Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/luluw8071/neural-network-from-scratch-using-numpy
A linear neural network from scratch using Numpy for training MNIST Dataset
https://github.com/luluw8071/neural-network-from-scratch-using-numpy
deep-neural-networks digit-recognition-mnist mnist-classification neural-network-from-scratch numpy python
Last synced: about 1 month ago
JSON representation
A linear neural network from scratch using Numpy for training MNIST Dataset
- Host: GitHub
- URL: https://github.com/luluw8071/neural-network-from-scratch-using-numpy
- Owner: LuluW8071
- License: mit
- Created: 2024-03-29T15:34:08.000Z (11 months ago)
- Default Branch: main
- Last Pushed: 2024-03-31T06:44:35.000Z (11 months ago)
- Last Synced: 2024-11-12T06:09:28.850Z (3 months ago)
- Topics: deep-neural-networks, digit-recognition-mnist, mnist-classification, neural-network-from-scratch, numpy, python
- Language: Python
- Homepage:
- Size: 12.7 KB
- Stars: 1
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# Neural-Network-From-Scratch-Using-Numpy
This repository contains code for building and training a neural network from scratch using Numpy, a Python library for numerical computing. The neural network is trained on the **MNIST dataset** for digit recognition.
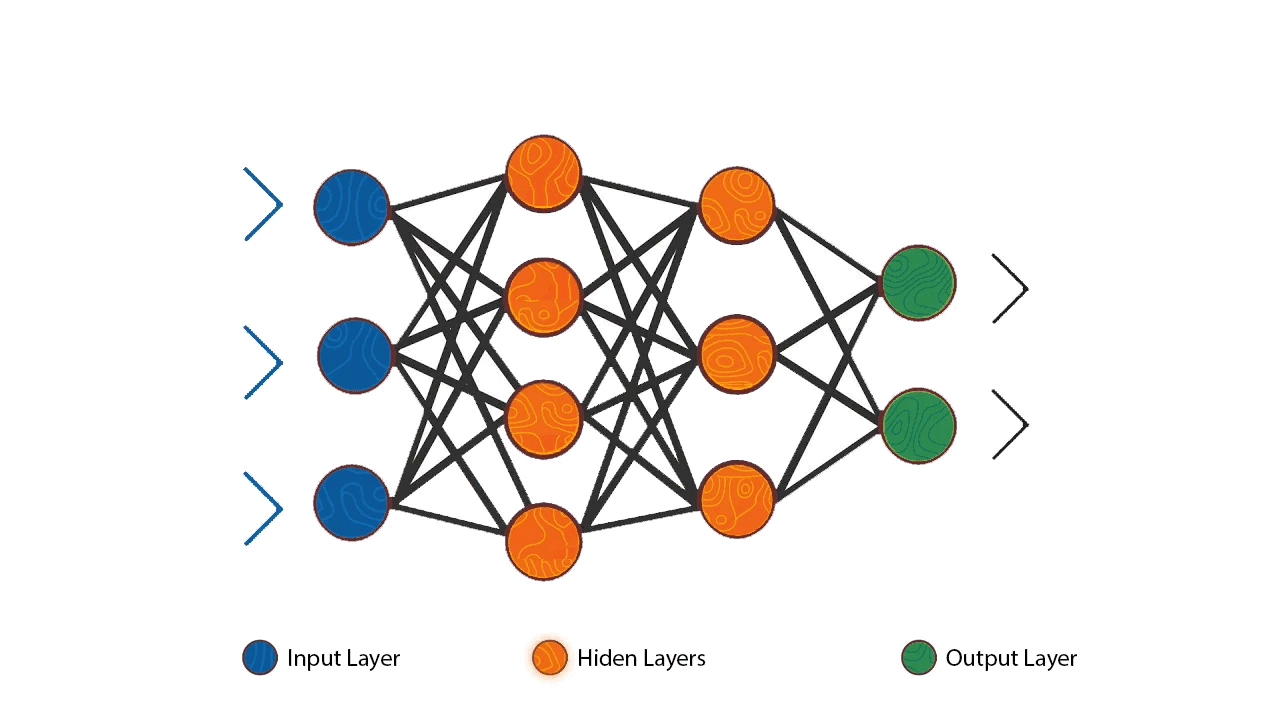
## Overview
In this project, we implement a simple neural network architecture with one hidden layer using the following steps:
| Step | Description |
|--------------------|---------------------------------------------------------------------------------------------------|
| **Data Loading** | Load the **MNIST dataset** containing images of handwritten digits which can be downloaded form [here](https://www.kaggle.com/competitions/digit-recognizer) |
| **Data Preprocessing** | Normalize the images to have pixel values between 0 and 1. **One-hot encode** labels for classification. |
| **Model Architecture** | Construct a neural network with an **input layer**, a **hidden layer** with **ReLU** activation, and an **output layer** with **softmax** activation. |
| **Training** | Train the neural network using **batch gradient descent** with **backpropagation**. Monitor training for loss and accuracy. |
| **Evaluation** | Evaluate the trained model on a separate test set to measure its performance. |
| **Prediction** | Use the trained model to make predictions on new unseen data. |## Usage
1. Clone the repository:
```bash
git clone https://github.com/LuluW8071/Neural-Network-From-Scratch-Using-Numpy.git
```2. Install the required dependencies:
```bash
pip install -r requirements.txt
```3. Run the training script:
```bash
python main.py --train_path "/path/to/train.csv" --test_path "/path/to/test.csv" --plot 1
```You can also adjust other parameters for train configs as needed. Here's a table summarizing the command-line arguments accepted by the script:
| Argument | Description |
|-------------------|-----------------------------------------------------------------------------------------------|
| `--train_path` | Path to the CSV file containing the training data. |
| `--test_path` | Path to the CSV file containing the test data. |
| `--learning_rate` | Learning rate to be used for training the model. Default is `0.001`. |
| `--epochs` | Number of epochs for training the model. Default is `150`. |
| `--batch_size` | Batch size to be used during training. Default is `64`. |
| `--plot` | Whether to plot the loss and accuracy curves after training. Set to `1` to plot, `0` otherwise. |
| `--save_path` | Directory to save the submission file. Default is `current_directory`. |#### Sample Demo Run Output
```bash
(env) PS D:\Neural-Network-From-Scratch-Using-Numpy> py .\main.py --epochs 150 --plot 1 --train_path ".\train.csv" --test_path ".\test.csv"
Loaded train.csv and test.csv
100%|███████████████████████████████████████████████████████| 150/150 [07:27<00:00, 2.99s/it, accuracy=1, loss=1.3e-5]
Saved inference.csv
```4. After training, the model will make predictions on the `test.csv` set and save the results in a `inference.csv` file.
### Accuracy and Loss Curves
Also if you want the **CNN(Convolutional Neural Network)** implementation, you can find the notebook [here](https://www.kaggle.com/code/luluw8071/mnist-trained-on-tinyvgg-model-with-pytorch?scriptVersionId=168950513).
## License
This project is licensed under the MIT License - see the [LICENSE](LICENSE) file for details.
---
Feel free to report any issues you encounter.
Don't forget to star the repo :)