Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/labex-labs/cpp-practice-labs
[C++ Practice Labs] This repository collects 59 of programming scenarios (labs and challenges) for C++ Practice Labs. This course contains lots of labs for C++, each lab is a small C++ project with detailed guidance and solutions. You can practice your C++ skills by completing these labs, improve...
https://github.com/labex-labs/cpp-practice-labs
List: cpp-practice-labs
awesome awesome-list challenges course cpp education hands-on labex labs programming
Last synced: 3 months ago
JSON representation
[C++ Practice Labs] This repository collects 59 of programming scenarios (labs and challenges) for C++ Practice Labs. This course contains lots of labs for C++, each lab is a small C++ project with detailed guidance and solutions. You can practice your C++ skills by completing these labs, improve...
- Host: GitHub
- URL: https://github.com/labex-labs/cpp-practice-labs
- Owner: labex-labs
- Created: 2024-05-24T09:03:19.000Z (9 months ago)
- Default Branch: master
- Last Pushed: 2024-08-01T01:28:54.000Z (7 months ago)
- Last Synced: 2024-08-02T04:23:52.236Z (7 months ago)
- Topics: awesome, awesome-list, challenges, course, cpp, education, hands-on, labex, labs, programming
- Homepage: https://labex.io/skilltrees/cpp
- Size: 9.77 KB
- Stars: 2
- Watchers: 3
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# C++ Practice Labs
[](https://labex.io/courses/cpp-practice-labs)
[](https://labex.io/courses/cpp-practice-labs)
This course contains lots of labs for C++, each lab is a small C++ project with detailed guidance and solutions. You can practice your C++ skills by completing these labs, improve your coding skills, and learn how to write clean and efficient code.

## Environment
LabEx is an interactive, hands-on learning platform dedicated to coding and technology. It combines labs, AI assistance, and virtual machines to provide a no-video, practical learning experience.
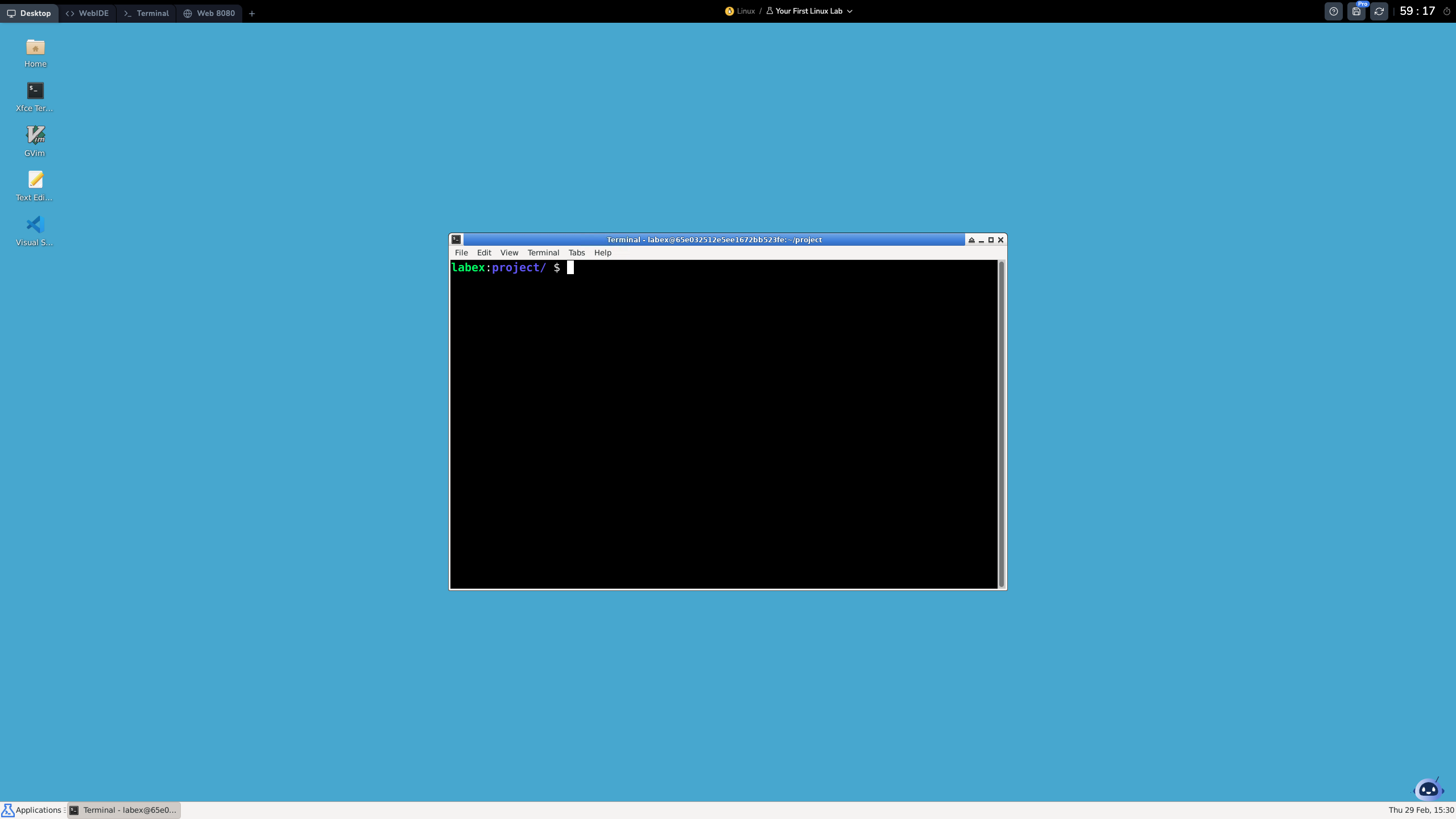
- A strict βLearn by Doingβ approach with exclusive hands-on labs and no videos.
- Interactive online environments within the browser, with automated step-by-step checks.
- A structured content organization with the Skill Tree based learning system.
- A growing learning resource of 30 Skill Trees and over 6,000 Labs.
- The AI learning assistant Labby, built on ChatGPT, providing a conversational learning experience.Learn more about [LabEx VM](https://support.labex.io/using-labex/virtual-machine).
## Exercises
| Index | Name | Difficulty | Practice |
|---------|-----------------------------------------------------|--------------|------------------------------------------------------------------------------------------------------------------------------|
| 01 | π Determine String Length in C++ | β ββ | Start Lab |
| 02 | π C++ Multiset in STL | β ββ | Start Lab |
| 03 | π Finding Average of N User Input Numbers | β ββ | Start Lab |
| 04 | π Draw a Perfect Christmas Tree Using C++ | β ββ | Start Lab |
| 05 | π Crafting C++ Diamond Pattern | β ββ | Start Lab |
| 06 | π C++ STL Map Erase Method | β ββ | Start Lab |
| 07 | π Half Pyramid Pattern Printing in C++ | β ββ | Start Lab |
| 08 | π Program to Find Divisor of a Number | β ββ | Start Lab |
| 09 | π Counting Characters in a File Using C++ | β ββ | Start Lab |
| 10 | π Convert String to Array of Characters | β ββ | Start Lab |
| 11 | π Print a Pattern Series | β ββ | Start Lab |
| 12 | π Reading and Summing Numbers From a File | β ββ | Start Lab |
| 13 | π Program to Print Fibonacci Series in CPP | β ββ | Start Lab |
| 14 | π Find the Maximum Element of the Stack | β ββ | Start Lab |
| 15 | π CPP Binary Search Using Dynamic Array | β ββ | Start Lab |
| 16 | π Adding Two Numbers Program | β ββ | Start Lab |
| 17 | π Swap Two Numbers Using a 3rd Variable | β ββ | Start Lab |
| 18 | π Perform Arithmetic Operations Using Functions | β ββ | Start Lab |
| 19 | π C++ Half Pyramid Pattern Using Characters | β ββ | Start Lab |
| 20 | π Initializing a Vector in STL Using C++ | β ββ | Start Lab |
| 21 | π CPP Program to Print Reverse Half Pyramid | β ββ | Start Lab |
| 22 | π Program to Print Full Pyramid Using CPP | β ββ | Start Lab |
| 23 | π Storing and Displaying Employee Information | β ββ | Start Lab |
| 24 | π Compute Sum of Squares in C++ | β ββ | Start Lab |
| 25 | π Bubble Sort Using Dynamic Array | β ββ | Start Lab |
| 26 | π Initializing a Vector | β ββ | Start Lab |
| 27 | π C++ Class Implementation | β ββ | Start Lab |
| 28 | π C++ Using STL Unordered Set | β ββ | Start Lab |
| 29 | π Calculate Sum of Reciprocal Series | β ββ | Start Lab |
| 30 | π Determine Perfect Square | β ββ | Start Lab |
| 31 | π C++ Half Pyramid Pattern Using Star Program | β ββ | Start Lab |
| 32 | π C++ STL Set Find Method | β ββ | Start Lab |
| 33 | π Determining Integer Digit Count in C++ | β ββ | Start Lab |
| 34 | π C++ Program for FCFS Scheduling Algorithm | β ββ | Start Lab |
| 35 | π C++ STL Stack | β ββ | Start Lab |
| 36 | π Custom Sort Method for STL Pair Template | β ββ | Start Lab |
| 37 | π Find GCD and LCM | β ββ | Start Lab |
| 38 | π CPP Program to Print a Pascal Triangle | β ββ | Start Lab |
| 39 | π File Content Copying in C++ | β ββ | Start Lab |
| 40 | π Sort Unordered Set Using STL | β ββ | Start Lab |
| 41 | π Sorting Strings by Length in C++ | β ββ | Start Lab |
| 42 | π Locating First Occurrence in Sorted Array | β ββ | Start Lab |
| 43 | π Swap Two Numbers Using Functions | β ββ | Start Lab |
| 44 | π Find Maximum Number in Three Given Numbers | β ββ | Start Lab |
| 45 | π CPP Program to Calculate Standard Deviation | β ββ | Start Lab |
| 46 | π Determine Prime Number in C++ | β ββ | Start Lab |
| 47 | π Minimum Element in Rotated Sorted Vector | β ββ | Start Lab |
| 48 | π Insertion Sort Using Dynamic Array | β ββ | Start Lab |
| 49 | π C++ Method Overloading | β ββ | Start Lab |
| 50 | π C++ Program to Find Greatest Number | β ββ | Start Lab |
| 51 | π Prime Number Identification in C++ | β ββ | Start Lab |
| 52 | π Flip Pattern Half Pyramid in C++ | β ββ | Start Lab |
| 53 | π Multiplication of Two Matrices | β ββ | Start Lab |
| 54 | π C++ Reverse Half Pyramid Pattern Using Characters | β ββ | Start Lab |
| 55 | π Implementing Dynamic Polymorphism in C++ | β ββ | Start Lab |
| 56 | π Video Object Tracking by Using OpenCV | β ββ | Start Lab |
| 57 | π C++ Hierarchical Inheritance Program | β ββ | Start Lab |
| 58 | π Read and Write File Line by Line in C++ | β ββ | Start Lab |
| 59 | π Memory Leak Detector with C++ | β ββ | Start Lab |## More
- π [C++ Programming Courses](https://github.com/labex-labs/awesome-programming-courses)
- π [C++ Programming Projects](https://github.com/labex-labs/awesome-programming-projects)
- π [C++ Free Tutorials](https://github.com/labex-labs/cpp-free-tutorials)