Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/kareemkibue/k2-react-translate
A simple, easy-to-use translation library based on Context API, with optional localized react-router routing.
https://github.com/kareemkibue/k2-react-translate
localization react react-js react-router react-translate translation
Last synced: 4 months ago
JSON representation
A simple, easy-to-use translation library based on Context API, with optional localized react-router routing.
- Host: GitHub
- URL: https://github.com/kareemkibue/k2-react-translate
- Owner: kareemkibue
- License: mit
- Created: 2019-09-30T14:30:21.000Z (over 5 years ago)
- Default Branch: master
- Last Pushed: 2023-03-26T18:28:58.000Z (almost 2 years ago)
- Last Synced: 2024-09-30T13:23:20.452Z (4 months ago)
- Topics: localization, react, react-js, react-router, react-translate, translation
- Language: TypeScript
- Homepage: https://codesandbox.io/s/confident-microservice-l0een
- Size: 2.48 MB
- Stars: 3
- Watchers: 1
- Forks: 0
- Open Issues: 24
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# k2-react-translate
[](https://www.npmjs.com/package/k2-react-translate)
[](https://www.npmjs.com/package/k2-react-translate)
[](https://www.npmjs.com/package/k2-react-translate)
[](https://github.com/kareemkibue/k2-react-translate/issues?q=is%3Aissue+is%3Aopen+sort%3Aupdated-desc)
[](https://github.com/kareemkibue/k2-react-translate/pulls?q=is%3Apr+is%3Aopen+sort%3Aupdated-desc)
[](https://github.com/kareemkibue/k2-react-translate/blob/master/LICENSE)


A simple, easy-to-use translation library based on React's Context API, with optional localized
`react-router` routing.See demo on [CodeSandbox](https://codesandbox.io/s/confident-microservice-l0een).
[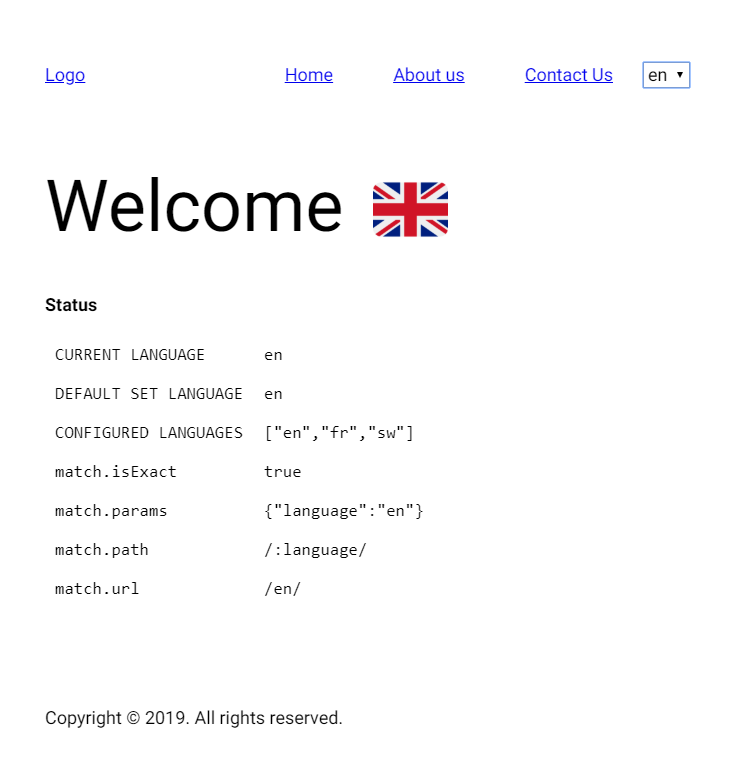](https://codesandbox.io/s/confident-microservice-l0een)
## Table of Contents
- [Problem Statement](#problem-statement)
- [Setup](#setup)
- [Documentation (Translation)](#documentation-translation)
- [Documentation (Localized Routing)](#documentation-localized-routing)
- [Development](#development)
- [Known Issues](#known-issues)
- [Changelog](#changelog)## Problem Statement
TBD
## Setup
This ES5 module is distributed via [npm](https://www.npmjs.com/package/k2-react-translate) and
should be installed as a production dependency.Note: TypeScript syntax is used throughout the docs, but the library should run without issue on a
`babel/react` setup.Using _yarn_ (preferred)
```
yarn add -E k2-react-translate
```or via _npm_
```
npm i -S -E k2-react-translate
```### `peerDependencies`
- [`react@^16.8.0`](https://github.com/facebook/react)
- `react@^16.8.0` would have to be installed to use the `useTranslate` hook.
- [`react-dom@^16.8.0`](https://github.com/facebook/react/tree/master/packages/react-dom)
- [`react-router-dom`](https://github.com/ReactTraining/react-router/tree/master/packages/react-router-dom)
- [`history`](https://github.com/ReactTraining/history)Typescirpt type definitions come bundled in.
### Project Setup
If using TypeScript in VS Code, add the following configuration to your `tsconfig.json` to allow for
importing json files into your modules:```jsonc
// tsconfig.json
{
"compilerOptions": {
// other config options ...
"resolveJsonModule": true
}
}
```Setup your translations:
If using VS Code, the richie5um2's
[Sort JSON objects](https://marketplace.visualstudio.com/items?itemName=richie5um2.vscode-sort-json)
marketplace extension may help to keep your translations file organized.```jsonc
// translations.json
{
"HELLO": {
"en": "Hello",
"fr": "Bonjour"
},
"HELLO_NAME": {
"en": "Hello, {firstName}",
"fr": "Bonjour, {firstName}"
},
"AVAILABLE_IN_COUNTRY": {
"en": "Available in {countryName}",
"fr": "Disponsible en {countryName}"
},
"PRIVACY_POLICY": {
"en": "Privacy Policy",
"fr": "Politique de Confidentialité"
}
}
```Entry point:
```tsx
// index.tsx
import * as React from 'react'; // standard TypeScript syntax
import * as ReactDOM from 'react-dom'; // standard TypeScript syntax
import { LocaleProvider } from 'k2-react-translate';
import { App } from './App';
import translations from './translations.json';ReactDOM.render(
,
document.getElementById('app')
);
```## Documentation (Translation)
`k2-react-translate` barrels (re-exports) the following sub-modules as named exports:
### `` - [source](https://github.com/kareemkibue/k2-react-translate/blob/master/src/LocaleProvider.tsx)
A Context API wrapper to ``. This wrapper is required to apply
`k2-react-translate` translations.| Props | Type | Description |
| ----------------- | ----------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `translations` | Object (required) | See [translations under Project Setup](#Project-Setup) |
| `languages` | Array (required) | An array of language codes. Used as [`params`](https://scotch.io/courses/using-react-router-4/route-params) to `react-router` if you choose to incorporate localized routing into your app. |
| `defaultLanguage` | string (optional) | Default language, Must be included in the `languages` array above |
| `localizedUrls` | boolean (optional), default={false} | Option to localize URLs with every route change, page reload or language change. Additionally, add a `` wrapper from `react-router`, meaning this need not be done in your `RouterConfig` |#### Usage
See [Project Setup](Project-Setup) above.
---
### `useTranslate` - [source](https://github.com/kareemkibue/k2-react-translate/blob/master/src/useTranslate.ts)
A custom hook for use in Function Components.
**Dependencies:** `react@^16.8.0`, `react-dom@^16.8.0`
#### Usage
```tsx
// MyComponent.tsx
import * as React from 'react'; // standard TypeScript syntax
import { useTranslate } from 'k2-react-translate';const links = {
en: '/en/privacy-policy',
fr: '/fr/privacy-policy',
};const MyComponent: React.FunctionComponent<{}> = () => {
const { translate, translateAndParse, language, changeLanguage } = useTranslate();const handleClick = (): void => {
// change language to French
changeLanguage('fr');
};return (
{translate('HELLO')}
// "Hello" (en) - string // "Bonjour" (fr) - string
{translate('HELLO_NAME', { firstName: 'Jaqen' })}
// "Hello, Jaqen" (en) - string // "Bonjour, Jaqen" (fr) - string
{translateAndParse('PRIVACY_POLICY', { link: links[language] })}
// Privacy Policy (en) - ReactElement //
Politique de Confidentialité
(fr) - ReactElement
Change Language
);
};
```---
### `` - [source](https://github.com/kareemkibue/k2-react-translate/blob/master/src/Translator.tsx)
A React component that wraps `` that performs translations, given
translation keys as prop arguments.If using `react v16.8.0+`, I'd strongly recommend using the `useTranslate` hook
[above](#usetranslate---source) instead. `useTranslate` works in the same way but provides for
cleaner and less verbose use.| Props | Type | Description |
| ----------- | ----------------------------------- | ---------------------------------------------------------------------------- |
| `id` | string (optional) | translation key |
| `vars` | object (optional) | dynamic translation variables, set outside the `translations.json` |
| `render` | function (optional) | render prop, returning `(language:string)=>ReactNode` |
| `parseHtml` | boolean (optional), `default=false` | option to sanitize and parse stringified HTML set in the `translations.json` |#### Usage
```tsx
// MyComponent.tsx
import * as React from 'react'; // standard TypeScript syntax
import { Translator } from 'k2-react-translate';const links = {
en: '/en/privacy-policy',
fr: '/fr/privacy-policy',
};const MyComponent: React.StatelessComponent<{}> = () => {
return (
// "Hello" (en) - string // "Bonjour" (fr) - string
// "Hello, Jaqen" (en) - string // "Bonjour, Jaqen" (fr) - string
// Privacy Policy (en) - ReactElement //
Politique de Confidentialité
(fr) - ReactElement
);
};
```---
### `` - [source](https://github.com/kareemkibue/k2-react-translate/blob/master/src/LanguageSwitcher.tsx)
A button wrapped React component that provides the ability to set languages.
Switching languages can alternatively be performed under an exposed function in the `useTranslate`
hook documented [here](#usetranslate---source).| Props | Type | Description |
| --------- | -------- | ---------------- |
| `onClick` | Function | Synthentic event |#### Usage
```tsx
// MyComponent.tsx
import * as React from 'react'; // standard TypeScript syntax
import { LanguageSwitcher } from 'k2-react-translate';const MyComponent: React.FunctionComponent<{}>=()=>{
const handleClick:(changeLanguage: (language:string)=> void ): void=>{
// change language to French
changeLanguage('fr');
}return
;
}
```## Documentation (Localized Routing)
Localized routing is optional. If used, `react-router-dom` would need to be installed as a
production dependency.### `` - [source](https://github.com/kareemkibue/k2-react-translate/blob/master/src/LocalizedRoutes.tsx)
Provides a simple, non-intrusive way of setting up localized routes. Returns localized ``s
or ``s components given the `routes` prop config.If `` is used, you need not wrap your RouterConfig with `` or
`` as this is done within ``.`` is not recursive.
| Props | Type | Description |
| ------------- | ----------------------------------- | -------------------------------------------------------------------------- |
| `routes` | Array (required) | Based on `react-router-dom`'s props |
| `localize` | Boolean (optional), default=`true` | Option to localize URLs (prepend the language code in the URL) |
| `applySwitch` | Boolean (optional), default=`false` | Wrap the Route config with `` components. Required in most cases. |#### Usage
```tsx
// RouteConfig.tsx
import * as React from 'react'; // standard TypeScript syntax
import { LocalizedRoutes, Route } from 'k2-react-translate';const RouteConfig: React.FunctionComponent<{}> = () => {
const routes: Route[] = [
{
path: '/', // resolves to /:language/, unless localize={false}
exact: true,
component: Home,
},
{
path: '/about', // resolves to /:language/about, unless localize={false}
component: About,
localize: true, // should override
},
{
// Redirect props
to: '/page-not-found', // resolves to /:language/page-not-found, unless localize={false}
},
];return (
);
};
```---
### `` - [source](https://github.com/kareemkibue/k2-react-translate/blob/master/src/LocalizedRoute.tsx)
A localized wrapper to `react-router-dom`'s `Route`.
You wouldn't need to use `` if [``](#localizedroutes---source) is
configured.| Props | Type | Description |
| ---------- | ---------------------------------- | ------------------------------------------------------------------------------------------- |
| ...props | Object | Standard `` component [props](https://reacttraining.com/react-router/web/api/Route) |
| `localize` | Boolean (optional), default=`true` | Option to localize URLs (prepend the language code in the URL) |#### Usage
```tsx
// MyComponent.tsx
import * as React from 'react'; // standard TypeScript syntax
import { LocalizedRoute } from 'k2-react-translate';
import { Home } from './Home';
import { About } from './About';const MyComponent: React.FunctionComponent<{}> = () => {
return (
// automatically resolves to the "/:language" // if "en" is active, then "/en"
// automatically resolves to the "/:language/about-us" // if "en" is active, then
"/en/about-us"
);
};
```---
### `` - [source](https://github.com/kareemkibue/k2-react-translate/blob/master/src/LocalizedLink.tsx)
A localized wrapper to `react-router-dom`'s ``.
| Props | Type | Description |
| ---------- | ---------------------------------- | ----------------------------------------------------------------------------------------- |
| ...props | Object | Standard `` component [props](https://reacttraining.com/react-router/web/api/Link) |
| `localize` | Boolean (optional), default=`true` | Option to localize URLs (prepend the language code in the URL) |#### Usage
```tsx
// MyComponent.tsx
import * as React from 'react'; // standard TypeScript syntax
import { LocalizedLink } from 'k2-react-translate';const MyComponent: React.FunctionComponent<{}> = () => {
return (
// automatically resolves to the "/:language/about-us" // if "en" is active, then
"/en/about-us"
About Us
);
};
```---
### `` - [source](https://github.com/kareemkibue/k2-react-translate/blob/master/src/LocalizedRedirect.tsx)
A localized wrapper to `react-router-dom`'s ``.
| Props | Type | Description |
| ---------- | ---------------------------------- | ------------------------------------------------------------------------------------------------- |
| ...props | Object | Standard `` component [props](https://reacttraining.com/react-router/web/api/Redirect) |
| `localize` | Boolean (optional), default=`true` | Option to localize URLs (prepend the language code in the URL) |#### Usage
```tsx
// MyComponent.tsx
import * as React from 'react'; // standard TypeScript syntax
import { LocalizedRedirect } from 'k2-react-translate';const MyComponent: React.FunctionComponent<{}> = () => {
// automatically resolves to the "/:language/page-not-found"
// if "en" is active, then "/en/page-not-found"
if (someCondition) {
return About Us;
}// alternatively, `props.history.push` would also resolve URLs by current langauge
return
;
};
```## Development
- Run `yarn` on the root of the repository.
- Run `yarn start` to start the project.
- Run `yarn test:watch` to ammend tests.## Known Issues
- Routing works with `` for now
- `` is yet to be adapted from ``. `` works equally as
well, with the exception of the `activeClassName` prop.