Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/labex-labs/rust-practice-labs
[Rust Practice Labs] This repository collects 231 of programming scenarios (labs and challenges) for Rust Practice Labs. This course contains lots of labs for Rust, each lab is a small Rust project with detailed guidance and solutions. You can practice your Rust skills by completing these labs, i...
https://github.com/labex-labs/rust-practice-labs
List: rust-practice-labs
awesome awesome-list challenges course education hands-on labex labs programming rust
Last synced: about 2 months ago
JSON representation
[Rust Practice Labs] This repository collects 231 of programming scenarios (labs and challenges) for Rust Practice Labs. This course contains lots of labs for Rust, each lab is a small Rust project with detailed guidance and solutions. You can practice your Rust skills by completing these labs, i...
- Host: GitHub
- URL: https://github.com/labex-labs/rust-practice-labs
- Owner: labex-labs
- Created: 2024-05-24T09:07:05.000Z (7 months ago)
- Default Branch: master
- Last Pushed: 2024-08-01T01:31:26.000Z (5 months ago)
- Last Synced: 2024-08-02T04:25:19.327Z (5 months ago)
- Topics: awesome, awesome-list, challenges, course, education, hands-on, labex, labs, programming, rust
- Homepage: https://labex.io/skilltrees/rust
- Size: 35.2 KB
- Stars: 0
- Watchers: 2
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# Rust Practice Labs
[](https://labex.io/courses/rust-practice-labs)
[](https://labex.io/courses/rust-practice-labs)
This course contains lots of labs for Rust, each lab is a small Rust project with detailed guidance and solutions. You can practice your Rust skills by completing these labs, improve your coding skills, and learn how to write clean and efficient code.

## Environment
LabEx is an interactive, hands-on learning platform dedicated to coding and technology. It combines labs, AI assistance, and virtual machines to provide a no-video, practical learning experience.
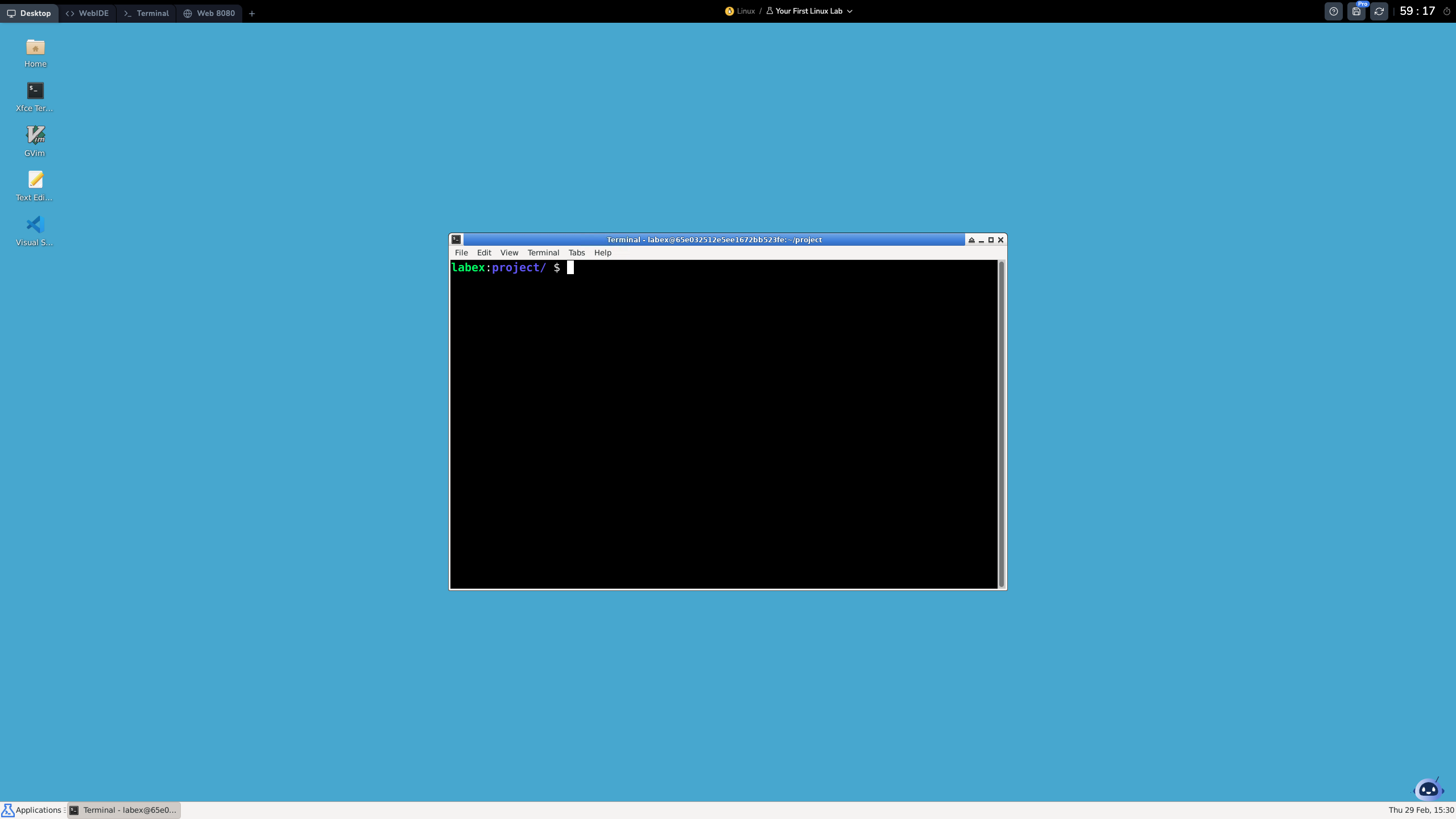
- A strict βLearn by Doingβ approach with exclusive hands-on labs and no videos.
- Interactive online environments within the browser, with automated step-by-step checks.
- A structured content organization with the Skill Tree based learning system.
- A growing learning resource of 30 Skill Trees and over 6,000 Labs.
- The AI learning assistant Labby, built on ChatGPT, providing a conversational learning experience.Learn more about [LabEx VM](https://support.labex.io/using-labex/virtual-machine).
## Exercises
| Index | Name | Difficulty | Practice |
|---------|---------------------------------------------------------|--------------|-----------------------------------------------------------------------------------------------------------------------------------------------|
| 001 | π Defining Modules to Control Scope and Privacy | β ββ | Start Lab |
| 002 | π Refutability in Rust Pattern Matching | β ββ | Start Lab |
| 003 | π Rust Metaprogramming with Macros | β ββ | Start Lab |
| 004 | π Disabling Rust Unused Code Warnings | β ββ | Start Lab |
| 005 | π Conditional Rust Function Compilation | β ββ | Start Lab |
| 006 | π Lifetime Concepts in Rust Programming | β ββ | Start Lab |
| 007 | π Domain Specific Languages | β ββ | Start Lab |
| 008 | π Variadic Interfaces in Rust Programming | β ββ | Start Lab |
| 009 | π Rust Namespacing with use Declaration | β ββ | Start Lab |
| 010 | π Rust Variable Binding Declaration | β ββ | Start Lab |
| 011 | π Rust Programming Fundamentals: Variable Bindings a... | β ββ | Start Lab |
| 012 | π Mutable Variable Bindings in Rust | β ββ | Start Lab |
| 013 | π Immutable Data Binding in Rust | β ββ | Start Lab |
| 014 | π Returning From Loops | β ββ | Start Lab |
| 015 | π An Example Program Using Structs | β ββ | Start Lab |
| 016 | π Controlling How Tests Are Run | β ββ | Start Lab |
| 017 | π Rust Borrow Checker Lifetime Elision | β ββ | Start Lab |
| 018 | π Rust Variable Bindings and Type Inference | β ββ | Start Lab |
| 019 | π Nesting and Labels | β ββ | Start Lab |
| 020 | π Rust FizzBuzz Loop Programming | β ββ | Start Lab |
| 021 | π Concise Control Flow with if Let | β ββ | Start Lab |
| 022 | π Rust Constants Exploration | β ββ | Start Lab |
| 023 | π Creating a Library | β ββ | Start Lab |
| 024 | π Macro Repetition in Rust | β ββ | Start Lab |
| 025 | π Exploring Rust Infinite Loops | β ββ | Start Lab |
| 026 | π Rust Literals and Type Specification | β ββ | Start Lab |
| 027 | π Rust Mutability Ownership Demonstration | β ββ | Start Lab |
| 028 | π Overloading Rust Macros with macro_rules! | β ββ | Start Lab |
| 029 | π Unrecoverable Errors with Panic | β ββ | Start Lab |
| 030 | π Destructuring and Dereferencing in Rust | β ββ | Start Lab |
| 031 | π Exploring Cargo Workspaces in Rust | β ββ | Start Lab |
| 032 | π Lifetime Management in Rust Structs | β ββ | Start Lab |
| 033 | π Lifetime Annotation in Rust Traits | β ββ | Start Lab |
| 034 | π Abort and Unwind | β ββ | Start Lab |
| 035 | π Cargo Attributes in Rust Development | β ββ | Start Lab |
| 036 | π Conditional Compilation with Rust's cfg Attribute | β ββ | Start Lab |
| 037 | π Scope and Shadowing | β ββ | Start Lab |
| 038 | π Destructuring Tuples in Rust | β ββ | Start Lab |
| 039 | π Reading a File | β ββ | Start Lab |
| 040 | π What Is Ownership? | β ββ | Start Lab |
| 041 | π The Slice Type | β ββ | Start Lab |
| 042 | π Printable Types in Rust's Standard Library | β ββ | Start Lab |
| 043 | π Waiting for Child Process Completion | β ββ | Start Lab |
| 044 | π Destructuring Rust Structs with Pattern Matching | β ββ | Start Lab |
| 045 | π Validating References with Lifetimes | β ββ | Start Lab |
| 046 | π Rust Enum Usage Examples | β ββ | Start Lab |
| 047 | π Rust Lifetime Annotations Borrow Checker | β ββ | Start Lab |
| 048 | π Rust Lifetime Coercion Exploration | β ββ | Start Lab |
| 049 | π Rust Primitive Types Exploration | β ββ | Start Lab |
| 050 | π Rust Lifetime and Trait Bounds | β ββ | Start Lab |
| 051 | π Rust Closures with Generic Constraints | β ββ | Start Lab |
| 052 | π Rust Multiple Bounds Exploration | β ββ | Start Lab |
| 053 | π Shared-State Concurrency in Rust | β ββ | Start Lab |
| 054 | π Defining and Instantiating Structs | β ββ | Start Lab |
| 055 | π Documenting Rust Projects with Markdown | β ββ | Start Lab |
| 056 | π Cargo Dependency Management in Rust | β ββ | Start Lab |
| 057 | π Publishing a Crate to Crates.io | β ββ | Start Lab |
| 058 | π Mutable Borrowing in Rust Book | β ββ | Start Lab |
| 059 | π Customizing Rust Struct Output with fmt::Display | β ββ | Start Lab |
| 060 | π Destructuring Arrays and Slices in Rust | β ββ | Start Lab |
| 061 | π As Output Parameters | β ββ | Start Lab |
| 062 | π Spawning Native Threads in Rust | β ββ | Start Lab |
| 063 | π Rust Enum Destructuring and Usage | β ββ | Start Lab |
| 064 | π Rust Comment Types and Documentation | β ββ | Start Lab |
| 065 | π Rust Loops and Ranges | β ββ | Start Lab |
| 066 | π The Ref Pattern | β ββ | Start Lab |
| 067 | π Aliases for Result | β ββ | Start Lab |
| 068 | π Exploring Rust's Functional Capabilities | β ββ | Start Lab |
| 069 | π Handling Errors with Early Returns in Rust | β ββ | Start Lab |
| 070 | π References and Borrowing | β ββ | Start Lab |
| 071 | π Rust Panic Handling Example | β ββ | Start Lab |
| 072 | π Rust Module Visibility Exploration | β ββ | Start Lab |
| 073 | π Multiple Error Types | β ββ | Start Lab |
| 074 | π Bringing Paths Into Scope with Use Keyword | β ββ | Start Lab |
| 075 | π Rust Aliasing: Enhancing Code Readability | β ββ | Start Lab |
| 076 | π Exploring Rust Closures and Environment Capture | β ββ | Start Lab |
| 077 | π Option & Unwrap | β ββ | Start Lab |
| 078 | π Executing Child Processes in Rust | β ββ | Start Lab |
| 079 | π Rust Aliasing Concept Exploration | β ββ | Start Lab |
| 080 | π Using Threads to Run Code Simultaneously | β ββ | Start Lab |
| 081 | π Rust Trait Inheritance and Composition | β ββ | Start Lab |
| 082 | π Chainable Option Handling with and_then() | β ββ | Start Lab |
| 083 | π Closures as Function Parameters | β ββ | Start Lab |
| 084 | π Exploring Rust's Result Type | β ββ | Start Lab |
| 085 | π The Use Declaration | β ββ | Start Lab |
| 086 | π Accepting Command Line Arguments | β ββ | Start Lab |
| 087 | π Rust Error Messages to Standard Error | β ββ | Start Lab |
| 088 | π Exploring Rust's Reference Counting Mechanism | β ββ | Start Lab |
| 089 | π Accessing Command Line Arguments in Rust | β ββ | Start Lab |
| 090 | π Rust Pattern Syntax Practice | β ββ | Start Lab |
| 091 | π Exploring Rust Struct Types and Applications | β ββ | Start Lab |
| 092 | π New Type Idiom | β ββ | Start Lab |
| 093 | π Packages and Crates | β ββ | Start Lab |
| 094 | π Cargo Documentation Generation and Testing | β ββ | Start Lab |
| 095 | π The Match Control Flow Construct | β ββ | Start Lab |
| 096 | π Storing Key-Value Pairs with Rust Hash Maps | β ββ | Start Lab |
| 097 | π Using Box for Heap Data | β ββ | Start Lab |
| 098 | π Rc, the Reference Counted Smart Pointer | β ββ | Start Lab |
| 099 | π Advanced Functions and Closures | β ββ | Start Lab |
| 100 | π Cloning Rust Structs with Clone Trait | β ββ | Start Lab |
| 101 | π Separating Modules Into Different Files | β ββ | Start Lab |
| 102 | π Exploring Rust Tuples and Transposing Matrices | β ββ | Start Lab |
| 103 | π Modular Rust File Organization | β ββ | Start Lab |
| 104 | π Rust Function Signatures with Lifetimes | β ββ | Start Lab |
| 105 | π Exploring Rust's Unsafe Operations | β ββ | Start Lab |
| 106 | π Using a Library | β ββ | Start Lab |
| 107 | π Treating Smart Pointers Like Regular References | β ββ | Start Lab |
| 108 | π Factoring Out Repetition with Rust Macros | β ββ | Start Lab |
| 109 | π Rust Method Syntax Practice | β ββ | Start Lab |
| 110 | π Rust Macros Designators Introduction | β ββ | Start Lab |
| 111 | π Binding and Destructuring in Rust | β ββ | Start Lab |
| 112 | π Alternate/Custom Key Types | β ββ | Start Lab |
| 113 | π To and From Strings | β ββ | Start Lab |
| 114 | π Rust Pattern Matching Exploration | β ββ | Start Lab |
| 115 | π Super and Self | β ββ | Start Lab |
| 116 | π Box, Stack and Heap | β ββ | Start Lab |
| 117 | π Rust Software Testing Fundamentals | β ββ | Start Lab |
| 118 | π Literals and Operators | β ββ | Start Lab |
| 119 | π Iterating Over Results | β ββ | Start Lab |
| 120 | π Formatted Print in Rust | β ββ | Start Lab |
| 121 | π Storing Lists of Values with Vectors | β ββ | Start Lab |
| 122 | π Rust Error Handling with Question Mark | β ββ | Start Lab |
| 123 | π Rust Library Functionality with Test-Driven Develo... | β ββ | Start Lab |
| 124 | π Efficient File Reading in Rust | β ββ | Start Lab |
| 125 | π Map for Result | β ββ | Start Lab |
| 126 | π Building a Single-Threaded Web Server | β ββ | Start Lab |
| 127 | π Rust Borrowing Ownership Fundamentals | β ββ | Start Lab |
| 128 | π Concise Rust Pattern Matching with Let-Else | β ββ | Start Lab |
| 129 | π Exploring Rust Traits for Customized Methods | β ββ | Start Lab |
| 130 | π Rust Type Inference Advanced Example | β ββ | Start Lab |
| 131 | π Concurrent Data Transfer with Rust Channels | β ββ | Start Lab |
| 132 | π Paths in Rust Module Tree | β ββ | Start Lab |
| 133 | π Shared Ownership with Rust Arc | β ββ | Start Lab |
| 134 | π Storing UTF-8 Encoded Text with Strings | β ββ | Start Lab |
| 135 | π Rust Book Lab: Unit and Integration Tests | β ββ | Start Lab |
| 136 | π Writing Rust Functions | β ββ | Start Lab |
| 137 | π Filesystem Operations in Rust | β ββ | Start Lab |
| 138 | π Concise Rust Option Iteration with While Let | β ββ | Start Lab |
| 139 | π Pulling Results Out of Options | β ββ | Start Lab |
| 140 | π Exploring Rust HashSet Operations | β ββ | Start Lab |
| 141 | π Command-Line Argument Parsing in Rust | β ββ | Start Lab |
| 142 | π Defining Generic Functions in Rust | β ββ | Start Lab |
| 143 | π Conditional Statements in Rust | β ββ | Start Lab |
| 144 | π Exploring Rust Generics Functionality | β ββ | Start Lab |
| 145 | π Rust Raw Identifiers Introduction | β ββ | Start Lab |
| 146 | π Phantom Type Parameters | β ββ | Start Lab |
| 147 | π Recoverable Errors with Result | β ββ | Start Lab |
| 148 | π Rust Method Usage Demonstration | β ββ | Start Lab |
| 149 | π Exploring Rust's Immutable Path Struct | β ββ | Start Lab |
| 150 | π To Panic or Not to Panic | β ββ | Start Lab |
| 151 | π How to Write Tests | β ββ | Start Lab |
| 152 | π Implement Generic Double Deallocation Trait | β ββ | Start Lab |
| 153 | π Exploring Rust's Drop Trait | β ββ | Start Lab |
| 154 | π Rust Operator Simplifies Error Handling | β ββ | Start Lab |
| 155 | π Diverging Functions in Rust | β ββ | Start Lab |
| 156 | π Visibility of Rust Struct Fields | β ββ | Start Lab |
| 157 | π Cleanup with Rust's Drop Trait | β ββ | Start Lab |
| 158 | π Reference Cycles Can Leak Memory | β ββ | Start Lab |
| 159 | π Implement fmt::Display for List in Rust | β ββ | Start Lab |
| 160 | π TryFrom and TryInto | β ββ | Start Lab |
| 161 | π Exploring Rust Iterator's Any Function | β ββ | Start Lab |
| 162 | π Rust Generics Type Constraints | β ββ | Start Lab |
| 163 | π Testcase: Empty Bounds | β ββ | Start Lab |
| 164 | π Exploring Rust's Impl Trait Functionality | β ββ | Start Lab |
| 165 | π Searching Through Iterators | β ββ | Start Lab |
| 166 | π Operator Overloading in Rust | β ββ | Start Lab |
| 167 | π Implementing an Object-Oriented Design Pattern | β ββ | Start Lab |
| 168 | π Foreign Function Interface | β ββ | Start Lab |
| 169 | π Simplifying Rust Option Handling with Map | β ββ | Start Lab |
| 170 | π Rust Integration Testing Fundamentals | β ββ | Start Lab |
| 171 | π Handling Errors with Box in Rust | β ββ | Start Lab |
| 172 | π From and Into | β ββ | Start Lab |
| 173 | π Rust Vectors: Resizable Array Essentials | β ββ | Start Lab |
| 174 | π Exploring Rust Closures and Capturing Behavior | β ββ | Start Lab |
| 175 | π Rust Formatting and Display Trait | β ββ | Start Lab |
| 176 | π Exploring Rust's Result Enum | β ββ | Start Lab |
| 177 | π Ownership and Moves | β ββ | Start Lab |
| 178 | π Rust Option Enum Handling Failure | β ββ | Start Lab |
| 179 | π Reading Files in Rust | β ββ | Start Lab |
| 180 | π Create File with Rust Standard Library | β ββ | Start Lab |
| 181 | π Child Process Interaction with Pipes | β ββ | Start Lab |
| 182 | π Rust Panic Handling and Memory Safety | β ββ | Start Lab |
| 183 | π Testcase: Unit Clarification | β ββ | Start Lab |
| 184 | π Closures: Anonymous Functions That Capture Their E... | β ββ | Start Lab |
| 185 | π Arrays and Slices | β ββ | Start Lab |
| 186 | π Unpacking Options and Defaults | β ββ | Start Lab |
| 187 | π Disambiguating Overlapping Traits | β ββ | Start Lab |
| 188 | π Parallel Data Processing in Rust | β ββ | Start Lab |
| 189 | π Generic Container Trait Implementation | β ββ | Start Lab |
| 190 | π Working with Environment Variables | β ββ | Start Lab |
| 191 | π Returning Traits with Dyn | β ββ | Start Lab |
| 192 | π Exploring Rust's if let Construct | β ββ | Start Lab |
| 193 | π Partial Move Destructuring in Rust | β ββ | Start Lab |
| 194 | π Rust Unit Testing Fundamentals | β ββ | Start Lab |
| 195 | π Using Rust Match Guards | β ββ | Start Lab |
| 196 | π Trait Objects for Heterogeneous Values | β ββ | Start Lab |
| 197 | π Processing a Series of Items with Iterators | β ββ | Start Lab |
| 198 | π Derive Traits in Rust Tuple Structs | β ββ | Start Lab |
| 199 | π Defining an Enum | β ββ | Start Lab |
| 200 | π Defining an Error Type | β ββ | Start Lab |
| 201 | π All the Places Patterns Can Be Used | β ββ | Start Lab |
| 202 | π Exploring Rust's Associated Types | β ββ | Start Lab |
| 203 | π Implementing Generic Types in Rust | β ββ | Start Lab |
| 204 | π Rust Casting: Explicit Type Conversion | β ββ | Start Lab |
| 205 | π Associated Functions & Methods | β ββ | Start Lab |
| 206 | π Rust RAII Resource Management | β ββ | Start Lab |
| 207 | π Characteristics of Object-Oriented Languages | β ββ | Start Lab |
| 208 | π Exploring Rust's Static Concept | β ββ | Start Lab |
| 209 | π Refactoring to Improve Modularity and Error Handli... | β ββ | Start Lab |
| 210 | π Implementing Iterators in Rust | β ββ | Start Lab |
| 211 | π Advanced Rust Traits Exploration | β ββ | Start Lab |
| 212 | π Other Uses of ? | β ββ | Start Lab |
| 213 | π Wrapping Errors with Custom Type | β ββ | Start Lab |
| 214 | π Exploring Rust String Concepts | β ββ | Start Lab |
| 215 | π As Input Parameters | β ββ | Start Lab |
| 216 | π Improving Our I/O Project | β ββ | Start Lab |
| 217 | π Traits: Defining Shared Behavior | β ββ | Start Lab |
| 218 | π Exploring Unsafe Rust Superpowers | β ββ | Start Lab |
| 219 | π Rust HashMap Data Storage Tutorial | β ββ | Start Lab |
| 220 | π Expressive Rust Generics with Where Clause | β ββ | Start Lab |
| 221 | π Unpacking Options with ? | β ββ | Start Lab |
| 222 | π Removing Duplication by Extracting a Function | β ββ | Start Lab |
| 223 | π Advanced Rust Types Practice | β ββ | Start Lab |
| 224 | π Rust Enum Concepts and Type Aliases | β ββ | Start Lab |
| 225 | π Rust Linked List Implementation | β ββ | Start Lab |
| 226 | π Rust Asynchronous Channels Communication | β ββ | Start Lab |
| 227 | π Exploring Rust Inline Assembly Usage | β ββ | Start Lab |
| 228 | π Rust Multithreaded Server Development | β ββ | Start Lab |
| 229 | π Rust Macros Exploration in LabEx | β ββ | Start Lab |
| 230 | π Graceful Shutdown and Cleanup | β ββ | Start Lab |
| 231 | π RefCell and the Interior Mutability Pattern | β ββ | Start Lab |## More
- π [Rust Programming Courses](https://github.com/labex-labs/awesome-programming-courses)
- π [Rust Programming Projects](https://github.com/labex-labs/awesome-programming-projects)
- π [Rust Free Tutorials](https://github.com/labex-labs/rust-free-tutorials)