https://github.com/intere/uitestkit
Programmatic UI Tests (not using the Apple UI Test Framework). XCTest-based tests.
https://github.com/intere/uitestkit
automated-testing cocoapods ios swift testing ui-testing
Last synced: 5 days ago
JSON representation
Programmatic UI Tests (not using the Apple UI Test Framework). XCTest-based tests.
- Host: GitHub
- URL: https://github.com/intere/uitestkit
- Owner: intere
- License: mit
- Created: 2017-11-05T21:55:27.000Z (over 7 years ago)
- Default Branch: develop
- Last Pushed: 2022-05-31T13:58:42.000Z (about 3 years ago)
- Last Synced: 2025-06-16T01:41:58.672Z (about 1 month ago)
- Topics: automated-testing, cocoapods, ios, swift, testing, ui-testing
- Language: Swift
- Size: 1.16 MB
- Stars: 12
- Watchers: 1
- Forks: 1
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# UITestKit
### An iOS Library for UI Testing
## Features
- UI Testing from a vanilla `XCTest` target
- Enable / Disable UI Animations
- Take screenshots on test assertion failures
- `waitForCondition` function to free up the UI thread and wait for your block condition to become true (or timeout and move on)
- `pauseForUIDebug()` function to allow you to pause a (configurable) amount of time between steps in your tests
- function becomes a no-op when `shouldPauseUI` is false (the default)
- variables to get you the top view controller
- Example App to demonstrate capabilities[](https://travis-ci.org/intere/UITestKit)
[](https://intere.github.io/UITestKit/index.html)
[](http://cocoadocs.org/docsets/UITestKit)
[](https://cocoapods.org/pods/UITestKit)
[](https://cocoapods.org/pods/UITestKit) [](https://cocoapods.org/pods/UITestKit)## Why not use XCUITests?
- UITestKit lets you interact with the application code directly from test code
- Easier mocking of model objects
- Easier invocation of ViewControllers
- Lower level access to your application
- UITestKit provides convenience variables for taking screenshots from failures
- UITestKit can be far less rigid than XCUITests## Example
To run the example project, clone the repo, and run `pod install` from the Example directory first.
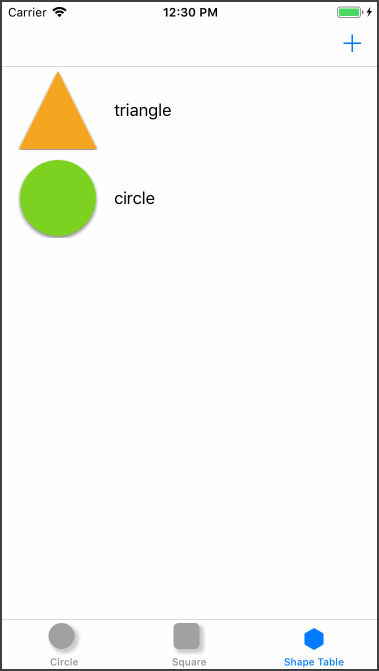## Requirements
- iOS 9.0 or higher## Installation
UITestKit is available through [CocoaPods](http://cocoapods.org). To install
it, simply add the following line to your Podfile:```ruby
pod 'UITestKit'
```### Code Examples
```swift
/// Pre-test initialization
override func setUp() {
super.setUp()
// Don't show view transitions - this will help prevent timing related failures
disableAnimations()
// pause when pauseForUIDebug() is called
shouldPauseUI = true
// how long to pause for when pauseForUIDebug() is called
pauseTimer = 0.3// Now do other setup tasks
}
``````swift
/// Tests logging in to the application
func testLoginSuccess() {// Verify that we're at the Main VC or fail and take a screenshot
XCTAssertTrue(waitForCondition({ self.mainVC != nil }, timeout: 3), topVCScreenshot)
pauseForUIDebug()
mainVC?.loadEmailLoginScreen()// Verify that we're at the Sign in VC or fail and take a screenshot
XCTAssertTrue(waitForCondition({ self.signInVC != nil}, timeout: 1), topVCScreenshot)
pauseForUIDebug()// Simulate typing email
if let emailText = signInVC?.emailText {
emailText.text = "[email protected]"
pauseForUIDebug()
}// simulate typing password
if let passwordText = signInVC?.passwordText {
passwordText.text = "UserPassword"
pauseForUIDebug()
}// log in
signInVC?.login()// Verify that we've signed in successfully and are at the Welcome VC or fail and take a screenshot
XCTAssertTrue(waitForCondition({ self.welcomeVC != nil}, timeout: 5), topVCScreenshot)
}
```### Best Practices
- It is recommended to create a `BaseUITest` class that specializes `UITestKitBase` and provides convenience variables for your application's view controllers:```swift
/// Gets you the `SquareTabVC` if it's the topVC
var squareTabVC: SquareTabVC? {
return topVC as? SquareTabVC
}/// Gets the `ShapesTableViewController`
var shapeTableVC: ShapesTableViewController? {
return topVC as? ShapesTableViewController
}
```- It is recommend that you use the screenshot capability when checking for specific UI's to be visible or in a specific UI state:
```swift
XCTAssertTrue(waitForCondition({ self.shapeTableVC != nil }, timeout: 1), topVCScreenshot)
````topVCScreenshot` will produce failures like this:
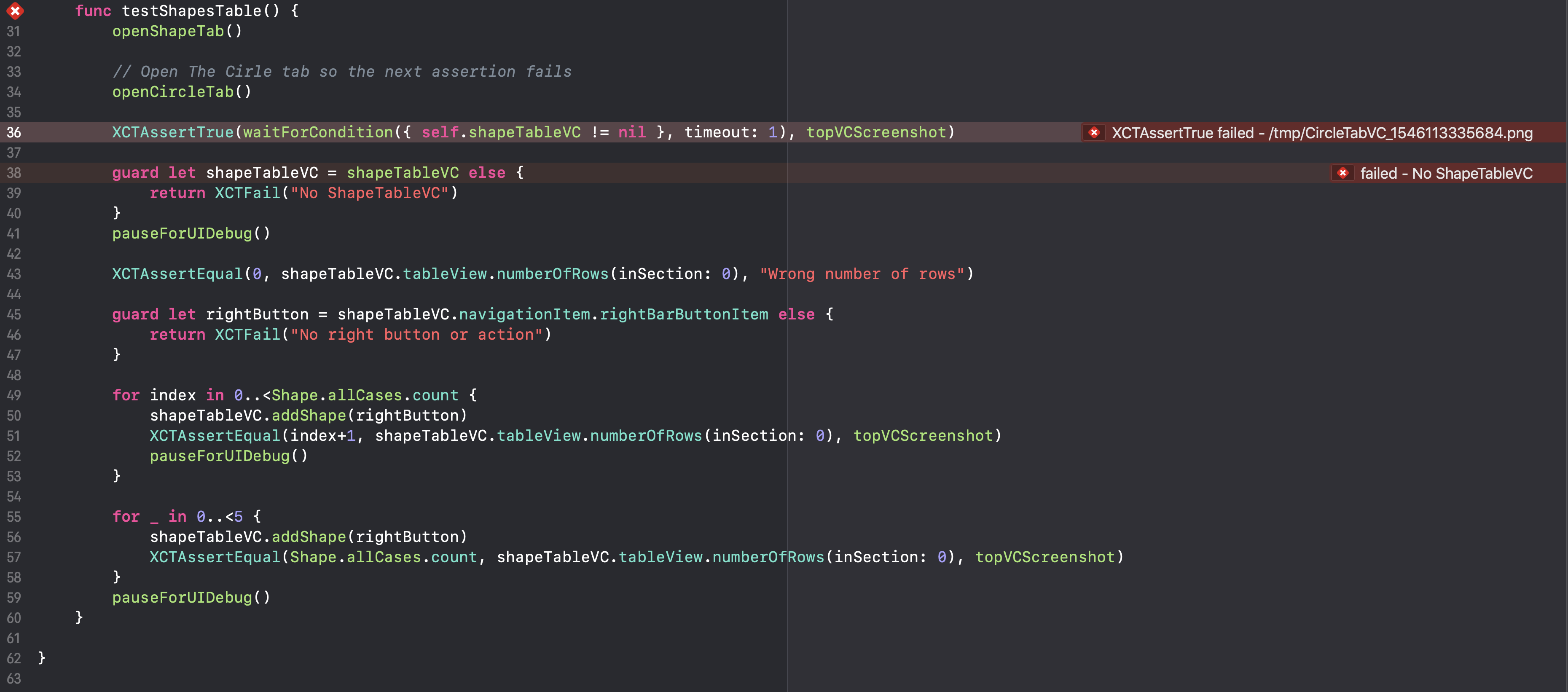
`The Screenshot that is produced will look something like the following:`
## Author
[Eric Internicola](https://github.com/intere) | [Eric's Github Site](https://intere.github.io/)
## License
UITestKit is available under the MIT license. See the [LICENSE file](https://raw.githubusercontent.com/intere/UITestKit/master/LICENSE) for more info.