https://github.com/keenethics/svelte-notifications
Simple and flexible notifications system
https://github.com/keenethics/svelte-notifications
notifications svelte svelte-components toast
Last synced: about 2 months ago
JSON representation
Simple and flexible notifications system
- Host: GitHub
- URL: https://github.com/keenethics/svelte-notifications
- Owner: keenethics
- License: mit
- Created: 2019-05-21T20:18:44.000Z (about 6 years ago)
- Default Branch: master
- Last Pushed: 2024-02-06T15:57:03.000Z (over 1 year ago)
- Last Synced: 2025-05-11T04:24:20.846Z (about 2 months ago)
- Topics: notifications, svelte, svelte-components, toast
- Language: JavaScript
- Homepage: https://svelte-notifications.netlify.com
- Size: 1.24 MB
- Stars: 581
- Watchers: 6
- Forks: 30
- Open Issues: 10
-
Metadata Files:
- Readme: README.md
- Contributing: CONTRIBUTING.md
- License: LICENSE
Awesome Lists containing this project
README


# Svelte notifications
Simple and flexible notifications system for Svelte 3
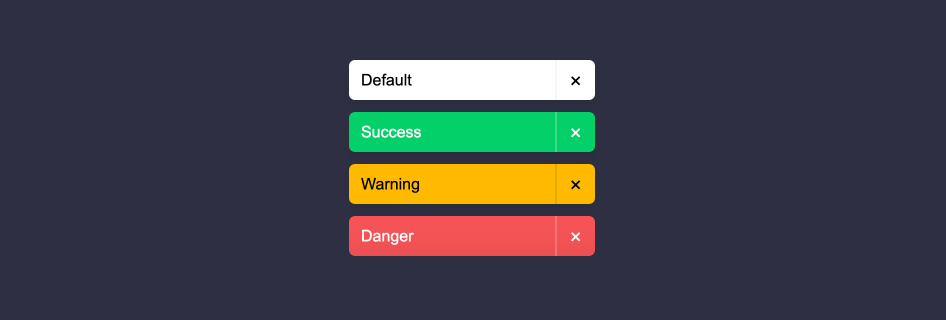
## Demonstration
[https://svelte-notifications.netlify.com](https://svelte-notifications.netlify.com)
## Getting started
```bash
npm install --save svelte-notifications
```## Basic usage
```svelte
// MainComponent.svelteimport Notifications from 'svelte-notifications';
import App from './App.svelte';
```
```svelte
// ChildrenComponent.svelteimport { getNotificationsContext } from 'svelte-notifications';
const { addNotification } = getNotificationsContext();
addNotification({
text: 'Notification',
position: 'bottom-center',
})}
>
Add notification```
## Providing custom notification component
```svelte
// MainComponent.svelteimport Notifications from 'svelte-notifications';
import CustomNotification from './CustomNotification.svelte';import App from './App.svelte';
```
```svelte
// CustomNotification.svelteexport let notification = {};
// `onRemove` function will be passed into your component.
export let onRemove = null;const handleButtonClick = () => {
onRemove();
}
{notification.heading}
{notification.description}
Close me
``````svelte
// AnotherComponent.svelteimport { getNotificationsContext } from 'svelte-notifications';
const { addNotification } = getNotificationsContext();
const handleButtonClick = () => {
addNotification({
position: 'bottom-right',
heading: 'hi i am custom notification',
type: 'error',
description: 'lorem ipsum',
});
}
Show notification
```## API
#### `Notifications`
The `Notifications` component supplies descendant components with notifications store through context.
- @prop {component} `[item=null]` - Custom notification component that receives the notification object
- @prop {boolean} `[withoutStyles=false]` - If you don't want to use the default styles, this flag will remove the classes to which the styles are attached
- @prop {string|number} `[zIndex]` - Adds a style with z-index for the notification container```svelte
// MainComponent.svelteimport Notifications from 'svelte-notifications';
import App from './App.svelte';
```
#### `getNotificationsContext`
A function that allows you to access the store and the functions that control the store.
```svelte
// ChildrenComponent.svelteimport { getNotificationsContext } from 'svelte-notifications';
const notificationsContext = getNotificationsContext();
const {
addNotification,
removeNotification,
clearNotifications,
subscribe,
} = notificationsContext;```
#### `getNotificationsContext:addNotification`
You can provide any object that the notification component will receive. The default object looks like this:
- @param {Object} `notification` - The object that will receive the notification component
- @param {string} `[id=timestamp-rand]` - Unique notification identificator
- @param {string} `text` – Notification text
- @param {string} `[position=bottom-center]` – One of these values: `top-left`, `top-center`, `top-right`, `bottom-left`, `bottom-center`, `bottom-right`
- @param {string} `type` – One of these values: `success`, `warning`, `error`
- @param {number} `[removeAfter]` – After how much the notification will disappear (in milliseconds)```svelte
// ChildrenComponent.svelteimport { getNotificationsContext } from 'svelte-notifications';
const { addNotification } = getNotificationsContext();
addNotification({
id: 'uniqNotificationId',
text: 'Notification',
position: 'top-center',
type: 'success',
removeAfter: 4000,
...rest,
});```
#### `getNotificationsContext:removeNotification`
- @param {string} `id` - Unique notification identificator
```svelte
// ChildrenComponent.svelteimport { getNotificationsContext } from 'svelte-notifications';
const { removeNotification } = getNotificationsContext();
removeNotification('uniqNotificationId');
```
#### `getNotificationsContext:clearNotifications`
```svelte
// ChildrenComponent.svelteimport { getNotificationsContext } from 'svelte-notifications';
const { clearNotifications } = getNotificationsContext();
clearNotifications();
```
#### `getNotificationsContext:subscribe`
Default Svelte subscribe method that allows interested parties to be notified whenever the store value changes
## Contributing
Read more about contributing [here](/CONTRIBUTING.md)