Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/ilkeresen/selenium-webtest-apitest_case
Selenium Api Test (https://reqres.in/) Web Test (https://demoqa.com/) / Netaş Bootcamp Programı Kapsamında / Test Otomasyon
https://github.com/ilkeresen/selenium-webtest-apitest_case
delete enums get java json libraries post rest-api selenium selenium-java webdriver xpath
Last synced: 13 days ago
JSON representation
Selenium Api Test (https://reqres.in/) Web Test (https://demoqa.com/) / Netaş Bootcamp Programı Kapsamında / Test Otomasyon
- Host: GitHub
- URL: https://github.com/ilkeresen/selenium-webtest-apitest_case
- Owner: ilkeresen
- Created: 2023-01-27T00:04:29.000Z (about 2 years ago)
- Default Branch: main
- Last Pushed: 2023-01-28T01:04:02.000Z (about 2 years ago)
- Last Synced: 2025-01-23T19:37:08.061Z (22 days ago)
- Topics: delete, enums, get, java, json, libraries, post, rest-api, selenium, selenium-java, webdriver, xpath
- Language: Java
- Homepage:
- Size: 21.5 KB
- Stars: 1
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# ApiTest GET,POST,DELETE (https://reqres.in/)
pom.xml içerisine eklenmesi gereken dependencies
```javascript
org.seleniumhq.selenium
selenium-java
4.2.1
io.rest-assured
rest-assured
4.3.0
com.googlecode.json-simple
json-simple
1.1.1
```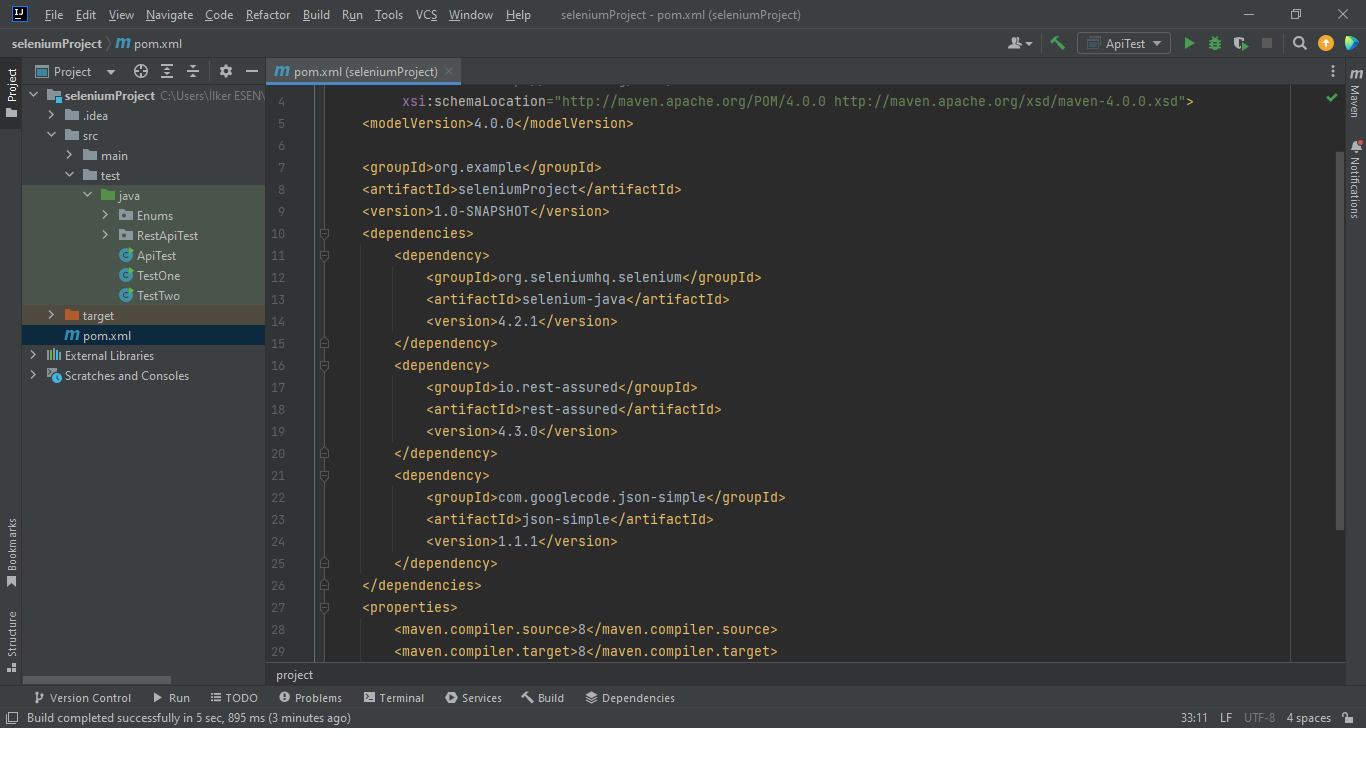
GetApiTest()
```javascript
package RestApiTest;import io.restassured.RestAssured;
import io.restassured.response.Response;public class Get {
public static void GetApiTest(){Response response = RestAssured.get("https://reqres.in/api/users?page=2");
//Http status code / (100 - 599) arasında dönen kod
System.out.println(response.statusCode());
System.out.println(response.asString());
//getBody All data get / Data'nın tamamını alır
System.out.println(response.getBody().asString());
//One data get / Tüm data içerisinden sadece first_name alır
String first_name = response.jsonPath().getString("data.first_name");
System.out.println(first_name);
/*
* Http status line / Bir Yanıt mesajının ilk satırı,
* her öğenin SP karakterleriyle ayrıldığı sayısal bir durum
* kodu ve bununla ilişkili metin cümlesinin takip ettiği protokol
* versiyonundan oluşan Durum Satırıdır.
*/
System.out.println(response.statusLine());}
}```
GetApiTest() ->Run
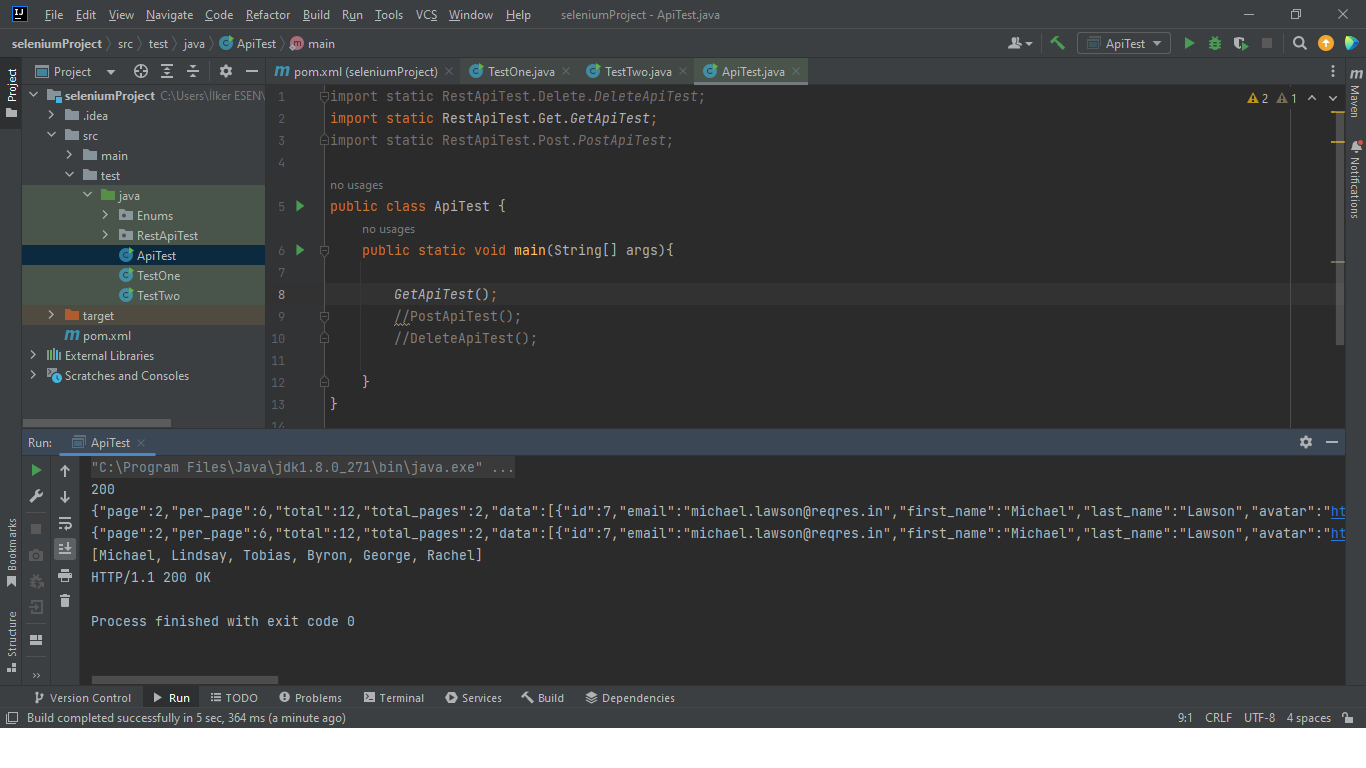
PostApiTest()
```javascript
package RestApiTest;import io.restassured.RestAssured;
import io.restassured.http.ContentType;
import org.json.simple.JSONObject;import static io.restassured.RestAssured.given;
public class Post {
public static void PostApiTest(){JSONObject request = new JSONObject();
request.put("name", "İlker");
request.put("job", "Senior Test Automation Development Engineer");RestAssured
.given()
.contentType(ContentType.JSON)
.body(request.toString())
.log().method()
.log().uri()
.log().body().when()
.post("https://reqres.in/api/users").then()
.assertThat().statusCode(201)
.log().body();}
}```
PostApiTest() ->Run
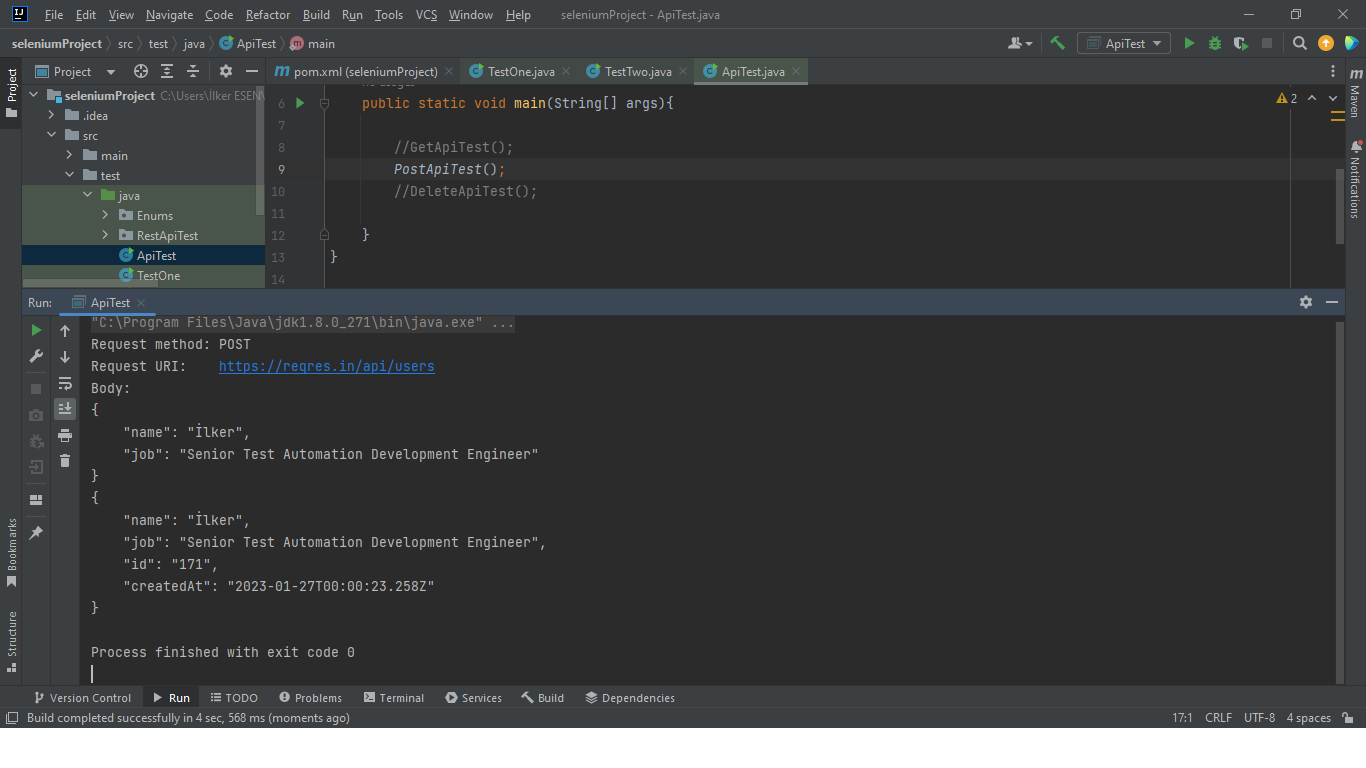
DeleteApiTest()
```javascript
package RestApiTest;import org.json.simple.JSONObject;
import static io.restassured.RestAssured.given;
public class Delete {
public static void DeleteApiTest(){JSONObject request = new JSONObject();
given().
body(request.toJSONString()).
when().
delete("https://reqres.in/api/users/2").
then().statusCode(204).
log().all();}
}```