Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/inevitable-dao/klaytn-multicall
🥁 Batch contract/on-chain queries to the same block. Multicall SDK for the Klaytn blockchain.
https://github.com/inevitable-dao/klaytn-multicall
caver-js klaytn
Last synced: about 2 months ago
JSON representation
🥁 Batch contract/on-chain queries to the same block. Multicall SDK for the Klaytn blockchain.
- Host: GitHub
- URL: https://github.com/inevitable-dao/klaytn-multicall
- Owner: inevitable-dao
- License: mit
- Created: 2022-10-13T07:20:57.000Z (almost 2 years ago)
- Default Branch: main
- Last Pushed: 2023-04-03T14:54:58.000Z (over 1 year ago)
- Last Synced: 2024-07-31T10:01:32.706Z (about 2 months ago)
- Topics: caver-js, klaytn
- Language: TypeScript
- Homepage:
- Size: 618 KB
- Stars: 6
- Watchers: 2
- Forks: 0
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
[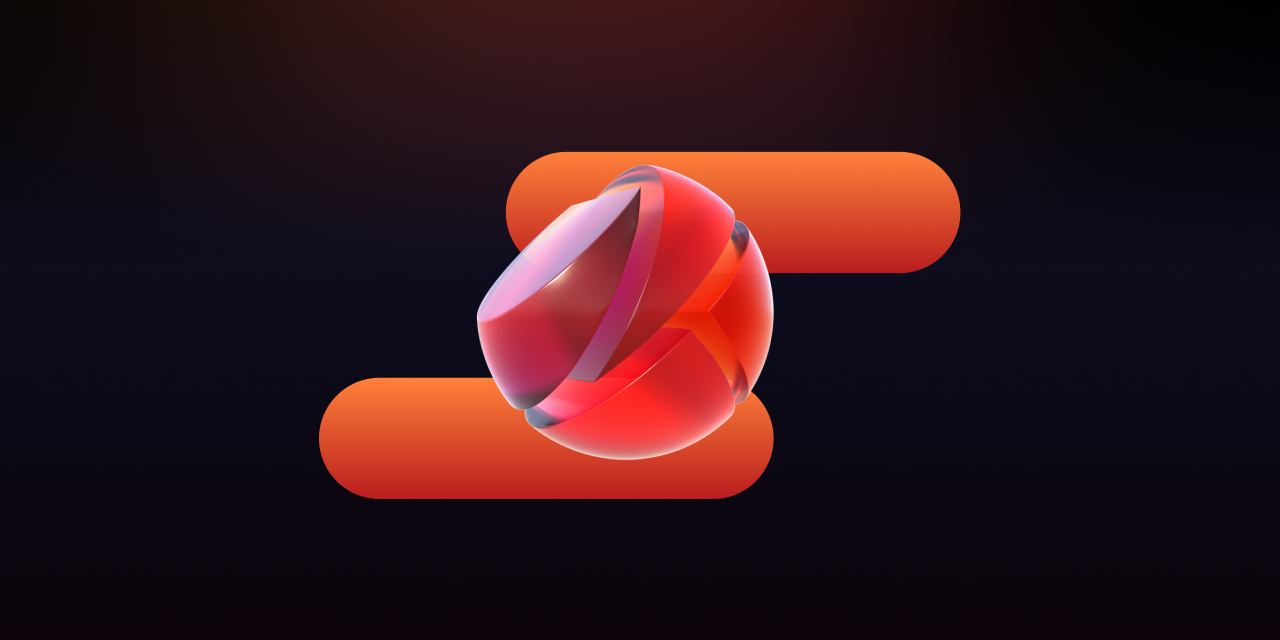](https://github.com/junhoyeo)
Klaytn Multicall
Built for inevitable-dao/bento
Inspired by makerdao/multicall and dopex-io/web3-multicall### 📦 Installation
```bash
# Yarn
yarn add klaytn-multicall# NPM
npm install klaytn-multicall
```### 🚀 Usage
```ts
import { Multicall } from 'klaytn-multicall';const provider = new Caver(...);
const multicall = new Multicall({ provider });const staking = new caver.klay.Contract(...);
const calls = [
staking.methods.balanceOf(
'0x7777777141f111cf9f0308a63dbd9d0cad3010c4',
),
staking.methods.rewardsOf(
'0x7777777141f111cf9f0308a63dbd9d0cad3010c4',
),
];await multicall.aggregate(calls)
.then((console.log));
```From version `1.1.1`, you can also use `web3` (to unify the interface or for other chains) as well.
```ts
const ethereumProvider = new Web3(...); // Ethereum
const klaytnProvider = new Caver(...); // Klaytnconst multicall = new Multicall({
provider: Config.CHAIN === 'klaytn'
? klaytnProvider
: ethereumProvider
});
```### Helpers live inside
- `getEthBalance`: Gets the ~~ETH~~ **KLAY** balance of an address
- `getBlockHash`: Gets the block hash
- `getLastBlockHash`: Gets the last blocks hash
- `getCurrentBlockTimestamp`: Gets the current block timestamp
- `getCurrentBlockDifficulty`: Gets the current block difficulty
- `getCurrentBlockGasLimit`: Gets the current block gas limit
- `getCurrentBlockCoinbase`: Gets the current block coinbase```ts
const multicall = new Multicall({ provider });const calls = [
staking.methods.balanceOf('0x7777777141f111cf9f0308a63dbd9d0cad3010c4'),
staking.methods.rewardsOf('0x7777777141f111cf9f0308a63dbd9d0cad3010c4'),// Queries KLAY balance of address
multicall.contract.methods.getEthBalance(
'0x7777777141f111cf9f0308a63dbd9d0cad3010c4',
),
multicall.contract.methods.getBlockHash(103742609),
multicall.contract.methods.getLastBlockHash(),
];await multicall.aggregate(calls).then(console.log);
```### Customization
You can inject contract address of your custom implementation, too:
```ts
new Multicall({
provider,
multicallV2Address: '0xd11dfc2ab34abd3e1abfba80b99aefbd6255c4b8',
});
````multicallV2Address` defaults to [`0xd11dfc2ab34abd3e1abfba80b99aefbd6255c4b8`](https://scope.klaytn.com/account/0xd11dfc2ab34abd3e1abfba80b99aefbd6255c4b8?tabId=contractCode)([**Multicall2**](https://github.com/makerdao/multicall/blob/master/src/Multicall2.sol) deployed in Cypress).