Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/luolingchun/flask-openapi3
Generate REST API and OpenAPI documentation for your Flask project.
https://github.com/luolingchun/flask-openapi3
flask flask-openapi3 openapi openapi3 pydantic python python3 rapidoc redoc rest swagger swagger-ui
Last synced: 3 days ago
JSON representation
Generate REST API and OpenAPI documentation for your Flask project.
- Host: GitHub
- URL: https://github.com/luolingchun/flask-openapi3
- Owner: luolingchun
- License: mit
- Created: 2021-04-28T02:39:57.000Z (over 3 years ago)
- Default Branch: master
- Last Pushed: 2024-08-31T09:00:46.000Z (about 1 month ago)
- Last Synced: 2024-09-21T12:04:54.078Z (12 days ago)
- Topics: flask, flask-openapi3, openapi, openapi3, pydantic, python, python3, rapidoc, redoc, rest, swagger, swagger-ui
- Language: Python
- Homepage: https://luolingchun.github.io/flask-openapi3/
- Size: 8.63 MB
- Stars: 186
- Watchers: 7
- Forks: 28
- Open Issues: 15
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- Contributing: CONTRIBUTING.md
- License: LICENSE.rst
- Code of conduct: CODE_OF_CONDUCT.md
Awesome Lists containing this project
- jimsghstars - luolingchun/flask-openapi3 - Generate REST API and OpenAPI documentation for your Flask project. (Python)
README
Generate REST API and OpenAPI documentation for your Flask project.
**Flask OpenAPI3** is a web API framework based on **Flask**. It uses **Pydantic** to verify data and automatic
generation of interaction documentation.The key features are:
- **Easy to code:** Easy to use and easy to learn
- **Standard document specification:** Based on [OpenAPI Specification](https://spec.openapis.org/oas/v3.1.0)
- **Interactive OpenAPI documentation:** [Swagger](https://github.com/swagger-api/swagger-ui), [Redoc](https://github.com/Redocly/redoc), [RapiDoc](https://github.com/rapi-doc/RapiDoc), [RapiPdf](https://mrin9.github.io/RapiPdf/), [Scalar](https://github.com/scalar/scalar), [Elements](https://github.com/stoplightio/elements)
- **Data validation:** Fast data verification based on [Pydantic](https://github.com/pydantic/pydantic)
## Requirements
Python 3.8+
flask-openapi3 is dependent on the following libraries:
- [Flask](https://github.com/pallets/flask) for the web app.
- [Pydantic](https://github.com/pydantic/pydantic) for the data validation.## Installation
```bash
pip install -U flask-openapi3[swagger]
```or
```bash
conda install -c conda-forge flask-openapi3[swagger]
```Optional dependencies
- [python-email-validator](https://github.com/JoshData/python-email-validator) supports email verification.
- [python-dotenv](https://github.com/theskumar/python-dotenv#readme) enables support
for [Environment Variables From dotenv](https://flask.palletsprojects.com/en/latest/cli/#dotenv) when running `flask`
commands.
- [pyyaml](https://github.com/yaml/pyyaml) is used to output the OpenAPI document in yaml format.
- [asgiref](https://github.com/django/asgiref) allows views to be defined with `async def` and use `await`.
- [flask-openapi3-plugins](https://github.com/luolingchun/flask-openapi3-plugins) Provide OpenAPI UI for flask-openapi3.To install these dependencies with flask-openapi3:
```bash
pip install flask-openapi3[yaml]
# or
pip install flask-openapi3[async]
# or
pip install flask-openapi3[dotenv]
# or
pip install flask-openapi3[email]
# or all
pip install flask-openapi3[yaml,async,dotenv,email]
# or manually
pip install pyyaml asgiref python-dotenv email-validator
# OpenAPI UI plugins
pip install -U flask-openapi3[swagger,redoc,rapidoc,rapipdf,scalar,elements]
```## A Simple Example
Here's a simple example, further go to the [Example](https://luolingchun.github.io/flask-openapi3/latest/Example/).
```python
from pydantic import BaseModelfrom flask_openapi3 import Info, Tag
from flask_openapi3 import OpenAPIinfo = Info(title="book API", version="1.0.0")
app = OpenAPI(__name__, info=info)book_tag = Tag(name="book", description="Some Book")
class BookQuery(BaseModel):
age: int
author: str@app.get("/book", summary="get books", tags=[book_tag])
def get_book(query: BookQuery):
"""
to get all books
"""
return {
"code": 0,
"message": "ok",
"data": [
{"bid": 1, "age": query.age, "author": query.author},
{"bid": 2, "age": query.age, "author": query.author}
]
}if __name__ == "__main__":
app.run(debug=True)
```Class-based API View Example
```python
from typing import Optionalfrom pydantic import BaseModel, Field
from flask_openapi3 import OpenAPI, Tag, Info, APIView
info = Info(title='book API', version='1.0.0')
app = OpenAPI(__name__, info=info)api_view = APIView(url_prefix="/api/v1", view_tags=[Tag(name="book")])
class BookPath(BaseModel):
id: int = Field(..., description="book ID")class BookQuery(BaseModel):
age: Optional[int] = Field(None, description='Age')class BookBody(BaseModel):
age: Optional[int] = Field(..., ge=2, le=4, description='Age')
author: str = Field(None, min_length=2, max_length=4, description='Author')@api_view.route("/book")
class BookListAPIView:
a = 1@api_view.doc(summary="get book list")
def get(self, query: BookQuery):
print(self.a)
return query.model_dump_json()@api_view.doc(summary="create book")
def post(self, body: BookBody):
"""description for a created book"""
return body.model_dump_json()@api_view.route("/book/")
class BookAPIView:
@api_view.doc(summary="get book")
def get(self, path: BookPath):
print(path)
return "get"@api_view.doc(summary="update book")
def put(self, path: BookPath):
print(path)
return "put"@api_view.doc(summary="delete book", deprecated=True)
def delete(self, path: BookPath):
print(path)
return "delete"app.register_api_view(api_view)
if __name__ == "__main__":
app.run(debug=True)
```## API Document
Run the [simple example](https://github.com/luolingchun/flask-openapi3/blob/master/examples/simple_demo.py), and go to http://127.0.0.1:5000/openapi.
> OpenAPI UI plugins are optional dependencies that require manual installation.
>
> `pip install -U flask-openapi3[swagger,redoc,rapidoc,rapipdf,scalar,elements]`
>
> More optional ui templates goto the document
> about [UI_Templates](https://luolingchun.github.io/flask-openapi3/latest/Usage/UI_Templates/).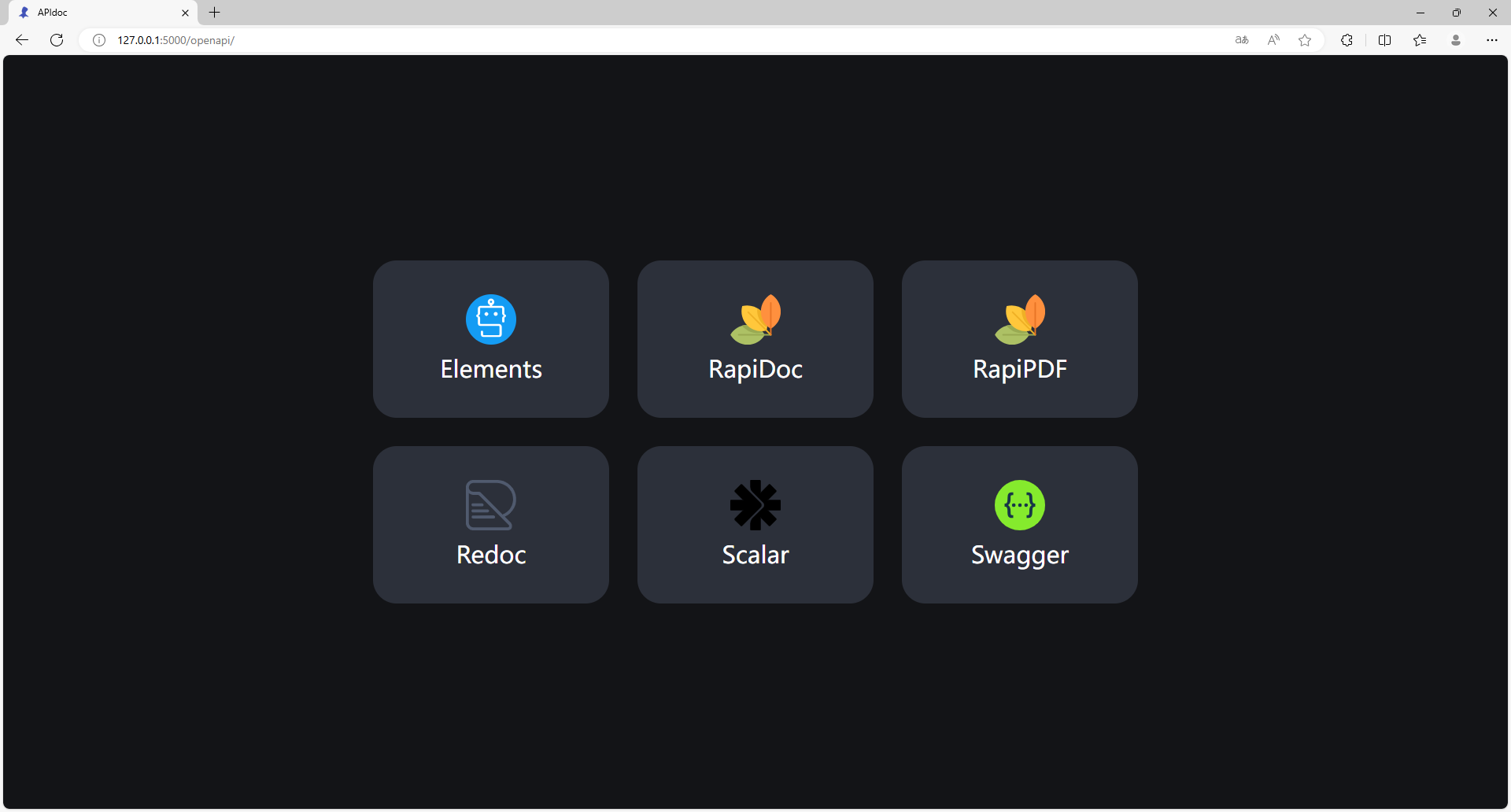