Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/jasonsturges/sqlite-dotnet-core
.NET 7.0 Console Application using SQLite with Entity Framework and Dependency Injection
https://github.com/jasonsturges/sqlite-dotnet-core
console-application database dependency-injection dotnet dotnet-core entity-framework sqlite
Last synced: about 2 months ago
JSON representation
.NET 7.0 Console Application using SQLite with Entity Framework and Dependency Injection
- Host: GitHub
- URL: https://github.com/jasonsturges/sqlite-dotnet-core
- Owner: jasonsturges
- Created: 2018-05-23T02:34:32.000Z (over 6 years ago)
- Default Branch: main
- Last Pushed: 2023-11-18T21:22:46.000Z (about 1 year ago)
- Last Synced: 2023-11-18T22:28:48.941Z (about 1 year ago)
- Topics: console-application, database, dependency-injection, dotnet, dotnet-core, entity-framework, sqlite
- Language: C#
- Homepage:
- Size: 43.9 KB
- Stars: 21
- Watchers: 2
- Forks: 9
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# SQLite .NET 8 Console App
.NET 8.0 Console Application using SQLite with Entity Framework and Dependency InjectionThis example shows how to incorporate ASP.NET concepts such as dependency injection within a console application using [VS Code](https://code.visualstudio.com/) on macOS or linux targets.
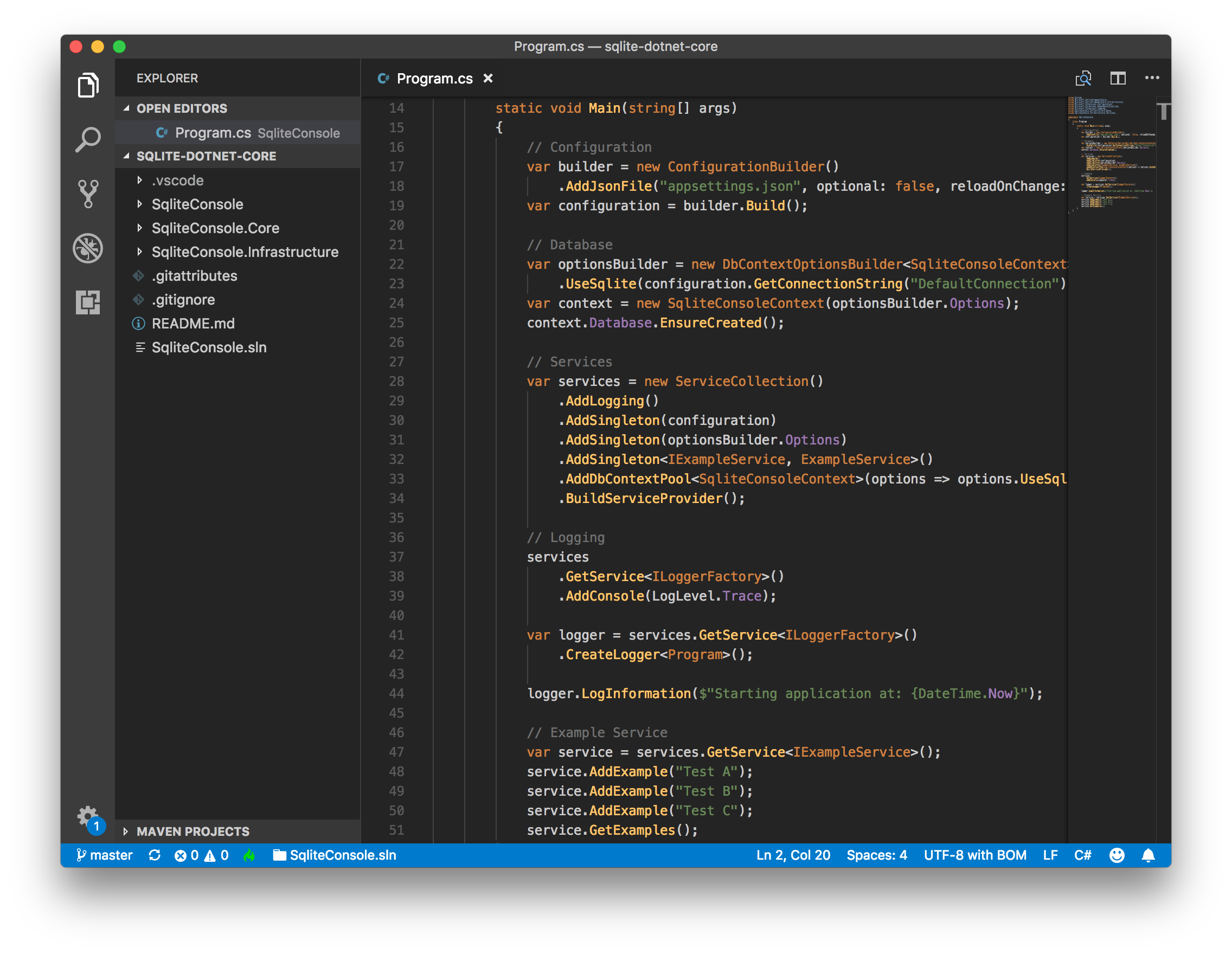
## Project Structure
This solution is divided into three projects:
- **SqliteConsole**: The main console application
- **SqliteConsole.Core**: Entity models
- **SqliteConsole.Infrasture**: Entity framework database context and service classes## Concepts
The following concepts are demonstrated within this example console application project:
- SQLite Entity Framework
- Dependency Injection### SQLite Entity Framework
Using dependency injection, the database context can be passed to a constructor of a class:
```cs
public class ExampleService : IExampleService
{
private readonly SqliteConsoleContext context;public ExampleService(SqliteConsoleContext sqliteConsoleContext)
{
context = sqliteConsoleContext;
}
```This way, the context may be used as follows:
```cs
public void GetExamples()
{
var examples = context.Examples
.OrderBy(e => e.Name)
.ToList();
```Otherwise, there's a factory method to instantiate new contexts:
```cs
using (var context = SqliteConsoleContextFactory.Create(config.GetConnectionString("DefaultConnection")))
{
var examples = context.Examples
.OrderBy(e => e.Name)
.ToList();
}
```
### Dependency InjectionService classes are added to the main console application's Program.cs:
```cs
// Services
var services = new ServiceCollection()
.AddSingleton()
.BuildServiceProvider();
```Then, obtain the instance of the service as:
```cs
var service = services.GetService();
```