https://github.com/jgrancher/react-native-sketch
🎨 A React Native <Sketch /> component for touch-based drawing.
https://github.com/jgrancher/react-native-sketch
drawing ios react-native signature sketch touch
Last synced: 6 months ago
JSON representation
🎨 A React Native <Sketch /> component for touch-based drawing.
- Host: GitHub
- URL: https://github.com/jgrancher/react-native-sketch
- Owner: jgrancher
- License: mit
- Archived: true
- Created: 2016-05-10T12:41:43.000Z (about 9 years ago)
- Default Branch: master
- Last Pushed: 2021-08-12T07:17:47.000Z (almost 4 years ago)
- Last Synced: 2024-04-25T00:50:59.747Z (about 1 year ago)
- Topics: drawing, ios, react-native, signature, sketch, touch
- Language: Objective-C
- Homepage: http://npm.im/react-native-sketch
- Size: 396 KB
- Stars: 644
- Watchers: 8
- Forks: 68
- Open Issues: 12
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- License: LICENSE
Awesome Lists containing this project
- awesome-react-native - react-native-sketch ★467 - A react-native <Sketch /> component to draw with touch events. (Components / UI)
- awesome-react-native-native-modules - react-native-sketch ★363 - based drawing. (<a name="UI:-Native-Modules">UI: Native Modules</a>)
- awesome-reactnative-ui - react-native-sketch - based drawing.|<ul><li>Last updated : This week</li><li>Stars : 518</li><li>Open issues : 12</li></ul>|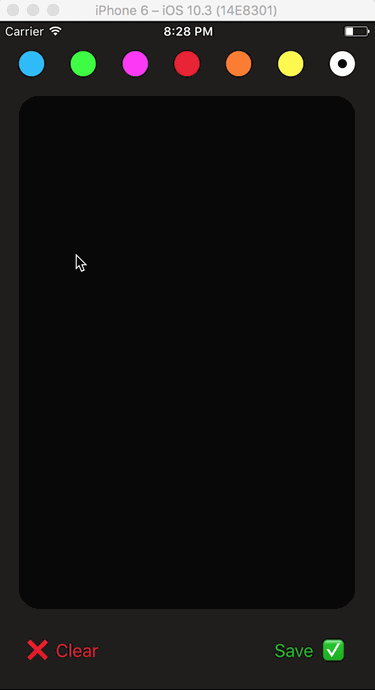| (Others)
- awesome-react-native - react-native-sketch ★467 - A react-native <Sketch /> component to draw with touch events. (Components / UI)
- awesome-reactnative-ui - react-native-sketch - based drawing.|<ul><li>Last updated : This week</li><li>Stars : 518</li><li>Open issues : 12</li></ul>|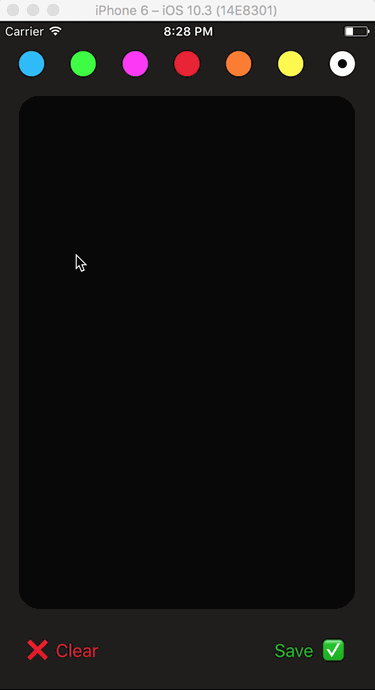| (Others)
- awesome-react-native - react-native-sketch ★467 - A react-native <Sketch /> component to draw with touch events. (Components / UI)
- awesome-react-native-ui - react-native-sketch ★109 - A react-native <Sketch /> component to draw with touch events. (Components / UI)
- awesome-react-native - react-native-sketch ★467 - A react-native <Sketch /> component to draw with touch events. (Components / UI)
README
# 🎨 React Native Sketch [Unmaintained]
[](https://www.npmjs.com/package/react-native-sketch)
[](https://www.npmjs.com/package/react-native-sketch)
[](http://opensource.org/licenses/MIT)**⚠️ NOTE [12/08/2021]: Archiving this repository because I haven't had the resources to maintain it for a while. Apologies.**
A React Native component for touch-based drawing.
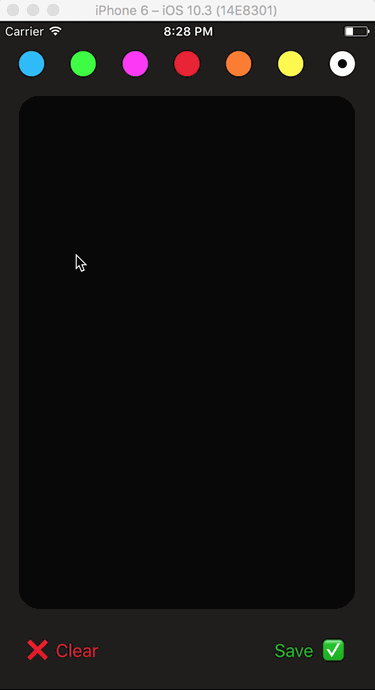
## Features
- 👆 Draw with your finger, and export an image from it.
- 🖍 Change the stroke color and thickness on the fly.
- 👻 Generate a transparent image (or not).## Setup
Install the module from npm:
```bash
npm i -S react-native-sketch
```Link the module to your project:
```bash
react-native link react-native-sketch
```## Usage
```javascript
import React from 'react';
import { Alert, Button, View } from 'react-native';
import Sketch from 'react-native-sketch';export default class MyPaint extends React.Component {
save = () => {
this.sketch.save().then(({ path }) => {
Alert.alert('Image saved!', path);
});
};render() {
return (
{
this.sketch = sketch;
}}
strokeColor="#ff69b4"
strokeThickness={3}
/>
);
}
}
```## API
Here are the `props` of the the component:
| Name | Type | Default value | Comment |
| ---- | ---- | ------------- | ---- |
| `fillColor` | `String` | `null` | The color of the sketch background. Default to null to keep it transparent! *Note: This is different from the `style.backgroundColor` property, as the former will be seen in your exported drawing image, whereas the latter is only used to style the view.* |
| `imageType` | `String` | `png` | The type of image to export. Can be `png` or `jpg`. Default to `png` to get transparency out of the box. |
| `onChange` | `Function` | `() => {}` | Callback function triggered after every change on the drawing. The function takes one argument: the actual base64 representation of your drawing.|
| `onClear` | `Function` | `() => {}` | Callback function triggered after a `clear` has been triggered. |
| `strokeColor` | `String` | `'#000000'` | The stroke color you want to draw with. |
| `strokeThickness` | `Number` | `1` | The stroke thickness, in pixels. |
| `style` | Style object | `null` | Some `View` styles if you need. |The component also has some instance methods:
| Name | Return type | Comment |
| ---- | ----------- | ------- |
| `clear()` | `Promise` | Clear the drawing. This method is a Promise mostly to be consistent with `save()`, but you could simply type: `this.sketch.clear();` |
| `save()` | `Promise` | Save the drawing to an image, using the defined props as settings (`imageType`, `fillColor`, etc...). The Promise resolves with an object containing the `path` property of that image. Ex: `this.sketch.save().then(image => console.log(image.path));` |## Examples
The project contains a folder `examples` that contains few demos of how to use `react-native-sketch`. Just copy and paste the code to your React Native application.
- [`Basic`](https://github.com/jgrancher/react-native-sketch/tree/master/examples/basic): uses the bare minimum to make it work.
- [`Digital Touch`](https://github.com/jgrancher/react-native-sketch/tree/master/examples/digital-touch): tries to reproduce the look and feel of iOS Message Digital Touch.Feel free to play with them!
## Known issues
- Rotating the screen gets to a weird behavior of the sketch view: [#23](https://github.com/jgrancher/react-native-sketch/issues/23)
## Notes
- If you're using Expo, you will have to [`detach` to ExpoKit](https://docs.expo.io/versions/latest/guides/detach.html), as this module uses native iOS code.
- The module is available *only on iOS for now*, as I don't know Android development... But if you think you can help on that matter, please feel free to create a Pull Request!
- The module uses this [smooth freehand drawing technique](http://code.tutsplus.com/tutorials/smooth-freehand-drawing-on-ios--mobile-13164) under the hood.## Contributing
Feel free to contribute by sending a pull request or [creating an issue](https://github.com/jgrancher/react-native-sketch/issues/new).
## License
[MIT](https://github.com/jgrancher/react-native-sketch/tree/master/LICENSE)