https://github.com/lbgm/phone-number-input
Phone Input library for Vue 3
https://github.com/lbgm/phone-number-input
input phone phone-number-input vue vue-phone-number-input vuejs-components vuejs-library vuejs3
Last synced: 3 months ago
JSON representation
Phone Input library for Vue 3
- Host: GitHub
- URL: https://github.com/lbgm/phone-number-input
- Owner: lbgm
- License: gpl-3.0
- Created: 2022-08-03T15:22:53.000Z (almost 3 years ago)
- Default Branch: main
- Last Pushed: 2024-02-26T17:05:15.000Z (over 1 year ago)
- Last Synced: 2025-04-19T21:56:08.741Z (3 months ago)
- Topics: input, phone, phone-number-input, vue, vue-phone-number-input, vuejs-components, vuejs-library, vuejs3
- Language: TypeScript
- Homepage:
- Size: 435 KB
- Stars: 31
- Watchers: 2
- Forks: 6
- Open Issues: 1
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# Phone number input
Simple Phone Number Input for VueJs
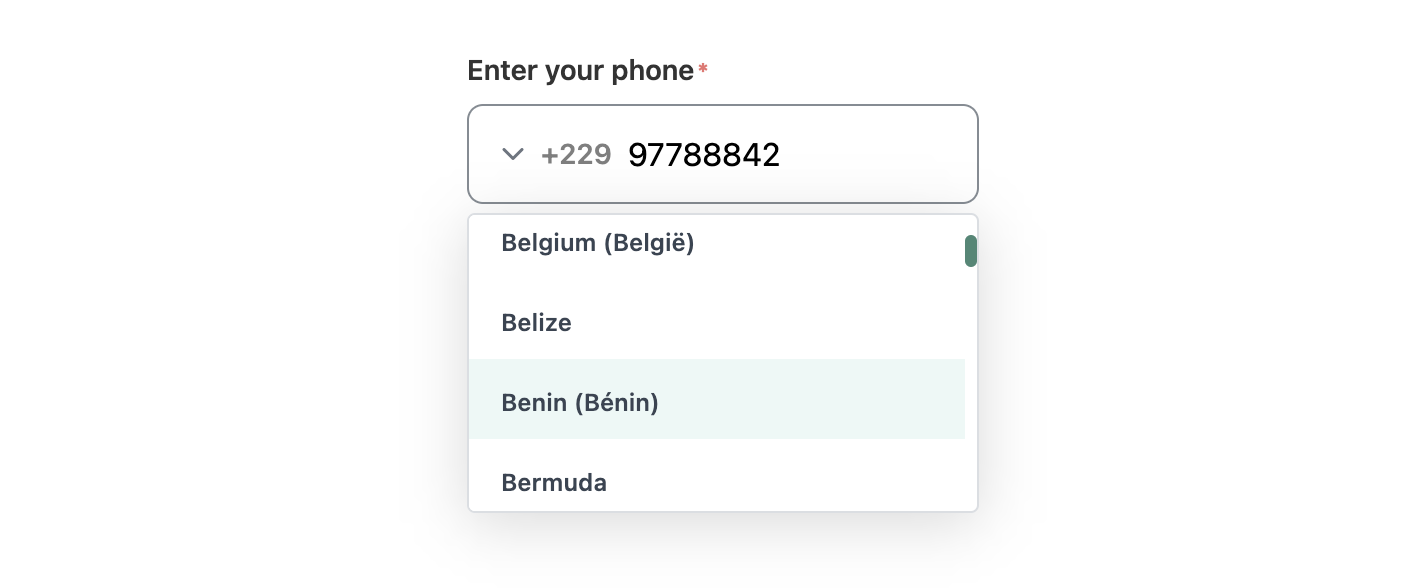
## install
```sh
npm i @lbgm/phone-number-input
```## Props & Types
```ts
interface PhoneDATA {
country?: string;
dialCode?: string | number;
nationalNumber?: string | number;
number?: string | number;
isValid?: boolean;
}interface Props {
value?: string;
label?: string;
hasError?: boolean;
hasSuccess?: boolean;
successMessage?: string;
errorMessage?: string;
placeholder?: string;
name?: string;
required?: boolean;
defaultCountry?: string;
arrow?: boolean;
listHeight?: number;
allowed?: string[];
}// default props values
{
value: "", // like '22997000000',
label: "",
hasError: false,
hasSuccess: false,
successMessage: "",
errorMessage: "",
placeholder: "",
name: "",
required: false,
defaultCountry: "BJ",
arrow: true, // show or hide arrow
listHeight: 150,
allowed: () => [],
}
```- pass `value` on this format: `${dialCode}${nationalNumber}`
- `allowed` is an array of country iso2 (string).## Slots
### arrow
```html
```use global slot to append content at the end of the component.
```html
Hello
```## Use
main.ts :
```js
import { PhoneInput } from '@lbgm/phone-number-input';// register as global component
app.component('PhoneInput', PhoneInput);
```App.vue :
```js
// import component style
import '@lbgm/phone-number-input/style';
```use component:
```html
```- `phone` is string
- `country` is string
- `phoneData` is type [PhoneDATA](#props--types)## Use it with Vee-validate
Sample wrapper code:
```html
```
```ts
import { useField } from 'vee-validate';
import { computed, onMounted, useAttrs, getCurrentInstance, type ComponentInternalInstance } from 'vue';
import { PhoneInput, type PhoneDATA } from '@lbgm/phone-number-input';type T_PhoneInput = typeof PhoneInput;
const that: ComponentInternalInstance | null = getCurrentInstance();
const attrs = useAttrs();
const emit = defineEmits(['inputData']);const {
value: inputValue,
errorMessage,
handleBlur,
handleChange,
meta,
} = useField(attrs.name, undefined, {
initialValue: attrs.value ? attrs.value : '',
validateOnValueUpdate: false,
});// compute error from vee-validate
const hasError = computed((): boolean => {
return errorMessage.value !== undefined;
});const validatePhone = (data: PhoneDATA) => {
handleChange(data.nationalNumber, false);
emit('inputData', data);
};onMounted(() => {
if ((that?.refs?.phoneInput as T_PhoneInput).phone) {
handleChange((that?.refs.phoneInput as T_PhoneInput).phone);
}
});```