https://github.com/jsmolka/maze
Create and solve mazes in Python.
https://github.com/jsmolka/maze
algorithm maze python
Last synced: 3 months ago
JSON representation
Create and solve mazes in Python.
- Host: GitHub
- URL: https://github.com/jsmolka/maze
- Owner: jsmolka
- Created: 2017-07-24T19:26:59.000Z (almost 8 years ago)
- Default Branch: master
- Last Pushed: 2018-12-11T10:27:30.000Z (over 6 years ago)
- Last Synced: 2025-03-20T17:05:45.236Z (3 months ago)
- Topics: algorithm, maze, python
- Language: Python
- Homepage:
- Size: 218 KB
- Stars: 38
- Watchers: 2
- Forks: 8
- Open Issues: 0
-
Metadata Files:
- Readme: readme.md
Awesome Lists containing this project
README
# maze
Create and solve mazes in Python.## How to use
```python
from maze import *m = Maze()
m.create(25, 25, Maze.Create.BACKTRACKING)
m.save_maze()
m.solve((0, 0), (24, 24), Maze.Solve.DEPTH)
m.save_solution()
```
The code above creates the following pictures: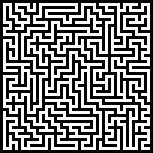 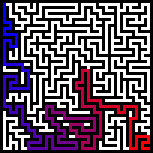
## Algorithms
### Creating
- Recursive backtracking algorithm
- Hunt and kill algorithm
- Eller's algorithm
- Sidewinder algorithm
- Prim's algorithm
- Kruskal's algorithm### Solving
- Depth-first search
- Breadth-first search### C
The recursive backtracking algorithm and depth-first search are also implemented in C. They are around 100x faster than their Python counterparts.## How to install
Simply go into the ```setup.py``` directory and run ```pip install .``` to install the package.## Requirements
- NumPy
- Pillow
- [pyprocessing](https://github.com/jsmolka/pyprocessing) if you want to run the visual examples