https://github.com/managedcode/orleans.graph
Managing and validating grain call transitions in Microsoft Orleans applications. It allows you to define allowed communication patterns between grains
https://github.com/managedcode/orleans.graph
csharp graph orleans
Last synced: 14 days ago
JSON representation
Managing and validating grain call transitions in Microsoft Orleans applications. It allows you to define allowed communication patterns between grains
- Host: GitHub
- URL: https://github.com/managedcode/orleans.graph
- Owner: managedcode
- License: mit
- Created: 2024-11-10T08:57:50.000Z (6 months ago)
- Default Branch: main
- Last Pushed: 2024-11-20T13:57:11.000Z (5 months ago)
- Last Synced: 2025-04-05T16:37:57.387Z (21 days ago)
- Topics: csharp, graph, orleans
- Language: C#
- Homepage:
- Size: 63.5 KB
- Stars: 4
- Watchers: 1
- Forks: 0
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
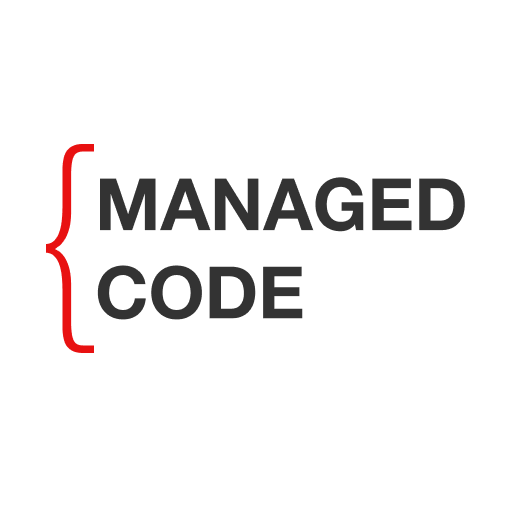
# Orleans Graph
[](https://www.nuget.org/packages/ManagedCode.Orleans.Graph)
[](https://github.com/managedcode/Orleans.Graph/actions/workflows/dotnet.yml)
[](https://github.com/managedcode/Orleans.Graph/actions/workflows/codeql-analysis.yml)A library for managing and validating grain call transitions in Microsoft Orleans applications. It allows you to define allowed communication patterns between grains and enforce them at runtime.
## Features
- Define allowed grain-to-grain communication patterns
- Validate grain call transitions at runtime
- Detect and prevent circular dependencies
- Support for method-level granularity
- Reentrancy control
- Client-to-grain call validation## Installation
```sh
dotnet add package ManagedCode.Orleans.Graph
```## Usage
### Configure Silo
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AddGrainTransition()
.Method(a => a.MethodA1(), b => b.MethodB1())
.And()
.AllowClientCallGrain();
});
```### Configure Client
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AddGrainTransition()
.AllMethods()
.And()
.AllowClientCallGrain();
});
```## Example
Here are more examples of how to configure the graph in your Orleans application:### Example 1: Simple Grain-to-Grain Transition
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AddGrainTransition()
.AllMethods();
});
```### Example 2: Method-Level Transition
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AddGrainTransition()
.Method(a => a.MethodA1(), b => b.MethodB1());
});
```### Example 3: Allowing Reentrancy
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AddGrainTransition()
.WithReentrancy()
.AllMethods();
});
```### Example 4: Multiple Transitions
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AddGrainTransition()
.AllMethods()
.And()
.AddGrainTransition()
.AllMethods();
});
```### Example 5: Client-to-Grain Call Validation
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AllowClientCallGrain()
.And()
.AddGrainTransition()
.AllMethods();
});
```### Example 6: Detecting and Preventing Circular Dependencies
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AddGrainTransition()
.AllMethods()
.And()
.AddGrainTransition()
.AllMethods()
.And()
.AddGrainTransition()
.AllMethods();
});
```### Example 7: Simple Self-Call
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AddGrain()
.WithReentrancy();
});
```### Example 8: Self-Call with All Methods
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AddGrain()
.AllMethods()
.WithReentrancy();
});
```### Example 9: Self-Call with Specific Method and Parameters
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AddGrain()
.Method(a => a.MethodA1(GraphParam.Any()))
.WithReentrancy();
});
```### Example 10: Self-Call with Client Call Validation
```csharp
builder.AddOrleansGraph()
.CreateGraph(graph =>
{
graph.AddGrain()
.AllowClientCallGrain()
.WithReentrancy();
});
```These examples demonstrate various ways to configure a grain to call itself, including method-level granularity, reentrancy, and client call validation.
## LicenseThis project is licensed under the MIT License.
## Contributing
Contributions are welcome! Please feel free to submit a Pull Request.
## Support
If you have any questions or run into issues, please open an issue on the [GitHub repository](https://github.com/managedcode/Orleans.Graph).
---
Created and maintained by [ManagedCode](https://github.com/managedcode)