Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/labex-labs/quick-start-with-javascript
[Quick Start with JavaScript] This repository collects 6 of programming scenarios (labs and challenges) for Quick Start with JavaScript. This course is designed for beginners who want to learn JavaScript and programming fundamentals. We will try to build a dynamic personal finance tracker, which ...
https://github.com/labex-labs/quick-start-with-javascript
List: quick-start-with-javascript
awesome awesome-list challenges course education hands-on javascript labex labs programming
Last synced: about 2 months ago
JSON representation
[Quick Start with JavaScript] This repository collects 6 of programming scenarios (labs and challenges) for Quick Start with JavaScript. This course is designed for beginners who want to learn JavaScript and programming fundamentals. We will try to build a dynamic personal finance tracker, which ...
- Host: GitHub
- URL: https://github.com/labex-labs/quick-start-with-javascript
- Owner: labex-labs
- Created: 2024-05-22T09:03:36.000Z (8 months ago)
- Default Branch: master
- Last Pushed: 2024-08-01T01:28:19.000Z (5 months ago)
- Last Synced: 2024-08-02T04:23:19.812Z (5 months ago)
- Topics: awesome, awesome-list, challenges, course, education, hands-on, javascript, labex, labs, programming
- Homepage: https://labex.io/skilltrees/javascript
- Size: 5.86 KB
- Stars: 0
- Watchers: 2
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# Quick Start with JavaScript
[](https://labex.io/courses/quick-start-with-javascript)
[](https://labex.io/courses/quick-start-with-javascript)
This course is designed for beginners who want to learn JavaScript and programming fundamentals. We will try to build a dynamic personal finance tracker, which will help you to understand the basics of JavaScript.

## Environment
LabEx is an interactive, hands-on learning platform dedicated to coding and technology. It combines labs, AI assistance, and virtual machines to provide a no-video, practical learning experience.
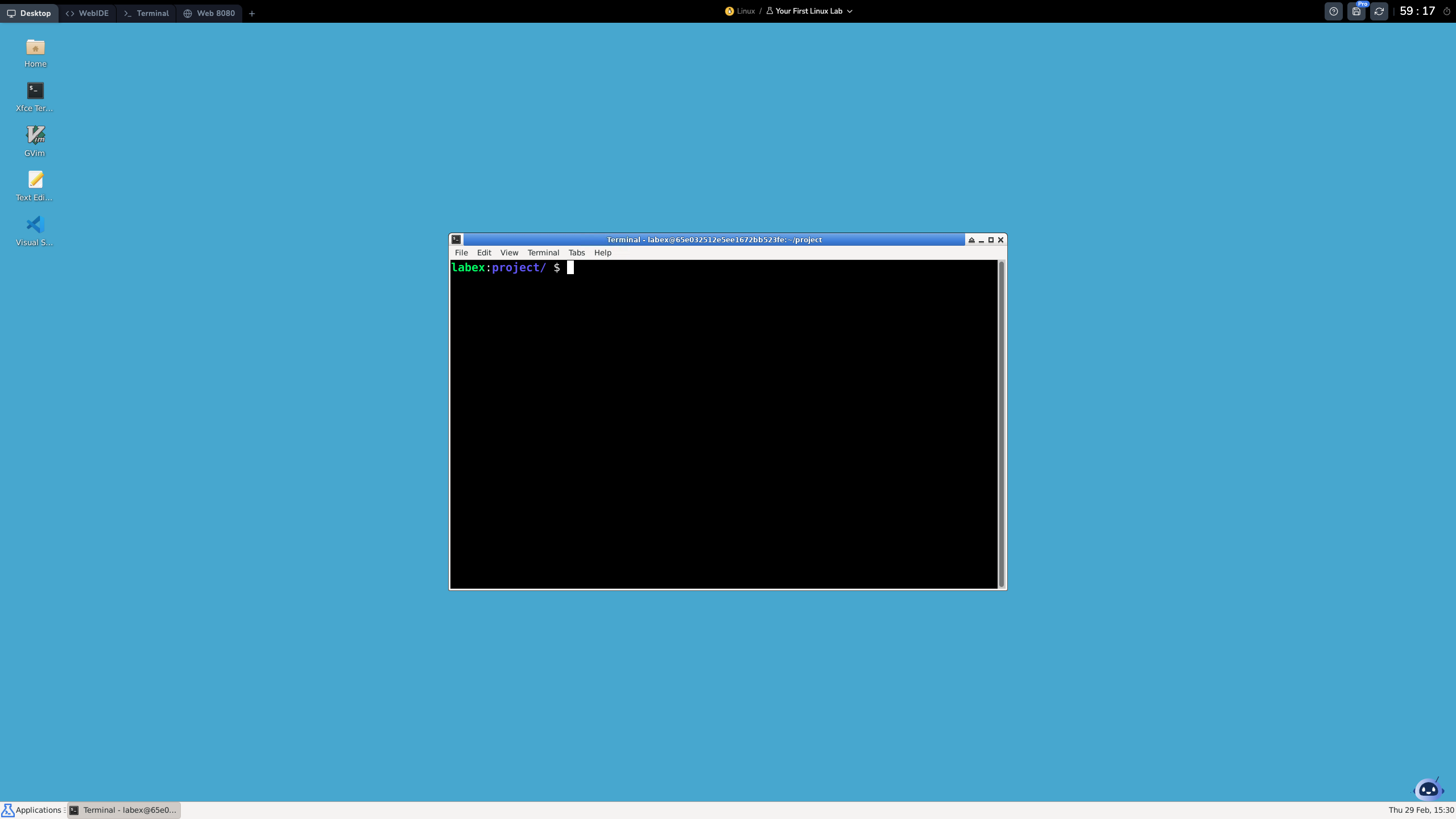
- A strict βLearn by Doingβ approach with exclusive hands-on labs and no videos.
- Interactive online environments within the browser, with automated step-by-step checks.
- A structured content organization with the Skill Tree based learning system.
- A growing learning resource of 30 Skill Trees and over 6,000 Labs.
- The AI learning assistant Labby, built on ChatGPT, providing a conversational learning experience.Learn more about [LabEx VM](https://support.labex.io/using-labex/virtual-machine).
## Exercises
| Index | Name | Difficulty | Practice |
|---------|-----------------------------------------------------|--------------|---------------------------------------------------------------------------------------------------------------------------------------|
| 01 | π Your First JavaScript Lab | β ββ | Start Lab |
| 02 | π Basic JavaScript and DOM | β ββ | Start Lab |
| 03 | π Arrays and Objects | β ββ | Start Lab |
| 04 | π Data Storage and Retrieval | β ββ | Start Lab |
| 05 | π Enhancing Personal Finance Tracker | β ββ | Start Lab |
| 06 | π Implementing the Summary | β ββ | Start Lab |
| 07 | π Value Is Null | β ββ | Start Lab |
| 08 | π Value Is Undefined | β ββ | Start Lab |
| 09 | π Value Is Number | β ββ | Start Lab |
| 10 | π Value Is String | β ββ | Start Lab |
| 11 | π Value Is Boolean | β ββ | Start Lab |
| 12 | π Type of Value | β ββ | Start Lab |
| 13 | π Round Number to Given Precision | β ββ | Start Lab |
| 14 | π Clamping Numbers in JavaScript | β ββ | Start Lab |
| 15 | π Number Is Even | β ββ | Start Lab |
| 16 | π Number Is Odd | β ββ | Start Lab |
| 17 | π Random Number in Range | β ββ | Start Lab |
| 18 | π Number to Currency String | β ββ | Start Lab |
| 19 | π Capitalize First Letter in JavaScript | β ββ | Start Lab |
| 20 | π Reverse String with JavaScript | β ββ | Start Lab |
| 21 | π Convert Strings to Camelcase with JavaScript | β ββ | Start Lab |
| 22 | π Truncating Strings in JavaScript | β ββ | Start Lab |
| 23 | π Padding Strings in JavaScript | β ββ | Start Lab |
| 24 | π String Manipulation with JavaScript | β ββ | Start Lab |
| 25 | π Head of Array | β ββ | Start Lab |
| 26 | π JavaScript Fundamentals Through Coding | β ββ | Start Lab |
| 27 | π Last Array Element | β ββ | Start Lab |
| 28 | π Array Without Last Element | β ββ | Start Lab |
| 29 | π Filtering Falsy Values in JavaScript Arrays | β ββ | Start Lab |
| 30 | π Random Element in Array | β ββ | Start Lab |
| 31 | π Check if Array Has Duplicates | β ββ | Start Lab |
| 32 | π Check if Array Includes All Values | β ββ | Start Lab |
| 33 | π Check if Array Includes Any Values | β ββ | Start Lab |
| 34 | π Filter Unique Array Values | β ββ | Start Lab |
| 35 | π Efficient Array Intersection in JavaScript | β ββ | Start Lab |
| 36 | π Beginner's Guide to JavaScript Fundamentals | β ββ | Start Lab |
| 37 | π Shallow Clone Object | β ββ | Start Lab |
| 38 | π Pick Object Keys | β ββ | Start Lab |
| 39 | π Omit Object Keys | β ββ | Start Lab |
| 40 | π Merging JavaScript Objects with Reduce and Concat | β ββ | Start Lab |
| 41 | π Convert Object to Pairs | β ββ | Start Lab |
| 42 | π Object From Pairs | β ββ | Start Lab |
| 43 | π Limiting Function Arguments in JavaScript | β ββ | Start Lab |
| 44 | π Function Composition in JavaScript | β ββ | Start Lab |
| 45 | π Composing Functions Efficiently in JavaScript | β ββ | Start Lab |
| 46 | π Optimizing JavaScript Functions with Memoization | β ββ | Start Lab |
| 47 | π Debouncing Promises in JavaScript | β ββ | Start Lab |
| 48 | π JavaScript Currying Techniques | β ββ | Start Lab |
| 49 | π Check for Leap Year | β ββ | Start Lab |
| 50 | π Explore JavaScript Programming Concepts | β ββ | Start Lab |
| 51 | π Calculate Date Difference in JavaScript | β ββ | Start Lab |
| 52 | π Add Date by Days in JavaScript | β ββ | Start Lab |
| 53 | π Create Human-Readable Time Formatting | β ββ | Start Lab |
| 54 | π Date Range Generator | β ββ | Start Lab |
| 55 | π Asynchronous Functions to Promises | β ββ | Start Lab |
| 56 | π Deep Clone Object | β ββ | Start Lab |
| 57 | π String Is Valid JSON | β ββ | Start Lab |
| 58 | π Stringify Circular JSON | β ββ | Start Lab |
| 59 | π Flatten JavaScript Object with Recursion | β ββ | Start Lab |
| 60 | π Deep Freeze Object | β ββ | Start Lab |## More
- π [JavaScript Programming Courses](https://github.com/labex-labs/awesome-programming-courses)
- π [JavaScript Programming Projects](https://github.com/labex-labs/awesome-programming-projects)
- π [JavaScript Free Tutorials](https://github.com/labex-labs/javascript-free-tutorials)