Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/iminside/candy-loader
Load css files as pure jsx components with classnames as boolean props
https://github.com/iminside/candy-loader
css jsx react webpack webpack-loader
Last synced: 3 months ago
JSON representation
Load css files as pure jsx components with classnames as boolean props
- Host: GitHub
- URL: https://github.com/iminside/candy-loader
- Owner: iminside
- License: mit
- Created: 2022-02-28T11:57:15.000Z (almost 3 years ago)
- Default Branch: master
- Last Pushed: 2022-03-18T14:18:52.000Z (almost 3 years ago)
- Last Synced: 2024-09-27T19:41:03.554Z (3 months ago)
- Topics: css, jsx, react, webpack, webpack-loader
- Language: TypeScript
- Homepage:
- Size: 3.96 MB
- Stars: 7
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
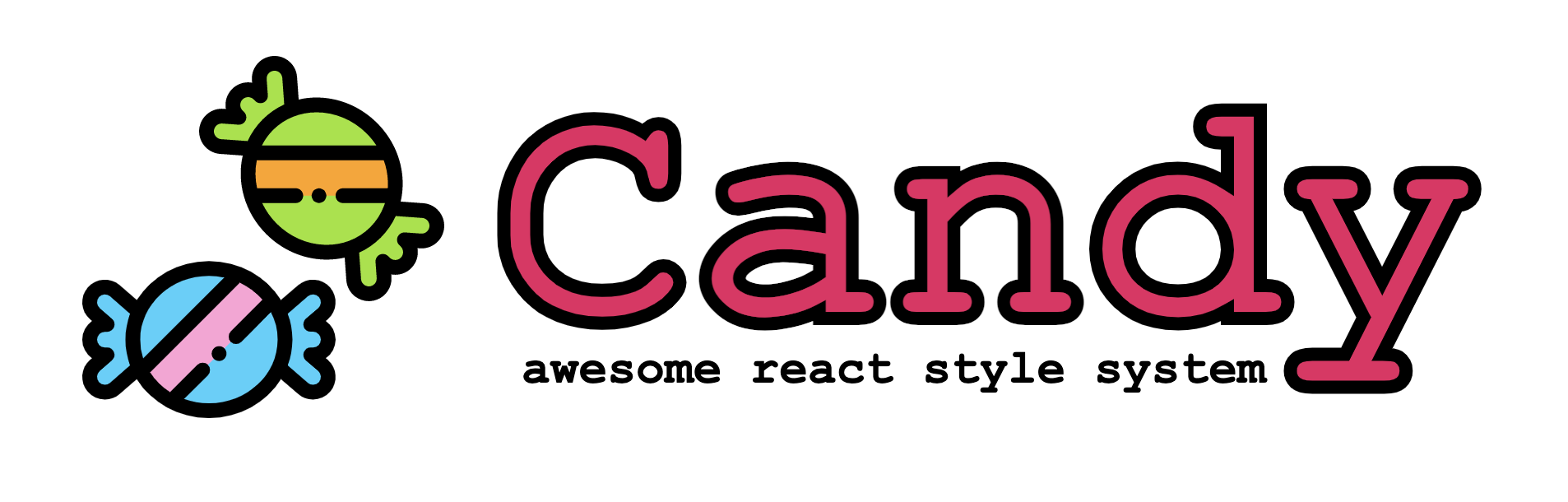
![]()
![]()
![]()
# Webpack Candy loader
Load css files as pure react jsx components with classnames as boolean props
## Install
```bash
npm i -D candy-loader
```## Settings
Update the loaders in your `webpack.config.js` file.
```js
{
rules: [
{
test: /\.css$/,
use: ['style-loader', 'candy-loader'],
},
],
}
```## Usage
Use classnames in camelCase mode
```css
/* style.css */.badge {
color: white;
}
.coral {
background-color: coral;
}
.green {
background-color: green;
}
```Import any html tag as pure jsx-component from css file
```tsx
import { Div } from './style.css'interface BadgeProps {
color: 'coral' | 'green'
}const Badge = (props: BadgeProps) => {
const isCoral = props.color === 'coral'
const isGreen = props.color === 'green'return (
Badge
)
}
```## Imports
You can include css files and access their styles.
```css
/* styles.css */
@import 'grid.css';.root {
/*...*/
}
``````tsx
import { Div } from './styles.css'function Component(props) {
return (
...
)
}
```## Pass css-variables
If a property starts with a double underscore, then its value can be retrieved using `var()` on any class applied to the element.
```tsx
import { Div } from './styles.css'function Component(props) {
return (
John
)
}
``````css
.name {
color: black;
font-size: var(--fontSize);
}
```## Get styles like css-modules
```css
.box {
width: 50px;
height: 50px;
}
``````tsx
import styles from './styles.css'function Box(props) {
return...
}
```## Based on `postcss`
You can use the usual postcss config file
```js
module.exports = {
plugins: {
autoprefixer: isProduction,
},
processOptions: {
map: isDevelopment,
},
}
```## Intellisense
Use [`typescript-plugin-candy`](https://github.com/iminside/typescript-plugin-candy) for type checking & autocomplete