https://github.com/jwilson8767/template-literals-cli
Provides a simple way to build ES6 Template Literals into static html files with an optional yaml or json config/data file.
https://github.com/jwilson8767/template-literals-cli
es6 npm npm-scripts template-engine-html template-literals
Last synced: 3 months ago
JSON representation
Provides a simple way to build ES6 Template Literals into static html files with an optional yaml or json config/data file.
- Host: GitHub
- URL: https://github.com/jwilson8767/template-literals-cli
- Owner: jwilson8767
- License: mit
- Created: 2018-09-20T13:12:43.000Z (over 6 years ago)
- Default Branch: master
- Last Pushed: 2022-03-16T18:58:01.000Z (about 3 years ago)
- Last Synced: 2025-01-31T08:47:05.679Z (4 months ago)
- Topics: es6, npm, npm-scripts, template-engine-html, template-literals
- Language: JavaScript
- Size: 15.6 KB
- Stars: 1
- Watchers: 2
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: readme.md
- License: LICENSE
Awesome Lists containing this project
README
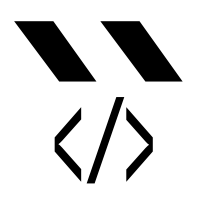
# template-literals-cli
> Templates so literal, a barbarian can do it
Provides a simple way to build [ES6 Template Literals](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Template_literals) into static html files with an optional yaml or json config/data file.
## Getting started
1. Install via `npm install template-literals-cli -g`2. Create a config/data file using either YAML or json. For example `mydata.yml`:
```yaml
fire_hot: true
exclamations:
- 'UYGH!'
- 'GRRAH!'
- 'OOAH!'
colors:
- 'red'
- 'orange'
- 'yellow'
```3. Create a template file which exports a default function. For example `touchfire.js`:
```js
export default (config)=>`
${ config['fire_hot'] ? config['exclamations'][Math.floor(Math.random() * config['exclamations'].length)] : 'Wha'}
${ config['exclamations'].join(' ') }
${ config['fire_hot'] ? config['exclamations'].map((exclamation, index)=>`
${exclamation}
`).join(''):'' }
`
```3. Build the file into `dist/touchfire.html` using `template-literals --config config.yml --outdir dist touchfire.js`
4. (optional) Start the http server of your choice in `dist/` and visit `http://localhost/touchfire.html`. Optionally you can build again using `template-literals --config config.yml --outdir dist --indexes touchfire.js` and then visit `http://localhost/touchfire/` if you want a prettier url.
5. (optional) Add the npm script below to your project's `package.json` so can just run `npm run build` instead of remembering your exact build command:
```json
{
"scripts": {
"build": "template-literals --config config.yml --outdir dist --indexes src/*.js"
}
}
```
Note that the wildcard is expanded by your terminal and therefore may not work on Windows/wherever glob is not available.## Importing other templates
Using templates from other files is easy, just import the desired template like this:
```js
// File: templates/header.js
export default (config)=>`
`
// end file
// File: index.js
import header from 'templates/header';
export default (config)=>`
${ header(config) }
`
// endfile
```
## Complex logic
If your templates start to get complicated you can always fall back to javascript to handle complex bits - so long as the default export returns a string.
```js
import item_card from 'templates/item_card.js';
export default (config)=> {
let x = 0;
let cards = config['items'].map((item)=>{
return item_card(item, x++);
});
return `
${ cards.join('') }
`
}
```
## Injecting environment variables
Occasionally, it's helpful to inject variables at build-time. As of 0.2.0, any key=value pairs after `--` will be processed as additional config properties and can even override existing values in the config file.
```js
//myPage.js
export default ({env="prod"})=>`
...
...
${/* Use env to switch between minified and unminified javascript files */ '' }
${env === 'prod' ? `
` : `
`}
`;
```
`template-literals --config "config.yml" --outdir ./ ./src/myPage.js -- env=dev`
### A big stick
These overrides have a couple super powers. Take the following config:
```json5
{
"projects": [
{
"title": "My Project",
"figures": {
"sales_6mo": "/images/sales.png",
"sales_3mo": "/images/sales2.png"
}
},
// ...
],
// ...
}
```
Now imagine you need to override the project title. By specifying a key with **dot-notation** you can change properties deep in your config:
`template-literals --config "config.yml" --outdir ./ ./src/myPage.js -- projects.0.title="The Best Project"`
And to take things a step further, you can completely override `projects.0.figures` with a new object by passing **JSON as a value**:
`template-literals --config "config.yml" --outdir ./ ./src/myPage.js -- projects.0.title="The Best Project" projects.0.figures='{"sales_1mo":"/images/sales_1mo.png","sales_3mo":"/images/sales_3mo.png"}'`
Final result:
```json5
{
"projects": [
{
"title": "My Best Project",
"figures": {
"sales_1mo":"/images/sales_1mo.png",
"sales_3mo":"/images/sales_3mo.png",
}
},
// ...
],
// ...
}
```