Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/kyranet/canvas-constructor
An ES6 utility for canvas with built-in functions and chained methods.
https://github.com/kyranet/canvas-constructor
canvas chain-methods draw font-rendering image-generation image-rendering node-canvas
Last synced: 15 days ago
JSON representation
An ES6 utility for canvas with built-in functions and chained methods.
- Host: GitHub
- URL: https://github.com/kyranet/canvas-constructor
- Owner: kyranet
- License: mit
- Created: 2017-08-05T21:08:43.000Z (over 7 years ago)
- Default Branch: main
- Last Pushed: 2024-05-22T20:56:38.000Z (9 months ago)
- Last Synced: 2024-05-23T01:15:04.683Z (9 months ago)
- Topics: canvas, chain-methods, draw, font-rendering, image-generation, image-rendering, node-canvas
- Language: HTML
- Homepage: https://canvasconstructor.js.org
- Size: 12.5 MB
- Stars: 114
- Watchers: 4
- Forks: 24
- Open Issues: 5
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- Contributing: .github/CONTRIBUTING.md
- License: LICENSE.md
- Code of conduct: .github/CODE_OF_CONDUCT.md
- Codeowners: .github/CODEOWNERS
- Security: .github/SECURITY.md
Awesome Lists containing this project
README
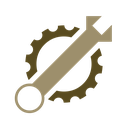
# canvas-constructor
**A utility for Canvas with chainable methods and consistent interface for all environments**
[](https://www.npmjs.com/package/canvas-constructor)
[](https://www.npmjs.com/package/canvas-constructor)
[](https://raw.githubusercontent.com/kyranet/canvas-constructor/master/LICENSE)[](https://discord.gg/taNgb9d)
---
## Live Demo
[](https://stackblitz.com/edit/canvas-constructor-vite?file=main.js)
## Installation
This module requires one of the following packages to be installed for Node.js:
- [`@napi-rs/canvas`](https://www.npmjs.com/package/@napi-rs/canvas)
- [`skia-canvas`](https://www.npmjs.com/package/skia-canvas)
- [`canvas`](https://www.npmjs.com/package/canvas)> **Note**: If you are building a website, no extra dependencies are required.
---
How to use it:
**Node.js**:
```js
const { Canvas } = require('canvas-constructor/napi-rs');
// or 'canvas-constructor/skia' if you are using `skia-canvas`
// or 'canvas-constructor/cairo' if you are using `canvas`new Canvas(300, 300)
.setColor('#AEFD54')
.printRectangle(5, 5, 290, 290)
.setColor('#FFAE23')
.setTextFont('28px Impact')
.printText('Hello World!', 130, 150)
.png();
```**Browser**:
```html
const canvasElement = document.getElementById('canvas');
new CanvasConstructor.Canvas(canvasElement)
.setColor('#AEFD54')
.printRectangle(5, 5, 290, 290)
.setColor('#FFAE23')
.setTextFont('28px Impact')
.printText('Hello World!', 130, 150);```
Alternatively, you can import `canvas-constructor/browser` if you are using a bundler such as Vite, Webpack, or Rollup:
```js
import { Canvas } from 'canvas-constructor/browser';
```Now, let's suppose we want to add images, we can use the `loadImage` function, which works in all supported environments:
```js
const { Canvas, loadImage } = require('canvas-constructor/napi-rs');async function createCanvas() {
const image = await loadImage('./images/kitten.png');return new Canvas(300, 400)
.printImage(image, 0, 0, 300, 400)
.setColor('#FFAE23')
.setTextFont('28px Impact')
.setTextAlign('center')
.printText('Kitten!', 150, 370)
.pngAsync();
}
```And now, you have created an image with a kitten in the background and some centered text at the bottom of it.
If you experience issues with `@napi-rs/canvas`, `skia-canvas`, or `canvas`, please refer to their respective package repositories, this
package is just a convenient wrapper that makes it easier to use the canvas in both Node.js and the browser. And does not modify the
behavior of the underlying canvas implementation.