https://github.com/hiradary/reoverlay
The missing solution for managing modals in React.
https://github.com/hiradary/reoverlay
modal overlay react react-modal react-overlay reoverlay
Last synced: 26 days ago
JSON representation
The missing solution for managing modals in React.
- Host: GitHub
- URL: https://github.com/hiradary/reoverlay
- Owner: hiradary
- License: mit
- Created: 2020-07-04T08:44:33.000Z (almost 5 years ago)
- Default Branch: master
- Last Pushed: 2023-03-05T04:00:56.000Z (about 2 years ago)
- Last Synced: 2025-04-02T05:08:36.424Z (about 1 month ago)
- Topics: modal, overlay, react, react-modal, react-overlay, reoverlay
- Language: JavaScript
- Homepage: https://hiradary.github.io/reoverlay
- Size: 44.8 MB
- Stars: 154
- Watchers: 2
- Forks: 8
- Open Issues: 16
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
- awesome-react-components - reoverlay - [demo](https://hiradary.github.io/reoverlay/) - The missing solution for managing modals. (UI Components / Overlay)
- awesome-react - reoverlay - The missing solution for managing modals.  (UI Components / Dialog/Modal/Alert)
- awesome-react-components - reoverlay - [demo](https://hiradary.github.io/reoverlay/) - The missing solution for managing modals. (UI Components / Overlay)
- fucking-awesome-react-components - reoverlay - π [demo](hiradary.github.io/reoverlay/) - The missing solution for managing modals. (UI Components / Overlay)
- awesome-react-components - reoverlay - [demo](https://hiradary.github.io/reoverlay/) - The missing solution for managing modals. (UI Components / Overlay)
- awesome-react-components - reoverlay - [demo](https://hiradary.github.io/reoverlay/) - The missing solution for managing modals. (UI Components / Overlay)
README
# Reoverlay




The missing solution for managing modals in React.
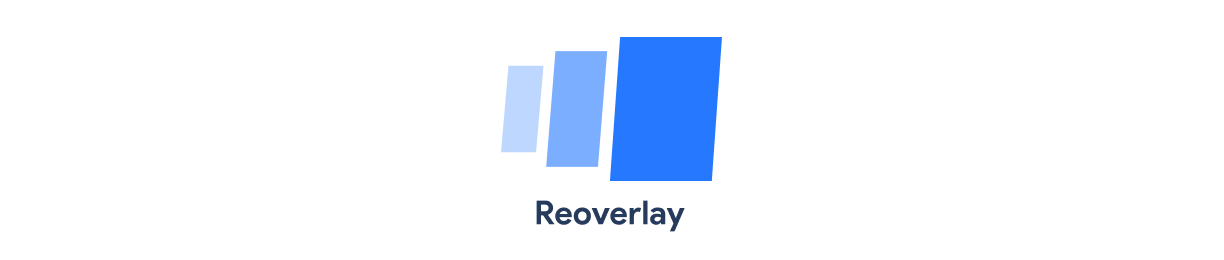
## Installation π₯
```bash
npm install reoverlay --save# or if you prefer Yarn:
yarn add reoverlay
```## Demo βοΈ
You can see a couple of examples on the [website](https://hiradary.github.io/reoverlay).
## Philosophy π
There are many ways you can manage your modals in React. You can ([See a relevant article](https://codeburst.io/modals-in-react-f6c3ff9f4701)):
- Use a modal component as a wrapper (like a button component) and include it wherever you trigger the hide/show of that modal.
- The βportalβ approach that takes a modal and attaches it to document.body.
- A top level modal component that shows different contents based on some property in the store.Each one of these has its own cons & pros. Take a look at the following example:
```javascript
const HomePage = () => {
const [isDeleteModalOpen, setDeleteModal] = useState(false)
const [isConfirmModalOpen, setConfirmModal] = useState(false)
return (
...
...
...
setDeleteModal(true)}>Show delete modal
setConfirmModal(true)}>Show confirm modal
)
}
```
This is the most commonly adopted approach. However, I believe it has a few drawbacks:
- You might find it difficult to show modals on top of each other. (aka "Stacked Modals")
- More boilerplate code. If you were to have 3 modals in a page, you had to use Modal component three times, declare more and more variables to handle visibility, etc.
- Unlike reoverlay, you can't manage your modals outside React scope (e.g Store). Though it's not generally a good practice to manage modals/overlays outside React scope, It comes in handy in some cases. (e.g Using axios interceptors to show modals according to network status, access control, etc.)Reoverlay, on the other hand, offers a rather more readable and easier approach. You'll be given a top-level modal component (`ModalContainer`), and a few APIs to handle triggering hide/show. Check [usage](#usage-) to see how it works.
## Usage π―
There are two ways you can use Reoverlay.
### #1 - Pass on your modals directly
`App.js`:
```javascript
import React from 'react';
import { ModalContainer } from 'reoverlay';const App = () => {
return (
<>
...
...
>
)
}
```
Later where you want to show your modal:
```javascript
import React from 'react';
import { Reoverlay } from 'reoverlay';import { ConfirmModal } from '../modals';
const PostPage = () => {
const deletePost = () => {
Reoverlay.showModal(ConfirmModal, {
text: "Are you sure you want to delete this post",
onConfirm: () => {
axios.delete(...)
}
})
}return (
This is your post page
Delete this post
)
}
```
Your modal file (`ConfirmModal` in this case):
```javascript
import React from 'react';
import { ModalWrapper, Reoverlay } from 'reoverlay';import 'reoverlay/lib/ModalWrapper.css';
const ConfirmModal = ({ confirmText, onConfirm }) => {
const closeModal = () => {
Reoverlay.hideModal();
}return (
{confirmText}
Yes
No
)
}
```
This is the simplest usage. If you don't want your modals to be passed directly to `Reoverlay.showModal(myModal)`, you could go on with the second approach.### #2 - Pass on your modal's name
`App.js`:
```javascript
import React from 'react';
import { Reoverlay, ModalContainer } from 'reoverlay';import { AuthModal, DeleteModal, ConfirmModal } from '../modals';
// Here you pass your modals to Reoverlay
Reoverlay.config([
{
name: "AuthModal",
component: AuthModal
},
{
name: "DeleteModal",
component: DeleteModal
},
{
name: "ConfirmModal",
component: ConfirmModal
}
])const App = () => {
return (
<>
...
...
>
)
}
```Later where you want to show your modal:
```javascript
import React from 'react';
import { Reoverlay } from 'reoverlay';const PostPage = () => {
const deletePost = () => {
Reoverlay.showModal("ConfirmModal", {
confirmText: "Are you sure you want to delete this post",
onConfirm: () => {
axios.delete(...)
}
})
}return (
This is your post page
Delete this post
)
}
```
Your modal file: (`ConfirmModal` in this case):
```javascript
import React from 'react';
import { ModalWrapper, Reoverlay } from 'reoverlay';import 'reoverlay/lib/ModalWrapper.css';
const ConfirmModal = ({ confirmText, onConfirm }) => {
const closeModal = () => {
Reoverlay.hideModal();
}return (
{confirmText}
Yes
No
)
}
```
NOTE: Using `ModalWrapper` is optional. It's just a half-transparent full-screen div, with a few preset animation options. You can create and use your own ModalWrapper. In that case, you can fully customize animation, responsiveness, etc. Check the code for [ModalWrapper](https://github.com/hiradary/reoverlay/blob/master/src/ModalWrapper.js).## Props β
### Reoverlay methods
##### `config(configData)`
| Name | Type | Default | Descripiton |
|------------|------------------------------------------------|---------|-------------------------------------|
| configData | `Array<{ name: string, component: React.FC }>` | `[]` | An array of modals along with their name. |This method must be called in the entry part of your application (e.g `App.js`), or basically before you attempt to show any modal. It takes an array of objects, containing data about your modals.
```javascript
import { AuthModal, DeleteModal, PostModal } from '../modals';Reoverlay.config([
{
name: 'AuthModal',
component: AuthModal
},
{
name: 'DeleteModal',
component: DeleteModal
},
{
name: 'PostModal',
component: PostModal
}
])
```
NOTE: If you're code-splitting your app and you don't want to import all modals in the entry part, you don't need to use this. Please refer to [usage](#usage-) for more info.##### `showModal(modal, props)`
| Name | Type | Default | Descripiton |
|---------|-----------------------|---------|-------------------------------------------------------------------------------------------------------------|
| modal | `string` \| `React.FC`| `null` | Either your modal's name (in case you've already passed that to `Reoverlay.config()`) or your modal component.|
| props | `object` | `{}` | Optional |```javascript
import { Reoverlay } from 'reoverlay';
import { MyModal } from '../modals';const MyPage = () => {
const showModal = () => {
Reoverlay.showModal(MyModal);
// or Reoverlay.showModal("MyModal")
}return (
Show modal
)
}
```##### `hideModal(modalName)`
| Name | Type | Default | Descripiton |
|-------------|----------|-----------|---------------------------------------------------------------------------------------|
| modalName | `string` | `null` | Optional. Specifies which modal gets hidden. By default, the last visible modal gets hidden. |```javascript
import { Reoverlay, ModalWrapper } from 'reoverlay';const MyModal = () => {
const closeModal = () => {
Reoverlay.hideModal();
}return (
My modal content...
Close modal
)
}const MyPage = () => {
const showModal = () => {
Reoverlay.showModal(MyModal);
}return (
Show modal
)
}
```##### `hideAll()`
This comes in handy when dealing with multiple modals on top of each other (aka "Stacked Modals"). With this, you can hide all modals at once.### `ModalWrapper` props
| Name | Type | Default | Descripiton |
|---------------------------|-------------------------------------------------------------------------------------------------------------|-------------------------------|----------------------------------------------------------|
| wrapperClassName | `string` | `''` | Additional CSS class for modal wrapper element. |
| contentContainerClassName | `string` | `''` | Additional CSS class for modal content container element. |
| animation | `'fade'` \| `'zoom'` \| `'flip'` \| `'door'` \| `'rotate'` \| `'slideUp'` \| `'slideDown'` \| `'slideLeft'` \| `'slideRight'` | `'fade'` | A preset of various animations for your modal. |
| onClose | `function` | `() => Reoverlay.hideModal()` | Gets called when the user clicks outside modal content. |## Support β€οΈ
PRs are welcome! You can also [buy me a coffee](https://buymeacoffee.com/hiradary) if you wish.
## LICENSE
[MIT](LICENSE)