https://github.com/labex-labs/go-practice-challenges
Go Practice Challenges | This repo collects 80 of programming labs exercises for Go Practice Challenges. This course contains lots of challenges for Go, each challenge is a small Go project with detailed instructions and solutions. You can practice your Go skills by solving these challenges, impr...
https://github.com/labex-labs/go-practice-challenges
List: go-practice-challenges
challenges course exercises golang hands-on labex labs playgroud programming
Last synced: 2 months ago
JSON representation
Go Practice Challenges | This repo collects 80 of programming labs exercises for Go Practice Challenges. This course contains lots of challenges for Go, each challenge is a small Go project with detailed instructions and solutions. You can practice your Go skills by solving these challenges, impr...
- Host: GitHub
- URL: https://github.com/labex-labs/go-practice-challenges
- Owner: labex-labs
- Created: 2024-05-24T09:04:18.000Z (about 1 year ago)
- Default Branch: master
- Last Pushed: 2025-01-01T01:55:51.000Z (7 months ago)
- Last Synced: 2025-04-29T13:05:02.334Z (3 months ago)
- Topics: challenges, course, exercises, golang, hands-on, labex, labs, playgroud, programming
- Homepage: https://labex.io/courses/go-practice-challenges
- Size: 27.3 KB
- Stars: 0
- Watchers: 2
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# Go Practice Challenges
[](https://labex.io/courses/go-practice-challenges)
[](https://labex.io/courses/go-practice-challenges)
This course contains lots of challenges for Go, each challenge is a small Go project with detailed instructions and solutions. You can practice your Go skills by solving these challenges, improve your problem-solving skills, and learn how to write clean and efficient code.

## Environment
LabEx is an interactive, hands-on learning platform dedicated to coding and technology. It combines labs, AI assistance, and virtual machines to provide a no-video, practical learning experience.
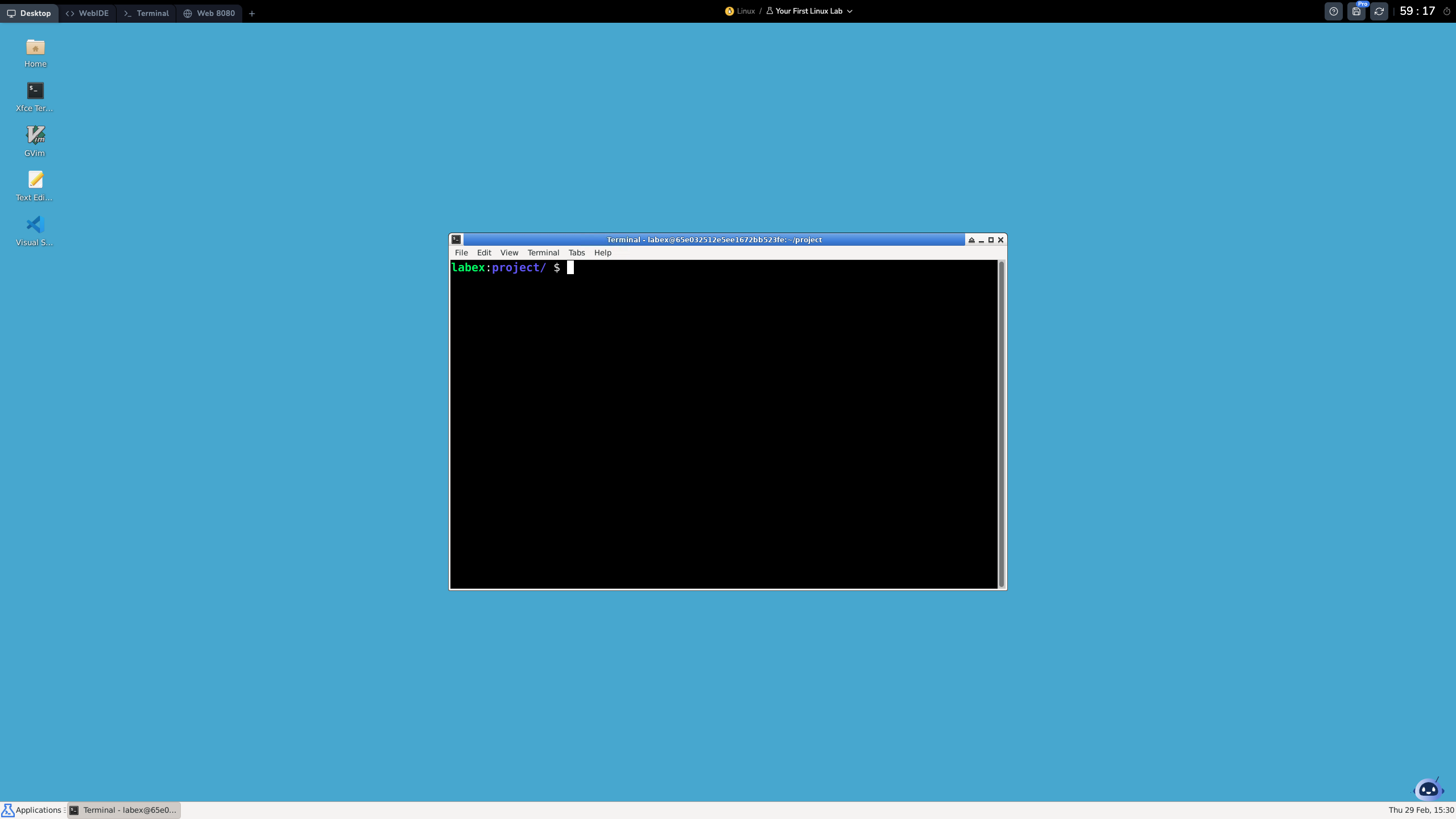
- A strict βLearn by Doingβ approach with exclusive hands-on labs and no videos.
- Interactive online environments within the browser, with automated step-by-step checks.
- A structured content organization with the Skill Tree based learning system.
- A growing learning resource of 30 Skill Trees and over 6,000 Labs.
- The AI learning assistant Labby, built on ChatGPT, providing a conversational learning experience.Learn more about [LabEx VM](https://support.labex.io/using-labex/virtual-machine).
## Exercises
| Index | Name | Difficulty | Practice |
|---------|---------------------------------------------------------|--------------|-----------------------------------------------------------------------------------------------------------------------------------------|
| 01 | π― Go Fundamentals Value Types | β ββ | Start Challenge |
| 02 | π― Golang Constants Programming Challenge | β ββ | Start Challenge |
| 03 | π― Golang Variable Manipulation | β ββ | Start Challenge |
| 04 | π― Hello World, Golang! | β ββ | Start Challenge |
| 05 | π― Golang String Formatting | β ββ | Start Challenge |
| 06 | π― Golang String Manipulation | β ββ | Start Challenge |
| 07 | π― Strings and Runes | Challenge | β ββ | Start Challenge |
| 08 | π― Array Manipulation in Go | β ββ | Start Challenge |
| 09 | π― Go Slice Data Type | β ββ | Start Challenge |
| 10 | π― Creating and Manipulating Go Maps | Challenge | β ββ | Start Challenge |
| 11 | π― Go Struct Data Grouping | β ββ | Start Challenge |
| 12 | π― Golang Pointers Challenge: Understanding Reference... | β ββ | Start Challenge |
| 13 | π― Golang For Loop | β ββ | Start Challenge |
| 14 | π― Golang If-Else Branching | β ββ | Start Challenge |
| 15 | π― Concise Introduction to Switch Statement | β ββ | Start Challenge |
| 16 | π― Go Functions Fundamentals | β ββ | Start Challenge |
| 17 | π― Multiple Return Values | Challenge | β ββ | Start Challenge |
| 18 | π― Variadic Functions in Go | β ββ | Start Challenge |
| 19 | π― Go Programming Method | β ββ | Start Challenge |
| 20 | π― Golang Interface Exploration | β ββ | Start Challenge |
| 21 | π― Embedding Structs in Golang | β ββ | Start Challenge |
| 22 | π― Exploring Golang's Range Keyword | β ββ | Start Challenge |
| 23 | π― Handling Errors in Golang | Challenge | β ββ | Start Challenge |
| 24 | π― Handling Unexpected Errors in Golang | β ββ | Start Challenge |
| 25 | π― Deferred Function Execution Cleanup | β ββ | Start Challenge |
| 26 | π― Handling Panics with Golang's Recover | β ββ | Start Challenge |
| 27 | π― Golang Generics Introduction | β ββ | Start Challenge |
| 28 | π― Creating Closures with Anonymous Functions in Go |... | β ββ | Start Challenge |
| 29 | π― Recursive Functions in Go | β ββ | Start Challenge |
| 30 | π― Concurrent Goroutines in Golang | β ββ | Start Challenge |
| 31 | π― Golang Buffered Channels | β ββ | Start Challenge |
| 32 | π― Golang Channel Function Parameters | Challenge | β ββ | Start Challenge |
| 33 | π― Synchronize Goroutines with Channels | β ββ | Start Challenge |
| 34 | π― Go Channels Concurrency | β ββ | Start Challenge |
| 35 | π― Golang Channel Communication with Workers | Challe... | β ββ | Start Challenge |
| 36 | π― Non-Blocking Channel Operations | Challenge | β ββ | Start Challenge |
| 37 | π― Range Over Channels | Challenge | β ββ | Start Challenge |
| 38 | π― Go Select Statement | β ββ | Start Challenge |
| 39 | π― Implementing Timeouts in Go Using Channels | β ββ | Start Challenge |
| 40 | π― Golang Timer and Ticker | β ββ | Start Challenge |
| 41 | π― Timers and Tickers | Challenge | β ββ | Start Challenge |
| 42 | π― Concurrent Worker Pool with Goroutines | β ββ | Start Challenge |
| 43 | π― Using WaitGroup in Goroutines | β ββ | Start Challenge |
| 44 | π― Implementing Rate Limiting in Go | Challenge | β ββ | Start Challenge |
| 45 | π― Atomic Counters in Concurrent Go Programming | β ββ | Start Challenge |
| 46 | π― Concurrent Data Access with Mutexes | Challenge | β ββ | Start Challenge |
| 47 | π― Synchronize Shared State with Goroutines | β ββ | Start Challenge |
| 48 | π― Reading Files in Go | Challenge | β ββ | Start Challenge |
| 49 | π― Write Files in Go | Challenge | β ββ | Start Challenge |
| 50 | π― Concise Go Text Transformation | β ββ | Start Challenge |
| 51 | π― File Path Handling in Golang | Challenge | β ββ | Start Challenge |
| 52 | π― Working with Directories in Go | Challenge | β ββ | Start Challenge |
| 53 | π― Temporary Files and Directories | Challenge | β ββ | Start Challenge |
| 54 | π― Embedding Files in Go Programs | β ββ | Start Challenge |
| 55 | π― Sorting Built-in Types in Go | Challenge | β ββ | Start Challenge |
| 56 | π― Sorting by Functions | Challenge | β ββ | Start Challenge |
| 57 | π― Golang Text Template Dynamic Content | β ββ | Start Challenge |
| 58 | π― Go Regular Expression Demonstration | β ββ | Start Challenge |
| 59 | π― Encoding and Decoding JSON in Golang | β ββ | Start Challenge |
| 60 | π― Golang XML Mapping | β ββ | Start Challenge |
| 61 | π― Go Time and Duration | β ββ | Start Challenge |
| 62 | π― Golang Epoch Time | β ββ | Start Challenge |
| 63 | π― Time Formatting and Parsing | Challenge | β ββ | Start Challenge |
| 64 | π― Random Number Generation in Go | Challenge | β ββ | Start Challenge |
| 65 | π― Parse Numbers in Go | Challenge | β ββ | Start Challenge |
| 66 | π― Parsing URLs in Go | Challenge | β ββ | Start Challenge |
| 67 | π― Compute SHA256 Hashes in Go | Challenge | β ββ | Start Challenge |
| 68 | π― Base64 Encoding Challenge in Go | β ββ | Start Challenge |
| 69 | π― Testing and Benchmarking | Challenge | β ββ | Start Challenge |
| 70 | π― Command-Line Argument Handling in Go | Challenge | β ββ | Start Challenge |
| 71 | π― Command-Line Flag Parsing in Golang | β ββ | Start Challenge |
| 72 | π― Command Line Subcommands | Challenge | β ββ | Start Challenge |
| 73 | π― Unix Environment Variables | β ββ | Start Challenge |
| 74 | π― Golang HTTP Request Handling | Challenge | β ββ | Start Challenge |
| 75 | π― Build Basic HTTP Server in Go | β ββ | Start Challenge |
| 76 | π― Golang Context Cancellation Demonstration | Challe... | β ββ | Start Challenge |
| 77 | π― Replacing Go Process with Exec Function | β ββ | Start Challenge |
| 78 | π― Spawning External Processes in Go | Challenge | β ββ | Start Challenge |
| 79 | π― Handling Unix Signals in Go Programs | β ββ | Start Challenge |
| 80 | π― Exit Challenge in Go | β ββ | Start Challenge |## More
- π [ Programming Courses](https://github.com/labex-labs/awesome-programming-courses)
- π [ Programming Projects](https://github.com/labex-labs/awesome-programming-projects)
- π [ Free Tutorials](https://github.com/labex-labs/go-free-tutorials)