https://github.com/mailslurp/mailslurp-client-php
MailSlurp PHP Client
https://github.com/mailslurp/mailslurp-client-php
Last synced: 3 months ago
JSON representation
MailSlurp PHP Client
- Host: GitHub
- URL: https://github.com/mailslurp/mailslurp-client-php
- Owner: mailslurp
- License: mit
- Created: 2019-08-27T09:31:36.000Z (almost 6 years ago)
- Default Branch: master
- Last Pushed: 2025-03-04T21:41:00.000Z (4 months ago)
- Last Synced: 2025-04-13T03:08:00.947Z (3 months ago)
- Language: PHP
- Homepage: https://www.mailslurp.com
- Size: 25.1 MB
- Stars: 6
- Watchers: 0
- Forks: 7
- Open Issues: 1
-
Metadata Files:
- Readme: README.md
- License: LICENSE
- Security: SECURITY.md
- Support: SUPPORT.md
Awesome Lists containing this project
README
# PHP Email and SMS API - Documentation
Create and manage email addresses and phone numbers in PHP. Send and receive emails, attachments, and SMS in code and tests. Here is a quick example:
```php
// configure mailslurp/mailslurp-client-php library
$config = MailSlurp\Configuration::getDefaultConfiguration()
->setApiKey('x-api-key', getenv("API_KEY"));
$inboxController = new MailSlurp\Apis\InboxControllerApi(null, $config);
// create an inbox
$inbox = $inboxController->createInboxWithDefaults();
// send an email
$sendOptions = new MailSlurp\Models\SendEmailOptions();
$sendOptions->setTo([$inbox->getEmailAddress()]);
$sendOptions->setSubject("Test");
$sendOptions->setBody("Hello");
$inboxController->sendEmail($inbox->getId(), $sendOptions);
// receive the email
$waitForController = new MailSlurp\Apis\WaitForControllerApi(null, $config);
$email = $waitForController->waitForLatestEmail($inbox->getId(), 120000, true);
$this->assertNotNull($email->getBody());
```## Video tutorial
[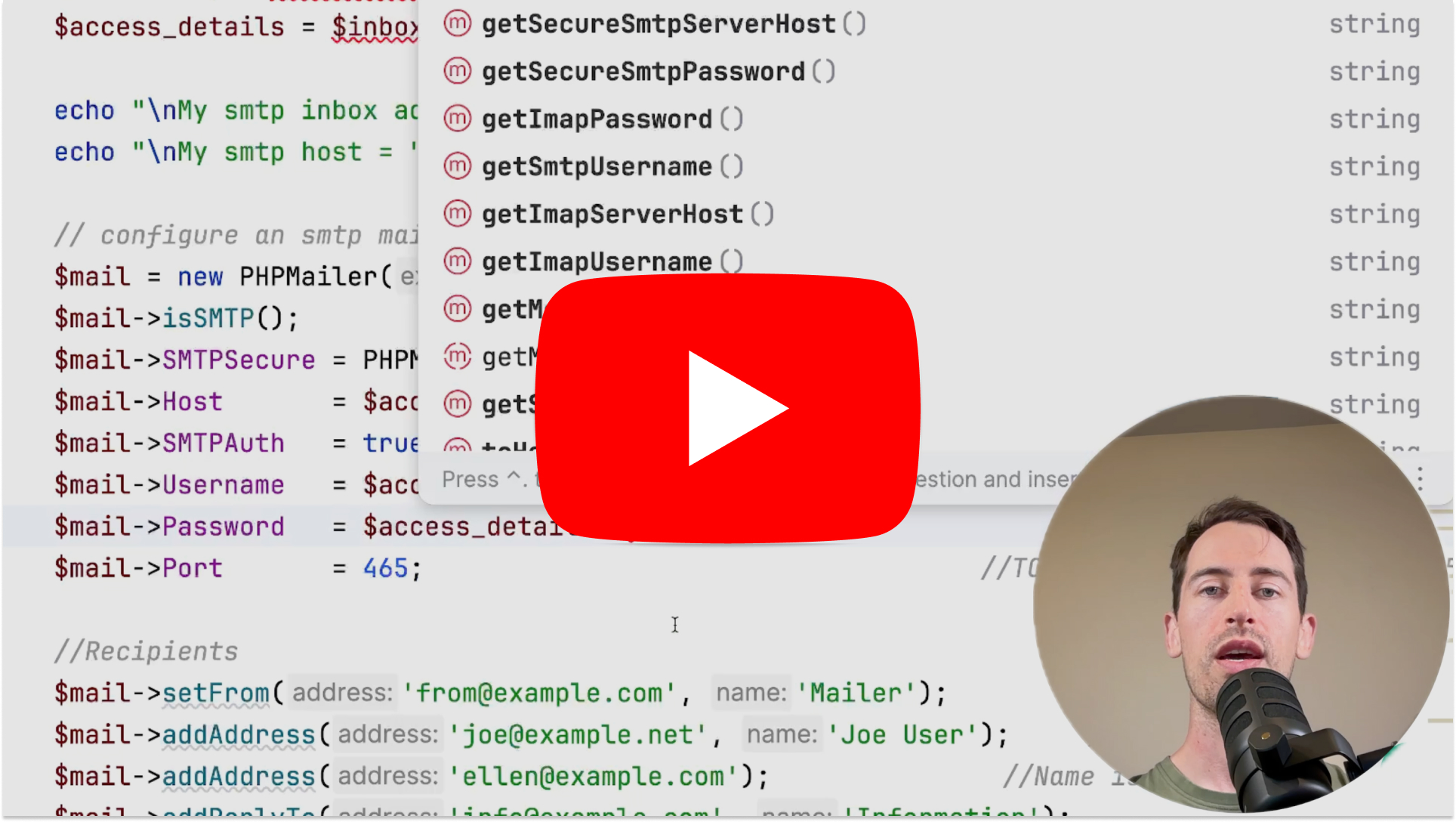](https://youtu.be/-gVB7WvHvfw)
## Quick links
- [API documentation](https://docs.mailslurp.com/api/)
- [Download PHP Plugin](https://www.github.com/mailslurp/mailslurp-client-php/)
- [GitHub Repository](https://www.github.com/mailslurp/mailslurp-client-php/)
- [Official PHP Library](https://packagist.org/packages/mailslurp/mailslurp-client-php)
- [Full method documentation](https://php.mailslurp.com/)## Examples
- [Laravel Mailable/Notification setup](https://www.mailslurp.com/guides/send-email-laravel-mailable-notifications/)
- [PHP Codeception Selenium Example](https://www.mailslurp.com/examples/php-test-email-api-codeception/)
- [PHPUnit Example](https://www.mailslurp.com/examples/phpunit-email-testing/)
- [PHP send email with SMTP](https://www.mailslurp.com/smtp/php-send-email-smtp/)## Get started
MailSlurp is an email API that lets you create email addresses on demand then send and receive emails in code and tests. **No MailServer is required**.
This section describes how to get up and running with the PHP client. To use another language or the REST API see the [developer page](https://www.mailslurp.com/developers/).
See the examples page for [code examples and use with common frameworks](https://www.mailslurp.com/examples/).
See the method documentation for a [list of all functions](https://php.mailslurp.com/).
## Get an API Key
You need a free MailSlurp account to use the service. [Sign up for a free account](https://app.mailslurp.com/sign-up/) first.
Once signed up [login your dashboard](https://app.mailslurp.com/login/). Find your API Key and copy the code. You'll need this to configure the MailSlurp client in PHP.
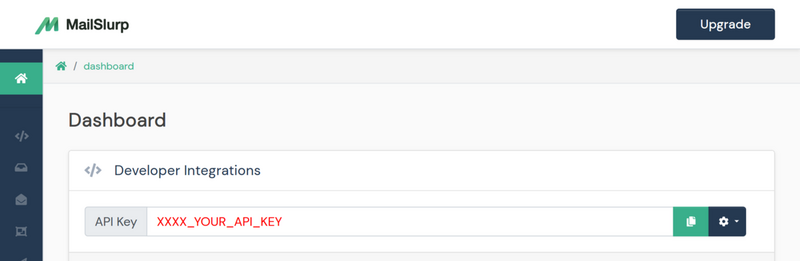
Copy your API Key from the [MailSlurp dashboard](https://app.mailslurp.com).
## PHP Setup
The client is tested for PHP 7 and requires the following non-default PHP extensions to be installed:
```bash
php-ext-curl
php-xml
php-mbstring
```Typically, these come with most PHP installations. On Linux they can also be installed like so:
`sudo apt-get install php-ext-curl php-mbstring php-xml`
## Add PHP Library
There are several ways to install MailSlurp.
### a) Composer dependency
If you use the [composer package manager](https://getcomposer.org) you can run:
```bash
composer require mailslurp/mailslurp-client-php
```Or add it to your `composer.json` file:
```json
{
"repositories": [
{
"type": "vcs",
"url": "https://github.com/mailslurp/mailslurp-client-php.git"
}
],
"require": {
"mailslurp/mailslurp-client-php": "*@dev"
}
}
```Then include the library with the composer autoload convention:
```php
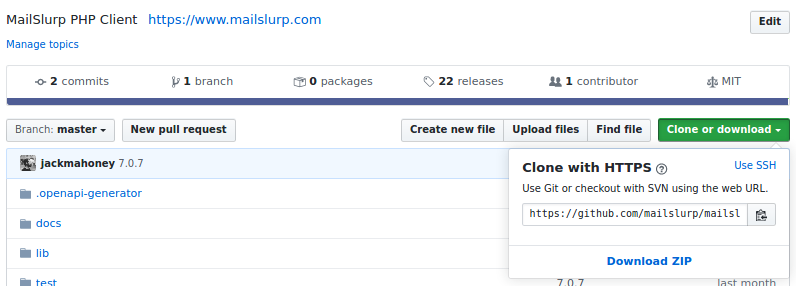
Download the MailSlurp PHP email libraryMailSlurp dashboard.
Then include the files to have access to MailSlurp in your code:
```php
setApiKey('x-api-key', getenv("API_KEY"));
}
```### Create a controller
```php
$inboxController = new MailSlurp\Apis\InboxControllerApi(null, $this->getConfig());
```## Basic usage
A common use case is to create a new email address, send it an email and then receive the contents:
### Create an email address
To create an email address use the inbox controller to create a new inbox. Inboxes have real email addresses and can send and receive emails and attachments.
```php
$options = new \MailSlurp\Models\CreateInboxDto();
$options->setName("Test inbox");
$options->setPrefix("test");
$inbox = $inboxController->createInboxWithOptions($options);
```### Send an email
Here is a simple example of sending an email:```php
$send_options = new MailSlurp\Models\SendEmailOptions();
$send_options->setTo([$inbox->getEmailAddress()]);
$send_options->setSubject("Test");
$send_options->setBody("Confirmation code = abc123");
$inbox_controller->sendEmail($inbox_2->getId(), $send_options);
```### Read an email
You can use the WaitFor controller methods to wait for an expected email to arrive in an inbox:```php
$wait_for_controller = new MailSlurp\Apis\WaitForControllerApi(null, $this->getConfig());
```Then call it like so:
```php
$timeout_ms = 30000;
$unread_only = true;
$email = $wait_for_controller->waitForLatestEmail($inbox->getId(), $timeout_ms, $unread_only);
```### Fetch emails
You can get emails from an inbox using the inbox controller:You can also fetch in paginated form:
### Get an email
Get an email directly by ID using the email controller:```php
s
$emails = $inbox_controller->getEmails($inbox->getId());
```### SMTP access (PHPMailer, mail function etc.)
SMTP inboxes provide IMAP and SMTP access credentials via the `getImapSmtpAccess` function. Use these to configure PHPMailer, PearMailer or other SMTP sending clients. First create an `SMTP_INBOX`:```php
$options = new \MailSlurp\Models\CreateInboxDto();
$options->setInboxType("SMTP_INBOX");
$inbox_smtp = $inboxController->createInboxWithOptions($options);
```Then use the access settings to configure PHPMailer:
```php
$access_details = $inboxController->getImapSmtpAccess($inbox_smtp->getId());
$mail = new PHPMailer\PHPMailer\PHPMailer(true);
// set mail server settings using the inbox access details
$mail->isSMTP();
$mail->Host = $access_details->getSecureSmtpServerHost();
$mail->SMTPAuth = true;
$mail->Username = $access_details->getSecureSmtpUsername();
$mail->Password = $access_details->getSecureSmtpPassword();
$mail->SMTPSecure = PHPMailer\PHPMailer\PHPMailer::ENCRYPTION_STARTTLS;
$mail->Port = $access_details->getSecureSmtpServerPort();
```### List inboxes
Inbox lists are paginated.
```php
config);$pageInboxes = $inboxController->getAllInboxes($favourite = null, $page = 0, $size = 20);
// assert pagination properties
$this->assertEquals(0, $pageInboxes->getNumber());
$this->assertEquals(20, $pageInboxes->getSize());
$this->assertGreaterThan(0, $pageInboxes->getTotalElements());// access inboxes via content
foreach ($pageInboxes->getContent() as $inbox) {
$this->assertNotNull($inbox->getId());
}
}
```### Send an email with more options
To send an email first create an inbox. Then use the `sendEmail` method on the `InboxController` and pass it the sender inbox's ID and email options.
```php
config);
$inbox = $inboxController->createInbox();// send options
$sendOptions = new MailSlurp\Models\SendEmailOptions();
$sendOptions->setTo([$inbox->getEmailAddress()]);
$sendOptions->setSubject("Welcome");
$sendOptions->setIsHtml(true);
// (you can use normal strings too)
$sendOptions->setBody(<<
MailSlurp supports HTML