Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/jafb321/react-game-life
React hook to easily create a Game of Life
https://github.com/jafb321/react-game-life
canvas game-of-life react
Last synced: 3 months ago
JSON representation
React hook to easily create a Game of Life
- Host: GitHub
- URL: https://github.com/jafb321/react-game-life
- Owner: JAFB321
- License: mit
- Created: 2022-07-12T01:39:05.000Z (over 2 years ago)
- Default Branch: main
- Last Pushed: 2022-11-27T03:48:35.000Z (about 2 years ago)
- Last Synced: 2024-10-11T09:39:24.044Z (3 months ago)
- Topics: canvas, game-of-life, react
- Language: TypeScript
- Homepage: https://www.npmjs.com/package/react-game-life
- Size: 15.6 KB
- Stars: 6
- Watchers: 1
- Forks: 1
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
Simple react hook to create amazing game of life automats.
### Install 🐱💻
```bash
npm i react-game-life
```### Basic usage 💡
```javascript
import React from 'react'
import {useGameLife} from 'react-game-life'export default function App() {
const [game, canvasRef] = useGameLife();
return (
)
}
```
##### Result:
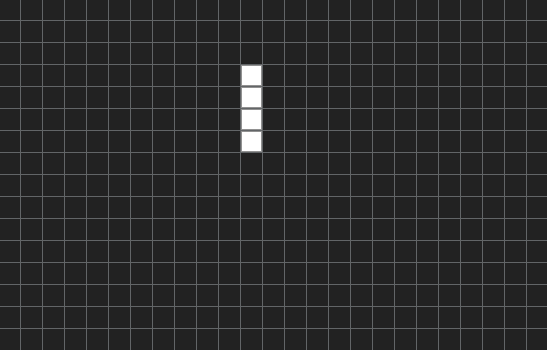##### How to use
- Drag to explore the board
- Double click to spawn/kill cells
- Mouse wheel to zoom in/out
- Enter to start/pause evolution
- +/- keys to speed up/down##### You can also pass default config
```javascript
import React from 'react'
import {useGameLife} from 'react-game-life'export default function App() {
const [game, canvasRef] = useGameLife({
game: {delay: 100},
graphics: {
colors: {background: "#FFF", cell: "0E0E0E"},
board: {height: 800, width: 1200}
}
});return (
)
}
```
##### Result:
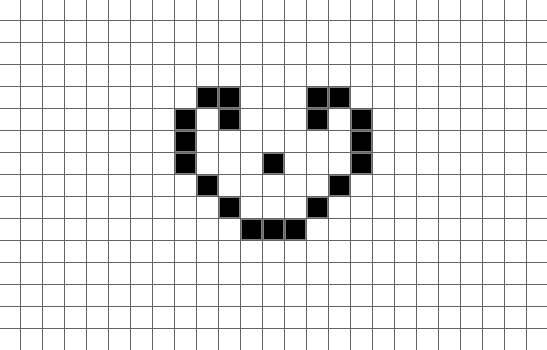### To manually interact/customize the game just add 🤖:
```javascript
useEffect(() => {
game.bornCell({x: 10, t: 10}) // Spawn cell
game.killCell({x: 10, y: 10}) // Kill cell
game.startEvolution() // Start
game.stopEvolution() // Stop
game.speedUp(1.5) // Speed up 1.5x
game.speedDown(0.8) // Speed down 0.8x
game.graphics.setConfig({ // Change graphics config
colors: {background: '#F0F0F0', cell: '#000000'}
})
// and more
}, [])
```
You can use thegame
object where you want to call it's methods (The useEffect is optional, you can use the game inside a simple function)##### Game API 🚀
[Game life repository](https://github.com/JAFB321/game-life)