Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/labex-labs/quick-start-with-algorithm
[Quick Start with Algorithm] This repository collects 90 of programming scenarios (labs and challenges) for Quick Start with Algorithm. This course offers numerous interactive coding challenges to help you comprehend and implement algorithms quickly. The challenges were designed by Donne Martin a...
https://github.com/labex-labs/quick-start-with-algorithm
List: quick-start-with-algorithm
algorithm awesome awesome-list challenges course education hands-on labex labs programming python
Last synced: 5 days ago
JSON representation
[Quick Start with Algorithm] This repository collects 90 of programming scenarios (labs and challenges) for Quick Start with Algorithm. This course offers numerous interactive coding challenges to help you comprehend and implement algorithms quickly. The challenges were designed by Donne Martin a...
- Host: GitHub
- URL: https://github.com/labex-labs/quick-start-with-algorithm
- Owner: labex-labs
- Created: 2024-05-22T07:02:01.000Z (6 months ago)
- Default Branch: master
- Last Pushed: 2024-08-01T01:26:32.000Z (4 months ago)
- Last Synced: 2024-08-01T04:21:36.531Z (4 months ago)
- Topics: algorithm, awesome, awesome-list, challenges, course, education, hands-on, labex, labs, programming, python
- Homepage: https://labex.io/skilltrees/python
- Size: 27.3 KB
- Stars: 0
- Watchers: 2
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# Quick Start with Algorithm
[](https://labex.io/courses/quick-start-with-algorithm)
[](https://labex.io/courses/quick-start-with-algorithm)
This course offers numerous interactive coding challenges to help you comprehend and implement algorithms quickly. The challenges were designed by Donne Martin and are available in the GitHub repository donnemartin/interactive-coding-challenges.

## Environment
LabEx is an interactive, hands-on learning platform dedicated to coding and technology. It combines labs, AI assistance, and virtual machines to provide a no-video, practical learning experience.
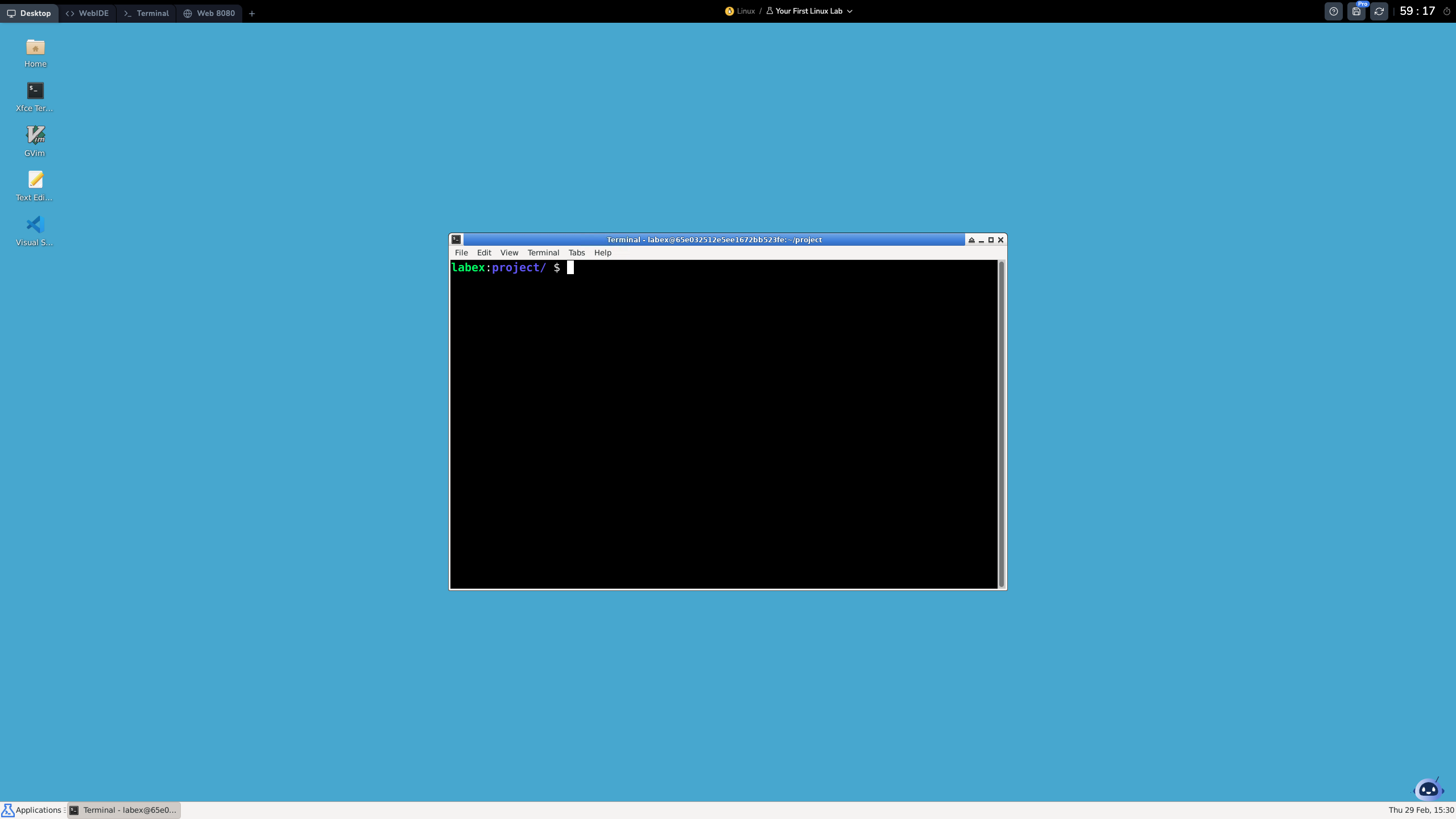
- A strict βLearn by Doingβ approach with exclusive hands-on labs and no videos.
- Interactive online environments within the browser, with automated step-by-step checks.
- A structured content organization with the Skill Tree based learning system.
- A growing learning resource of 30 Skill Trees and over 6,000 Labs.
- The AI learning assistant Labby, built on ChatGPT, providing a conversational learning experience.Learn more about [LabEx VM](https://support.labex.io/using-labex/virtual-machine).
## Exercises
| Index | Name | Difficulty | Practice |
|---------|---------------------------------------------------------|--------------|-------------------------------------------------------------------------------------------------------------------------------------------|
| 01 | π― Permutation Check of String Pairs | β ββ | Start Challenge |
| 02 | π― Solving the Two Sum Problem | β ββ | Start Challenge |
| 03 | π― Compress String Using Python | β ββ | Start Challenge |
| 04 | π― Implement Hash Table with Key-Value Operations | β ββ | Start Challenge |
| 05 | π― Find Differing Character in Strings | β ββ | Start Challenge |
| 06 | π― Implement Fizz Buzz in Python | β ββ | Start Challenge |
| 07 | π― String Compression Challenge | β ββ | Start Challenge |
| 08 | π― Reverse String In-Place Programming | β ββ | Start Challenge |
| 09 | π― Unique Character String Validation | β ββ | Start Challenge |
| 10 | π― Determine String Rotation in Computer Science | β ββ | Start Challenge |
| 11 | π― Efficient Array-Backed Priority Queue Implementati... | β ββ | Start Challenge |
| 12 | π― Linked List Data Structure | β ββ | Start Challenge |
| 13 | π― Reverse Order Number Addition in Python | β ββ | Start Challenge |
| 14 | π― Partition Linked List Around Value | β ββ | Start Challenge |
| 15 | π― Deleting Middle Node in Python Linked List | β ββ | Start Challenge |
| 16 | π― Find Loop Start | β ββ | Start Challenge |
| 17 | π― Kth To Last Elem | β ββ | Start Challenge |
| 18 | π― Removing Duplicates in Linked Lists | β ββ | Start Challenge |
| 19 | π― Palindrome Detection in Linked Lists | β ββ | Start Challenge |
| 20 | π― Implementing Multiple Stacks Using Array | β ββ | Start Challenge |
| 21 | π― Sorting Stack Using Additional Stack | β ββ | Start Challenge |
| 22 | π― Set of Stacks | β ββ | Start Challenge |
| 23 | π― Implement O(1) Stack with Push, Pop, Min | β ββ | Start Challenge |
| 24 | π― Implementing Queue Using Linked List | β ββ | Start Challenge |
| 25 | π― Concise Introduction to Stacks | β ββ | Start Challenge |
| 26 | π― Queue From Stacks | β ββ | Start Challenge |
| 27 | π― Breadth-First Search Graph Traversal Algorithm | β ββ | Start Challenge |
| 28 | π― Graph Shortest Path | β ββ | Start Challenge |
| 29 | π― Depth-First Traversal of Binary Trees | β ββ | Start Challenge |
| 30 | π― Balanced Binary Tree in Python | β ββ | Start Challenge |
| 31 | π― Binary Tree Lowest Common Ancestor | β ββ | Start Challenge |
| 32 | π― Graph Data Structure Fundamentals | β ββ | Start Challenge |
| 33 | π― Trie Data Structure for String Storage | β ββ | Start Challenge |
| 34 | π― Bst Second Largest | β ββ | Start Challenge |
| 35 | π― Graph Build Order | β ββ | Start Challenge |
| 36 | π― Min Heap Binary Tree Introduction | β ββ | Start Challenge |
| 37 | π― Determine Binary Tree Height | β ββ | Start Challenge |
| 38 | π― Binary Search Tree In-Order Successor | β ββ | Start Challenge |
| 39 | π― Depth-First Search on Directed Graphs | β ββ | Start Challenge |
| 40 | π― Inverting Binary Tree Technique | β ββ | Start Challenge |
| 41 | π― Breadth-First Traversal Binary Tree | β ββ | Start Challenge |
| 42 | π― Minimal Height Binary Search Tree | β ββ | Start Challenge |
| 43 | π― Graph Shortest Path Unweighted | β ββ | Start Challenge |
| 44 | π― Graph Path Exists | β ββ | Start Challenge |
| 45 | π― Validating Binary Search Tree | β ββ | Start Challenge |
| 46 | π― Binary Search Tree Implementation in Python | β ββ | Start Challenge |
| 47 | π― Tree Level Lists | β ββ | Start Challenge |
| 48 | π― Rotated Array Search | β ββ | Start Challenge |
| 49 | π― Efficient Merge Sort Algorithm Implementation | β ββ | Start Challenge |
| 50 | π― Search Sorted Matrix | β ββ | Start Challenge |
| 51 | π― Efficient Quick Sort Algorithm | β ββ | Start Challenge |
| 52 | π― Sorting with Selection Algorithm | β ββ | Start Challenge |
| 53 | π― Merge Sorted Arrays into One | β ββ | Start Challenge |
| 54 | π― Missing Integer in Array | β ββ | Start Challenge |
| 55 | π― Anagram Sorting Array | β ββ | Start Challenge |
| 56 | π― Efficient Insertion Sort Algorithm | β ββ | Start Challenge |
| 57 | π― Radix Sort: Efficient Integer Sorting Algorithm | β ββ | Start Challenge |
| 58 | π― Fibonacci Sequence Programming Tutorial | β ββ | Start Challenge |
| 59 | π― Max Profit K | β ββ | Start Challenge |
| 60 | π― Longest Inc Subseq | β ββ | Start Challenge |
| 61 | π― Solving the Towers of Hanoi Problem | β ββ | Start Challenge |
| 62 | π― Generating All Permutations of Input String | β ββ | Start Challenge |
| 63 | π― N Pairs Parentheses | β ββ | Start Challenge |
| 64 | π― Coin Change Ways | β ββ | Start Challenge |
| 65 | π― Longest Common Subsequence | β ββ | Start Challenge |
| 66 | π― Exploring Step Climbing with Python | β ββ | Start Challenge |
| 67 | π― Knapsack Problem Optimization Techniques | β ββ | Start Challenge |
| 68 | π― Coin Change Min | β ββ | Start Challenge |
| 69 | π― Generating Power Sets in Python | β ββ | Start Challenge |
| 70 | π― Unbounded Knapsack Optimization Problem | β ββ | Start Challenge |
| 71 | π― Longest Substr K Distinct | β ββ | Start Challenge |
| 72 | π― Optimizing 2x2 Matrix Multiplication | β ββ | Start Challenge |
| 73 | π― Identifying Magic Indexes in Arrays | β ββ | Start Challenge |
| 74 | π― Python Challenge: Longest Common Substring | β ββ | Start Challenge |
| 75 | π― Optimal Coin Change Solution | β ββ | Start Challenge |
| 76 | π― Robot Grid Path Planning | β ββ | Start Challenge |
| 77 | π― Primality Test in Python | β ββ | Start Challenge |
| 78 | π― Integers Manipulation Python | β ββ | Start Challenge |
| 79 | π― Subtracting Integers Without Arithmetic Operators | β ββ | Start Challenge |
| 80 | π― Efficient List Operations in Python | β ββ | Start Challenge |
| 81 | π― Generate Prime Numbers | β ββ | Start Challenge |
| 82 | π― Determine Power of Two in Python | β ββ | Start Challenge |
| 83 | π― Bit Manipulation Operations in Python | β ββ | Start Challenge |
| 84 | π― Efficient Bit Manipulation for Odd-Even Swap | β ββ | Start Challenge |
| 85 | π― Maximizing Longest Sequence of Ones | β ββ | Start Challenge |
| 86 | π― Bits To Flip | β ββ | Start Challenge |
| 87 | π― Insert M Into N | β ββ | Start Challenge |
| 88 | π― Find Next Largest Smallest Binary Numbers | β ββ | Start Challenge |
| 89 | π― Binary Representation of Real Numbers | β ββ | Start Challenge |
| 90 | π― Implementing Line Drawing Algorithm | β ββ | Start Challenge |## More
- π [Python Programming Courses](https://github.com/labex-labs/awesome-programming-courses)
- π [Python Programming Projects](https://github.com/labex-labs/awesome-programming-projects)
- π [Python Free Tutorials](https://github.com/labex-labs/python-free-tutorials)