Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/herbou/unityplayerprefsextra
Unity PlayerPrefsExtra gives you the ability to save more complexe data types such as : Vectors, Bool, Colors, Lists, ... and it uses the Unity's PlayerPrefs behind the scene.
https://github.com/herbou/unityplayerprefsextra
playerprefs unity unity-playerprefs unity-playerprefsextra unity-scripts unitypackage
Last synced: 11 days ago
JSON representation
Unity PlayerPrefsExtra gives you the ability to save more complexe data types such as : Vectors, Bool, Colors, Lists, ... and it uses the Unity's PlayerPrefs behind the scene.
- Host: GitHub
- URL: https://github.com/herbou/unityplayerprefsextra
- Owner: herbou
- License: mit
- Created: 2020-06-27T15:51:25.000Z (over 4 years ago)
- Default Branch: master
- Last Pushed: 2023-02-25T08:06:11.000Z (almost 2 years ago)
- Last Synced: 2023-03-04T15:29:14.567Z (almost 2 years ago)
- Topics: playerprefs, unity, unity-playerprefs, unity-playerprefsextra, unity-scripts, unitypackage
- Language: C#
- Homepage:
- Size: 45.9 KB
- Stars: 41
- Watchers: 2
- Forks: 7
- Open Issues: 3
-
Metadata Files:
- Readme: README.md
- Funding: .github/FUNDING.yml
Awesome Lists containing this project
README
# Unity PlayerPrefsExtra
### Video tutorial : [https://youtu.be/wqRYdFcfiVw](https://youtu.be/wqRYdFcfiVw)
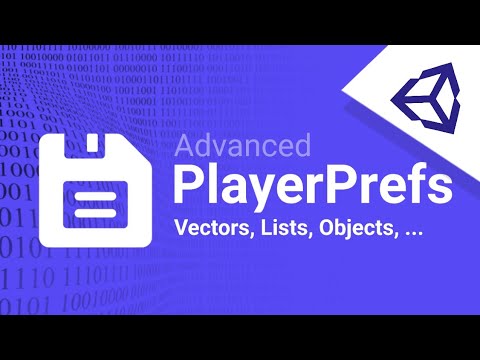Unity PlayerPrefsExtra gives you the ability to save more complexe data types such as :
Vectors, Bool, Colors, Lists, ...
and it uses the Unity's PlayerPrefs under the hood.# ⁉ How to use :
Use the same syntaxe as PlayerPrefs.## ■ Booleans :
```c#
//Load
bool b = PlayerPrefsExtra.GetBool("mybool", false);//Update (flip value)
b = !b;//Save
PlayerPrefsExtra.SetBool("mybool", b);
```
## ■ Vectors ( Vector2, Vector3, and Vector4 ):
```c#
//Load
Vector2 v = PlayerPrefsExtra.GetVector2("myV2", Vector2.zero);//Update
v+=Vector2.one;//Save
PlayerPrefsExtra.SetVector2("myV2", v);
```
## ■ Colors :
```c#
// Get color
Color c = PlayerPrefsExtra.GetColor("Col");// Set color
PlayerPrefsExtra.SetColor("Col", Color.red);
```
## ■ Quaternions :
```c#
// Get Quaternion
Quaternion qua = PlayerPrefsExtra.GetQuaternion("q");//Set Quaternion
PlayerPrefsExtra.SetQuaternion("q", qua);
```
## ■ Lists :
```c#
// Get List
List list = PlayerPrefsExtra.GetList("myList", new List());// Add data to List
list.Add(Random.Range(100,900);// Save List
PlayerPrefsExtra.SetList("myList", list);
```
## ■ Objects :
```c#
//Class
[System.Serializable]
public class Shape{
public int totalPoints = 3;
public float strokeWidth = 0f;
public List points = new List();
}
```
```c#
// Get object
Shape s = PlayerPrefsExtra.GetObject("myShape", new Shape());// Update object data
s.strokeWidth++;
s.points.Add(Vector3.one*Random.Range(0f,3f));// Save object
PlayerPrefsExtra.SetObject("myShape", s);
```
## ■ Delete All Keys (both PlayerPrefs & PlayerPrefsExtra) :
use PlayerPrefs instead of PlayerPrefsExtra
```c#
PlayerPrefs.DeleteAll();
```
## ■ Delete one key :
use PlayerPrefs instead of PlayerPrefsExtra
```c#
PlayerPrefs.DeleteKey("Key");
```
## ■ Check key existance :
use PlayerPrefs instead of PlayerPrefsExtra
```c#
PlayerPrefs.HasKey("Key");
```