https://github.com/javapuppteernodejs/puppeteer-recaptcha-solver
https://github.com/javapuppteernodejs/puppeteer-recaptcha-solver
javascript puppeteer recaptcha recaptcha-bypass-script recaptcha-solver recaptcha-v2 recaptcha-v2-captcha-solver recaptcha-v2-solver recaptcha-v3
Last synced: 3 months ago
JSON representation
- Host: GitHub
- URL: https://github.com/javapuppteernodejs/puppeteer-recaptcha-solver
- Owner: javapuppteernodejs
- Created: 2024-08-16T07:07:42.000Z (11 months ago)
- Default Branch: main
- Last Pushed: 2024-08-16T07:12:26.000Z (11 months ago)
- Last Synced: 2025-02-15T03:14:22.175Z (5 months ago)
- Topics: javascript, puppeteer, recaptcha, recaptcha-bypass-script, recaptcha-solver, recaptcha-v2, recaptcha-v2-captcha-solver, recaptcha-v2-solver, recaptcha-v3
- Homepage:
- Size: 3.91 KB
- Stars: 1
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# Puppeteer Recaptcha Solver: A Comprehensive Guide
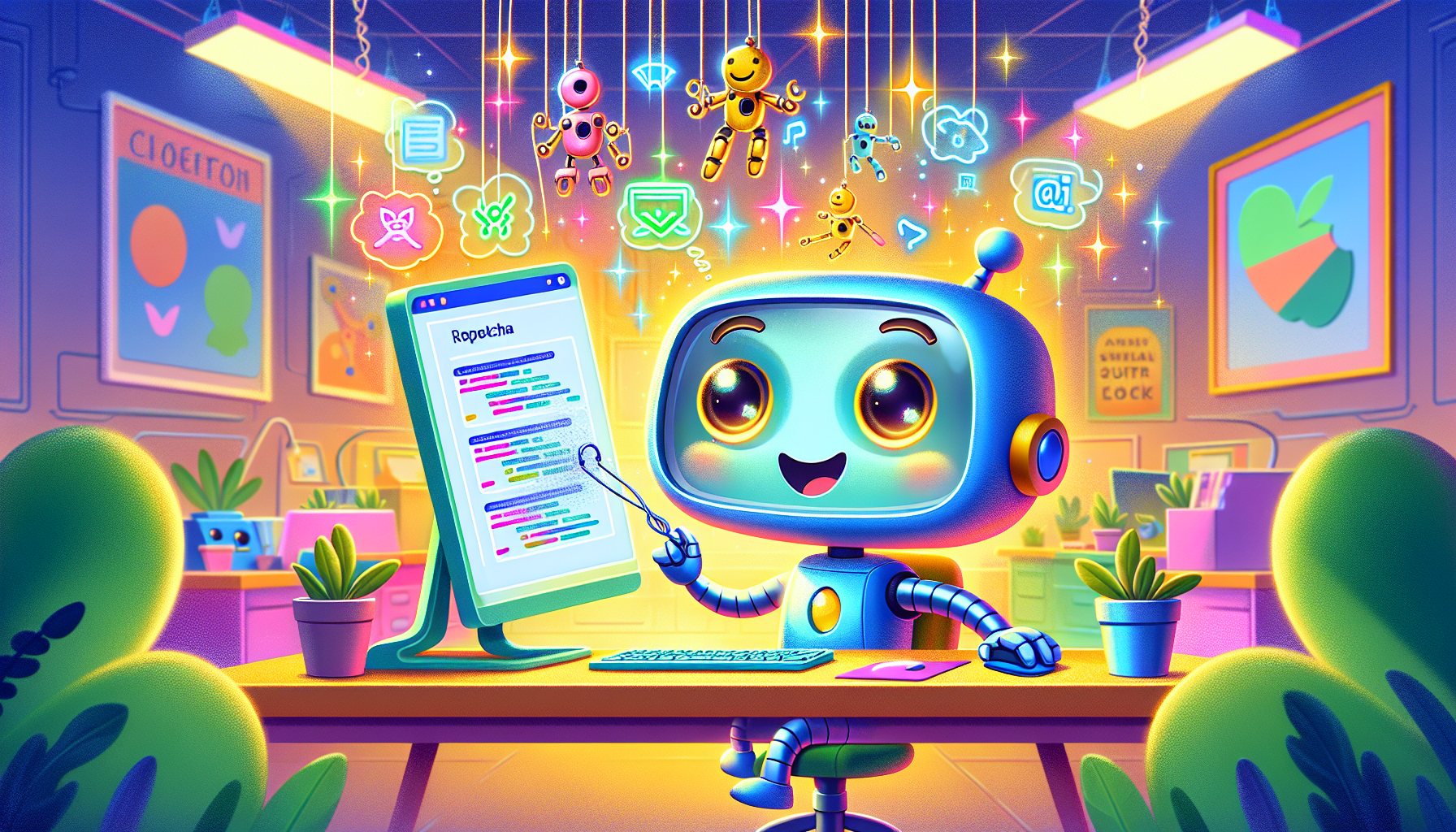
CAPTCHAs, especially reCAPTCHAs, are common security measures used by websites to distinguish between human users and automated bots. While CAPTCHAs serve an essential purpose, they can be a hindrance for developers involved in web scraping or automated testing. Thankfully, tools like Puppeteer, in conjunction with CAPTCHA solving services, make it possible to bypass these challenges efficiently. So today, we'll explore how to use Puppeteer as a reCAPTCHA solver and the various methods available to integrate it into your workflow.
## What is Puppeteer?
Puppeteer is a Node.js library that provides a high-level API to control Chrome or Chromium browsers. It's primarily used for tasks such as automated testing, scraping, and generating PDFs from web pages. Puppeteer is well-suited for navigating websites, clicking on buttons, and even handling complex JavaScript applications.
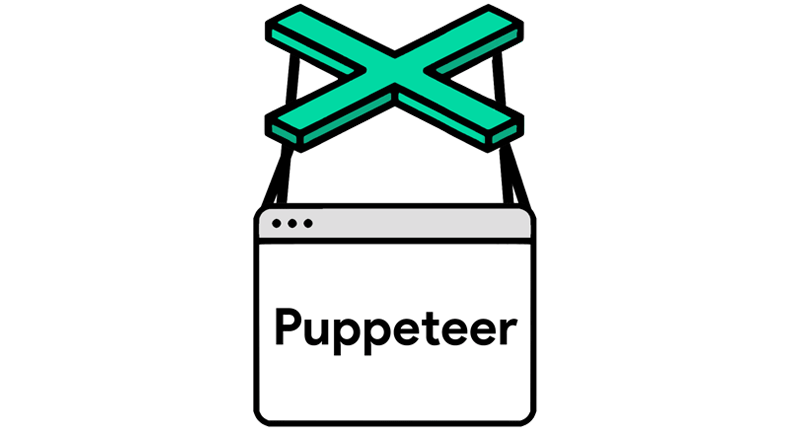## The Challenge with reCAPTCHA
reCAPTCHA is a more sophisticated CAPTCHA designed to prevent bots from accessing web services. It often requires users to identify objects in images or simply click on a checkbox. For a bot, these tasks are challenging without the appropriate tools.
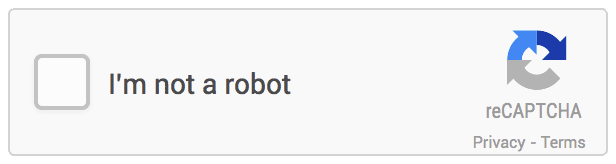When using Puppeteer for web scraping or automation, encountering a reCAPTCHA can halt the entire process. To continue, you need a way to solve the reCAPTCHA programmatically.
## Solving reCAPTCHA with Puppeteer
To solve reCAPTCHAs using Puppeteer, you can follow several approaches. Here’s an overview of the most effective methods:
1. **Manual Bypass**:
This method involves manually solving the CAPTCHA using Puppeteer’s interactive mode. This is feasible for testing, but not practical for large-scale automation.
2. **Third-Party CAPTCHA Solvers**:
The most efficient way to handle reCAPTCHAs programmatically is to use third-party CAPTCHA solving services like [CapSolver](https://www.capsolver.com/?utm_source=official&utm_medium=blog&utm_campaign=recaptchapuppteer). These services provide APIs that can solve reCAPTCHAs for you and return the response token that you can submit to the website.
3. **Custom Solutions**:
For advanced users, creating a custom reCAPTCHA solving system using machine learning models is possible. However, this requires substantial resources and expertise.
## Using a Third-Party CAPTCHA Solver with Puppeteer
Let’s focus on integrating a third-party CAPTCHA solver with Puppeteer. Below is a step-by-step guide to [solve reCAPTCHA using CapSolver](https://docs.capsolver.com/guide/captcha/ReCaptchaV2.html?utm_source=official&utm_medium=blog&utm_campaign=recaptchapuppteer).
1. **Install Required Dependencies**:
- First, ensure you have Puppeteer and the `axios` library installed, which will be used for making HTTP requests to the CAPTCHA solving service.
```bash
npm install puppeteer axios
```2. **Set Up Puppeteer**:
- Launch Puppeteer and navigate to the target website where the reCAPTCHA needs to be solved.
```javascript
const puppeteer = require('puppeteer');async function solveRecaptcha(url) {
const browser = await puppeteer.launch({ headless: false });
const page = await browser.newPage();
await page.goto(url);// Additional Puppeteer logic here
}solveRecaptcha('https://example.com');
```4. **Request reCAPTCHA Solving**:
- Use `axios` to send a request to CapSolver's API to solve the reCAPTCHA.
```javascript
const axios = require('axios');async function getCaptchaSolution(siteKey, pageUrl, apiKey) {
const response = await axios.post('https://api.capsolver.com/createTask', {
clientKey: apiKey,
task: {
type: 'ReCaptchaV2Task',
websiteURL: pageUrl,
websiteKey: siteKey,
},
});const taskId = response.data.taskId;
let solution = '';// Polling for the solution
while (!solution) {
const result = await axios.post('https://api.capsolver.com/getTaskResult', {
clientKey: apiKey,
taskId: taskId,
});if (result.data.status === 'ready') {
solution = result.data.solution.gRecaptchaResponse;
} else {
await new Promise((resolve) => setTimeout(resolve, 5000)); // Wait for 5 seconds before retrying
}
}return solution;
}
```5. **Inject the CAPTCHA Solution**:
- Once the solution is obtained, inject it into the page and submit the form.
```javascript
const siteKey = 'SITE_KEY';
const pageUrl = 'https://example.com';
const apiKey = 'YOUR_CAPSOLVER_API_KEY';const captchaSolution = await getCaptchaSolution(siteKey, pageUrl, apiKey);
await page.evaluate((captchaSolution) => {
document.querySelector('#g-recaptcha-response').innerHTML = captchaSolution;
document.querySelector('form').submit();
}, captchaSolution);
```7. **Complete the Process**:
- Close the browser or continue with the next steps in your automation.```javascript
await browser.close();
```## Advanced Techniques
For more advanced use cases, consider integrating Puppeteer with tools like `undetected-chromedriver` to avoid detection or using the Playwright library as an alternative. Playwright offers similar functionality to Puppeteer but provides more advanced browser automation features, including support for multiple browsers and better handling of web scraping challenges like dynamic content and CAPTCHAs.
## Conclusion
Solving [reCAPTCHA](https://docs.capsolver.com/guide/captcha/ReCaptchaV2.html?utm_source=official&utm_medium=blog&utm_campaign=recaptchapuppteer) with Puppeteer can significantly streamline your automation and web scraping tasks. By leveraging third-party CAPTCHA solvers like CapSolver, you can bypass these security measures efficiently. Whether you're scraping data or automating interactions, this guide provides the foundation you need to integrate reCAPTCHA solving into your Puppeteer projects.
Remember, it's essential to use these tools responsibly and ensure that your activities comply with the legal and ethical standards of the websites you're interacting with.