 24.4 MB
- Stars: 63
- Watchers: 3
- Forks: 2
- Open Issues: 13
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
- awesome-blinks - Znap - Framework to build APIs compatible with the Solana Actions spec. (Developer Tools)
- awesome-blinks - Znap - Framework to build APIs compatible with the Solana Actions spec. (Developer Tools)
README
[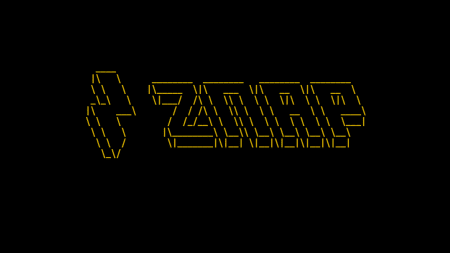](https://youtu.be/pmuwP9fWa3M)
Znap
[Watch the demo](https://youtu.be/pmuwP9fWa3M)
Performance-first Rust Framework to build APIs compatible with the Solana Actions Spec.
Znap is an innovative Rust-based framework designed to simplify the creation of [Solana Actions](#) on the Solana blockchain.
- Rust eDSL for writing Solana actions
- Macro collection
- CLI and workspace management for developing complete Solana actionsIf you're familiar with developing using the Anchor framework, then the experience will be familiar.
## Getting Started
1. `cargo install znap-cli`
2. `znap init `
3. `cd `
4. `znap new `## Packages
| Package | Description | Version | Docs |
| :---------------------- | :------------------------------------------------------- | :------------------------------------------------------------------------------------------------------------------------------- | :-------------------------------------------------------------------------------------------------------------- |
| `znap` | Znap framework's core library to create Solana actions | [](https://crates.io/crates/znap) | [](https://docs.rs/znap/latest/znap/) |
| `znap-syn` | Parsing and generating code for macros in Rust | [](https://crates.io/crates/znap-syn) | [](https://docs.rs/znap-syn/latest/znap_syn/) |
| `znap-macros` | Macro collection for creating Solana actions | [](https://crates.io/crates/znap-macros) | [](https://docs.rs/znap-macros/latest/znap_macros/) |
| `znap-cli` | Znap CLI to interact with a znap workspace. | [](https://crates.io/crates/znap-cli) | [](https://docs.rs/znap-cli/latest/znap_cli/) |## Example
```rust
use solana_sdk::{
message::Message, native_token::LAMPORTS_PER_SOL, pubkey::Pubkey, system_instruction::transfer,
transaction::Transaction,
};
use std::str::FromStr;
use znap::prelude::*;#[collection]
pub mod my_actions {
use super::*;pub fn send_donation(ctx: Context) -> Result {
let account_pubkey = Pubkey::from_str(&ctx.payload.account)
.or_else(|_| Err(Error::from(ActionError::InvalidAccountPublicKey)))?;
let receiver_pubkey = Pubkey::from_str(&ctx.params.receiver_address)
.or_else(|_| Err(Error::from(ActionError::InvalidReceiverPublicKey)))?;
let transfer_instruction = transfer(
&account_pubkey,
&receiver_pubkey,
ctx.query.amount * LAMPORTS_PER_SOL,
);
let transaction_message = Message::new(&[transfer_instruction], None);
let transaction = Transaction::new_unsigned(transaction_message);Ok(ActionTransaction {
transaction,
message: Some("send donation to alice".to_string()),
})
}
}#[derive(Action)]
#[action(
icon = "https://media.discordapp.net/attachments/1205590693041541181/1212566609202520065/icon.png?ex=667eb568&is=667d63e8&hm=0f247078545828c0a5cf8300a5601c56bbc9b59d3d87a0c74b082df0f3a6d6bd&=&format=webp&quality=lossless&width=660&height=660",
title = "Send a Donation to {{params.receiver_address}}",
description = "Send a donation to {{params.receiver_address}} using the Solana blockchain via a Blink.",
label = "Send",
link = {
label = "Send 1 SOL",
href = "/api/send_donation/{{params.receiver_address}}?amount=1",
},
link = {
label = "Send 5 SOL",
href = "/api/send_donation/{{params.receiver_address}}?amount=5",
},
link = {
label = "Send SOL",
href = "/api/send_donation/{{params.receiver_address}}?amount={amount}",
parameter = { label = "Amount in SOL", name = "amount" }
},
)]
#[query(amount: u64)]
#[params(receiver_address: String)]
pub struct SendDonationAction;#[derive(ErrorCode)]
enum ActionError {
#[error(msg = "Invalid account public key")]
InvalidAccountPublicKey,
#[error(msg = "Invalid receiver public key")]
InvalidReceiverPublicKey,
}
```