Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/lbgm/phone-input
An Angular phone input component module
https://github.com/lbgm/phone-input
angular angular-material angular12 component library phone-input phone-number phoneinput
Last synced: about 2 months ago
JSON representation
An Angular phone input component module
- Host: GitHub
- URL: https://github.com/lbgm/phone-input
- Owner: lbgm
- License: gpl-3.0
- Created: 2022-10-10T11:06:43.000Z (about 2 years ago)
- Default Branch: main
- Last Pushed: 2023-11-04T17:17:38.000Z (about 1 year ago)
- Last Synced: 2024-10-30T05:58:22.414Z (about 2 months ago)
- Topics: angular, angular-material, angular12, component, library, phone-input, phone-number, phoneinput
- Language: TypeScript
- Homepage:
- Size: 527 KB
- Stars: 11
- Watchers: 2
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# PhoneInput
An Angular phone input component module.
This library was generated with [Angular CLI](https://github.com/angular/angular-cli) version 12.2.0.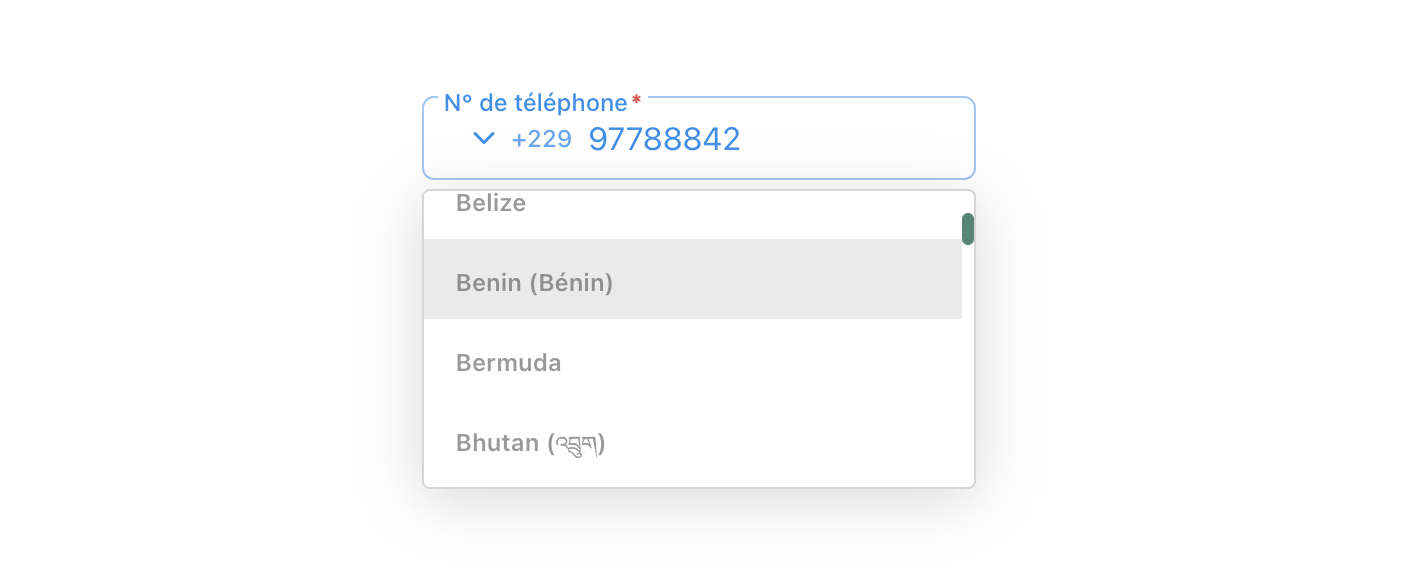
## Install
```sh
npm i @lbgm/phone-input
```## Props & Types
```ts
export type T_FormFieldControl = { [key: string]: AbstractControl; };export interface PhoneDATA {
country?: string;
dialCode?: string | number;
nationalNumber?: string | number;
number?: string | number;
isValid?: boolean;
}@Input() value?: string = ""; // like '22997000000'
@Input() label?: string = "";
@Input() hasError?: boolean = false;
@Input() hasSuccess?: boolean = false;
@Input() placeholder?: string = ""
@Input() name?: string = "lbgm-phone-input"
@Input() required?: boolean = false;
@Input() defaultCountry?: string = 'BJ';
@Input() arrow?: boolean = true; // to show or hide arrow
@Input() listHeight?: number = 150;
@Input() allowed?: string[] = ([]);@Input() group?: FormGroup;
@Input() controls?: T_FormFieldControl;
```- pass `value` on this format: `${dialCode}${nationalNumber}`
- `allowed` is an array of country iso2 (string).## Slots
```html
```
## Use
```ts
// app.module.tsimport { NgModule } from '@angular/core';
import { FormsModule, ReactiveFormsModule } from '@angular/forms';
import { BrowserModule } from '@angular/platform-browser';
import { PhoneInputModule } from '@lbgm/phone-input'; // hereimport { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent,
],
imports: [
BrowserModule,
FormsModule,
ReactiveFormsModule,
PhoneInputModule // here
],
providers: [
{
provide: Window,
useValue: window
}
],
bootstrap: [AppComponent]
})
export class AppModule { }
``````html
```
- phoneEvent is string
- country is string
- phoneData is type [PhoneDATA](#props--types)### Use with FormBuilder example
```ts
import { Component } from '@angular/core';
import { FormBuilder, Validators, FormGroup } from '@angular/forms';
import { PhoneDATA } from '@lbgm/phone-input';@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
title = 'phone-input';
form: FormGroup;
input?: string = "";
inputData?: PhoneDATA;
inputCountry: string = "";constructor(private fb: FormBuilder) {
this.form = fb.group({
'phone': [
'',
[
Validators.required,
Validators.minLength(8)
]
]
})
}onSubmit(): void {
this.form.markAllAsTouched();
}
}
```## Error on field
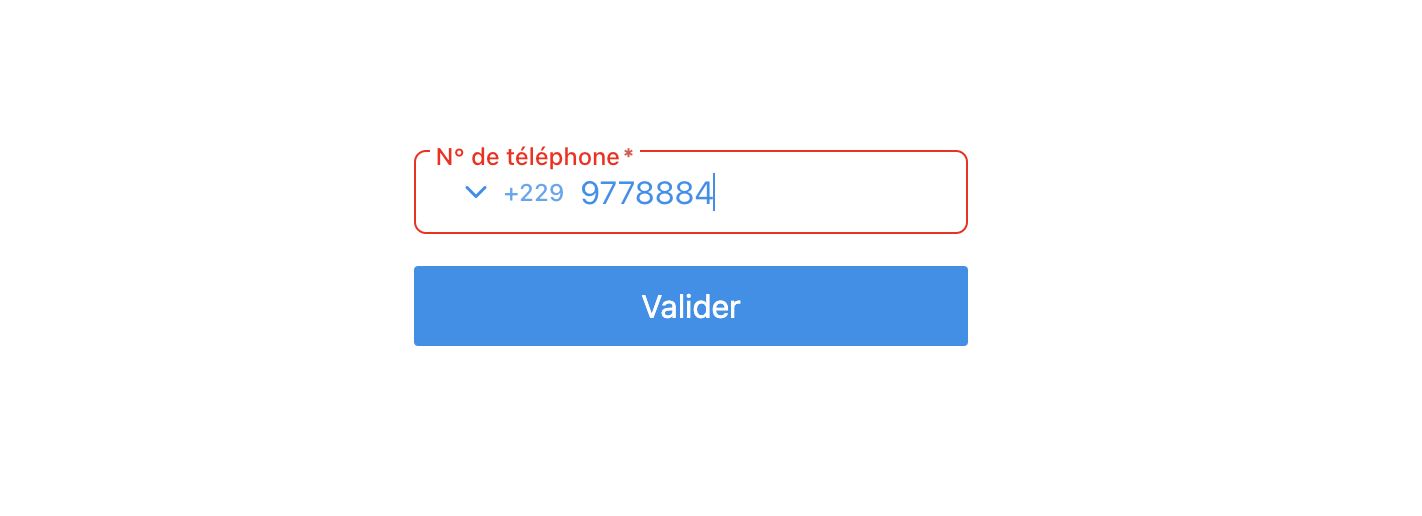