Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/jcouyang/owlet
Typed Spreadsheet UI library for ScalaJS
https://github.com/jcouyang/owlet
applicative birds functional-programming reactive scala scalajs spreadsheet ui
Last synced: about 2 months ago
JSON representation
Typed Spreadsheet UI library for ScalaJS
- Host: GitHub
- URL: https://github.com/jcouyang/owlet
- Owner: jcouyang
- Created: 2018-05-26T08:19:36.000Z (over 6 years ago)
- Default Branch: master
- Last Pushed: 2022-12-07T19:35:56.000Z (about 2 years ago)
- Last Synced: 2024-05-02T00:39:06.430Z (8 months ago)
- Topics: applicative, birds, functional-programming, reactive, scala, scalajs, spreadsheet, ui
- Language: Scala
- Homepage: https://oyanglul.us/owlet/
- Size: 2.98 MB
- Stars: 40
- Watchers: 4
- Forks: 5
- Open Issues: 6
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# Owlet
Owlet is a Typed Spreadsheet UI library for ScalaJS. It is built on top of [Monix](https://monix.io/) and [Typelevel Cats](https://typelevel.org/cats/) to combine predefined input fields to a reactive user interface, just like what you would done in spreadsheet. Owlet is inspired by the PureScript library [Flare](https://github.com/sharkdp/purescript-flare).
[
](https://zh.wikisource.org/wiki/%E6%AC%BD%E5%AE%9A%E5%8F%A4%E4%BB%8A%E5%9C%96%E6%9B%B8%E9%9B%86%E6%88%90/%E5%8D%9A%E7%89%A9%E5%BD%99%E7%B7%A8/%E7%A6%BD%E8%9F%B2%E5%85%B8)
**Do one thing and do it well** micro [birds](https://github.com/search?q=org%3Ajcouyang+topic%3Abirds&type=Repositories) library series
## Get Started
### 1. add dependency in your `build.sbt`
#### Stable
[](https://index.scala-lang.org/jcouyang/owlet/owlet)```
libraryDependencies += "us.oyanglul" %%% "owlet" % ""
```#### RC
[](https://jitpack.io/#jcouyang/owlet)```
resolvers += "jitpack" at "https://jitpack.io"
libraryDependencies += "com.github.jcouyang" % "owlet" % ""
```### 2. Now programming UI is just like using spreadsheet
```scala
import us.oyanglul.owlet._
import DOM._
val a1 = number("a1", 1)
val a2 = number("a2", 2)
val a3 = number("a3", 3)
val sum = fx[List, Double, Double](_.sum, List(a1, a2, a3))
render(a1 &> a2 &> a3 &> sum, "#app")
```or 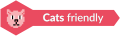
```scala
val a1 = number("a1", 1)
val a2 = number("a2", 2)
val a3 = number("a3", 3)
val sum = a1 |+| a2 |+| a3
renderOutput(sum, "#app")
```
### eh... Ready for 3D spreadsheet programming?
You know spreadsheet is 2D, when we have monad, it became 3D!!!Monad Warning!!!
```scala
val numOfItem = int("noi", 3)
val items = numOfItem
.flatMap(
no => (0 to no).toList.parTraverse(i => string("inner", i.toString))
)
```
- imagine that `numOfItem` lives in dimension (x=1, y=1, z=0)
- then `items` live in dimension (x=1,y=1,z=1)you can render either `numOfItem` or `items` seperatly, for they live in diffenrent z axis (which means render `items` you won't able to see `numOfItem` even it's flatMap from there
but you can some how connect the dots with magic `&>`
```scala
renderOutput(numOfItem &> items, "#output")
```Anyway, just keep in mind that monad ops `map` `ap` `flatMap`... will lift your z axis
`parMap` `parAp` `parXXX` instead, will keep them in the same z axis## More...
- [Tutorial](https://oyanglul.us/owlet/Tutorial.html)
- Tweaking Owlet with [Lens](https://oyanglul.us/owlet/Lens.html)
- [Todo MVC](https://oyanglul.us/owlet/todomvc.html) Completed
- [API Doc](https://oyanglul.us/owlet/api)