Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/markaren/three.kt
Three.js port for the JVM (desktop)
https://github.com/markaren/three.kt
3d-graphics java jvm kotlin lwjgl3 opengl threejs
Last synced: 1 day ago
JSON representation
Three.js port for the JVM (desktop)
- Host: GitHub
- URL: https://github.com/markaren/three.kt
- Owner: markaren
- License: mit
- Created: 2019-07-12T08:53:41.000Z (about 5 years ago)
- Default Branch: master
- Last Pushed: 2022-12-27T23:36:49.000Z (almost 2 years ago)
- Last Synced: 2023-12-08T17:12:28.955Z (10 months ago)
- Topics: 3d-graphics, java, jvm, kotlin, lwjgl3, opengl, threejs
- Language: Kotlin
- Homepage:
- Size: 14.8 MB
- Stars: 195
- Watchers: 11
- Forks: 15
- Open Issues: 7
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# three.kt (Work in progress)
[](https://opensource.org/licenses/MIT)
[](https://github.com/markaren/three.kt/issues)[](https://github.com/markaren/three.kt/actions)
[](https://gitter.im/markaren/three.kt?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge)Kotlin/JVM port of the popular [three.js](http://threejs.org) 3D library ([r106](https://github.com/mrdoob/three.js/tree/r106)).
Be warned, while the basics works, such as:
* Primitives, Points and TubeGeometry
* All materials and lights
* OrbitControls
* 2D textures
* Raycasting against Mesh
* OBJ, MTL and STL loaders
* Other stuff like mirror, sky and water shaders
a lot of features are still missing and the API can change rapidly.Right now, this is mostly interesting for developers that want to contribute.
#### API (subject to changes)
##### Kotlin
```kotlin
Window(antialias = 4).use { window ->
val scene = Scene().apply {
setBackground(Color.aliceblue)
}val camera = PerspectiveCamera(75, window.aspect, 0.1, 1000).apply {
position.z = 5f
}val renderer = GLRenderer(window.size)
val box = Mesh(BoxGeometry(1f), MeshBasicMaterial().apply {
color.set(0x00ff00)
})
scene.add(box)
val clock = Clock()
val controls = OrbitControls(camera, window)
window.animate {
val dt = clock.getDelta()
box.rotation.x += 1f * dt
box.rotation.y += 1f * dtrenderer.render(scene, camera)
}
}
```##### Java
```java
public class JavaExample {public static void main(String[] args) {
try (Window window = new Window()) {
Scene scene = new Scene();
PerspectiveCamera camera = new PerspectiveCamera();
camera.getPosition().z = 5;
GLRenderer renderer = new GLRenderer(window.getSize());BoxBufferGeometry boxBufferGeometry = new BoxBufferGeometry();
MeshPhongMaterial boxMaterial = new MeshPhongMaterial();
boxMaterial.getColor().set(Color.getRoyalblue());Mesh box = new Mesh(boxBufferGeometry, boxMaterial);
scene.add(box);MeshBasicMaterial wireframeMaterial = new MeshBasicMaterial();
wireframeMaterial.getColor().set(0x000000);
wireframeMaterial.setWireframe(true);
Mesh wireframe = new Mesh(box.getGeometry().clone(), wireframeMaterial);
scene.add(wireframe);AmbientLight light = new AmbientLight();
scene.add(light);OrbitControls orbitControls = new OrbitControls(camera, window);
window.animate(() -> {
renderer.render(scene, camera);
});}
}
}
```
Artifacts are available through Maven central:
```
repositories {
mavenCentral()
}dependencies {
def version = "..."
implementation "info.laht.threekt:core:$version"
}
```## Screenshots
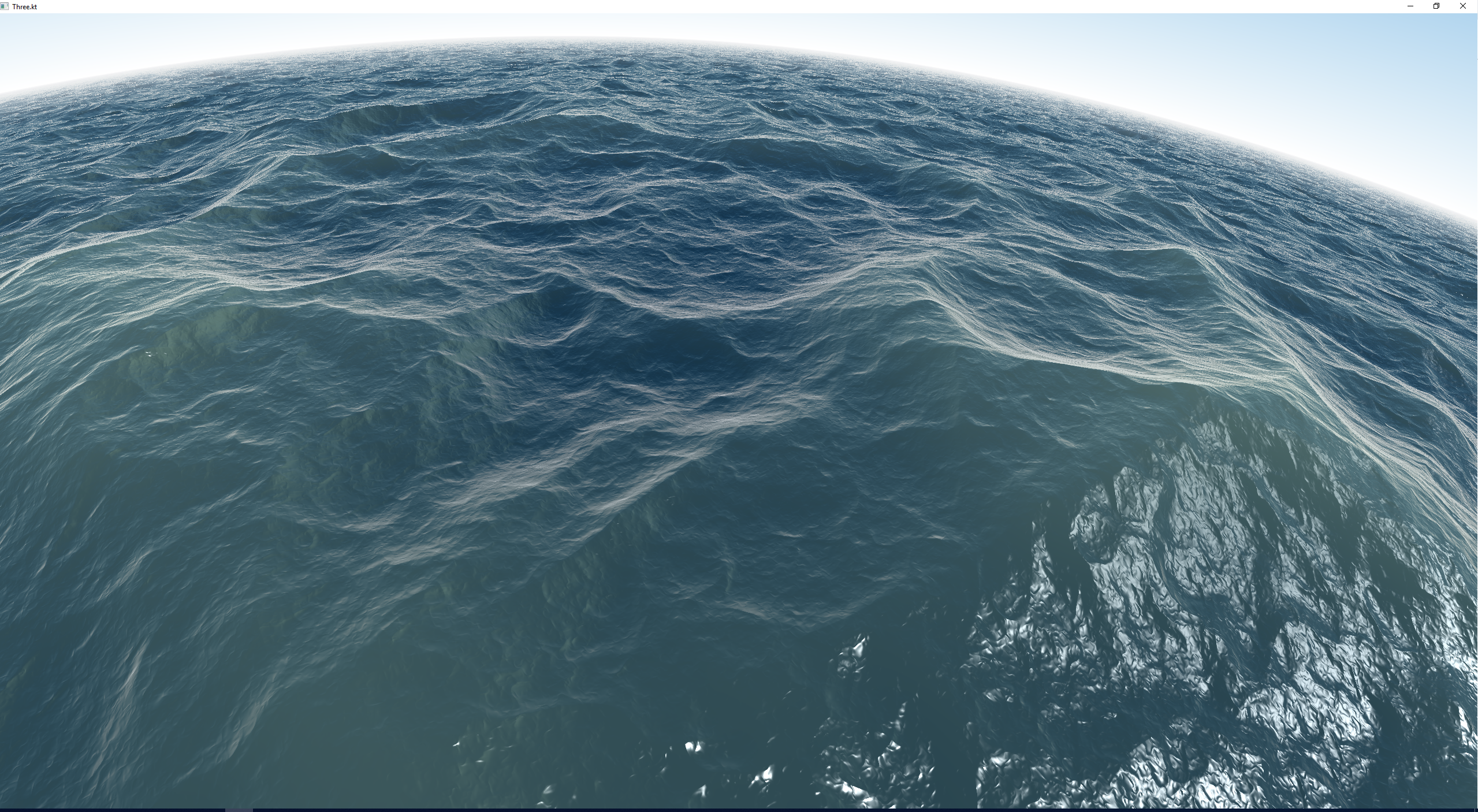
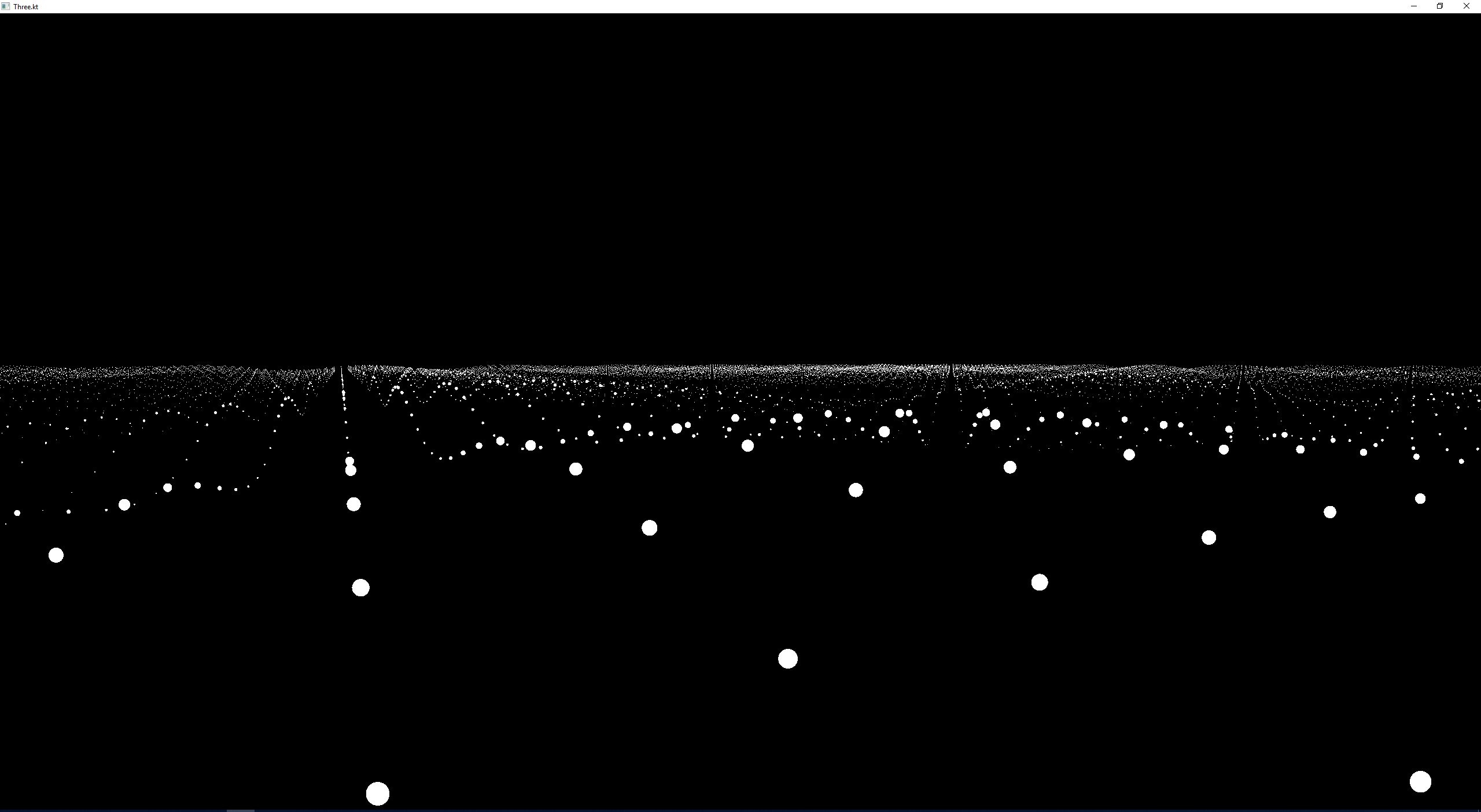
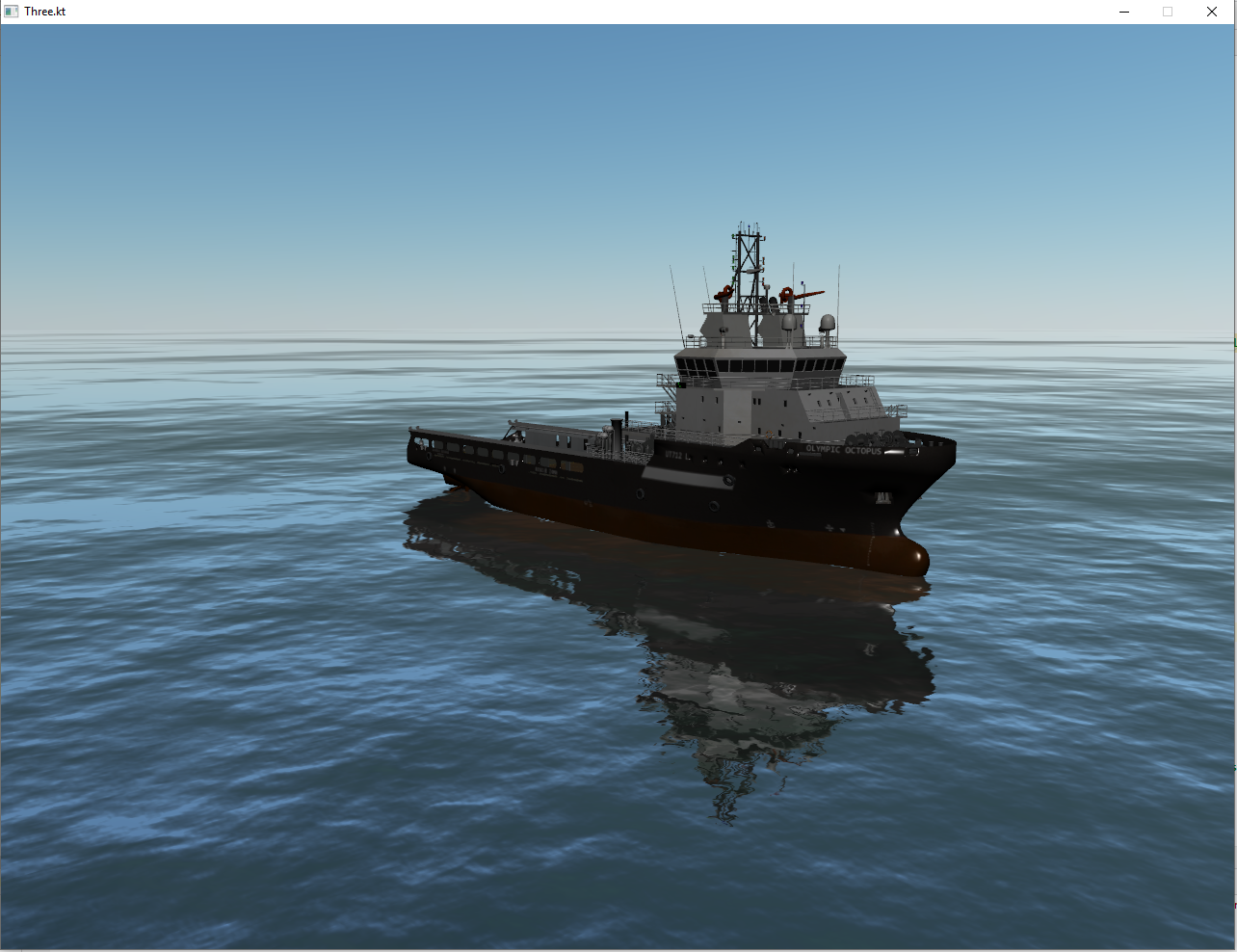
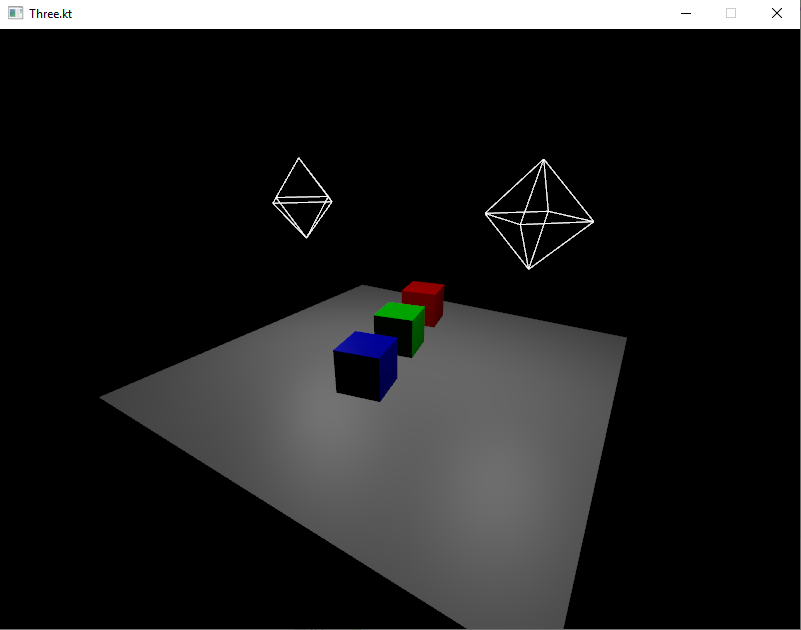## Looking for the Kotlin/JS wrapper project?
It has been renamed and moved to [here](https://github.com/markaren/three-kt-wrapper).