Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/matejbransky/react-katex
Display math in TeX with KaTeX and ReactJS
https://github.com/matejbransky/react-katex
katex latex math react
Last synced: 2 days ago
JSON representation
Display math in TeX with KaTeX and ReactJS
- Host: GitHub
- URL: https://github.com/matejbransky/react-katex
- Owner: MatejBransky
- License: mit
- Created: 2018-11-06T12:07:38.000Z (about 6 years ago)
- Default Branch: master
- Last Pushed: 2024-09-24T17:17:26.000Z (3 months ago)
- Last Synced: 2024-10-14T19:21:07.347Z (2 months ago)
- Topics: katex, latex, math, react
- Language: TypeScript
- Homepage: https://codesandbox.io/s/github/MatejMazur/react-katex/tree/master/demo?fontsize=14&hidenavigation=1&module=%2Fsrc%2FExample.tsx&theme=dark
- Size: 464 KB
- Stars: 147
- Watchers: 5
- Forks: 12
- Open Issues: 19
-
Metadata Files:
- Readme: README.md
- License: LICENSE
- Code of conduct: CODE_OF_CONDUCT.md
Awesome Lists containing this project
README
react-katex
Display math expressions with KaTeX and React.
![]()
(based on the https://github.com/talyssonoc/react-katex)
(the readme and the demo are "forked" from https://github.com/talyssonoc/react-katex)
Comparison with `react-katex`: https://github.com/talyssonoc/react-katex/issues/49.

## Installation
```sh
$ npm install katex @matejmazur/react-katex
# or
$ yarn add katex @matejmazur/react-katex
```## Usage
```jsx
import 'katex/dist/katex.min.css';
import TeX from '@matejmazur/react-katex';
```### Inline math
Display math in the middle of the text.
```jsx
ReactDOM.render(
,
document.getElementById('math')
);// or
ReactDOM.render(
\int_0^\infty x^2 dx,
document.getElementById('math')
);
```It will be rendered like this:

### Block math
Display math in a separated block, with larger font and symbols.
```jsx
ReactDOM.render(
,
document.getElementById('math')
);// or
ReactDOM.render(
\int_0^\infty x^2 dx,
document.getElementById('math')
);
```It will be rendered like this:
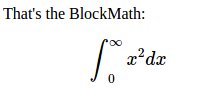
**Note:**
Don't forget to import KaTeX CSS file.```jsx
import 'katex/dist/katex.min.css';
```### Error handling
#### Default error message
By default the error rendering is handled by KaTeX. You can optionally pass `errorColor` (defaults to `#cc0000`) as a prop:
```jsx
ReactDOM.render(
,
document.getElementById('math')
);
```This will be rendered like so:
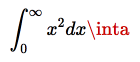
#### Custom error message
It's possible to handle parse errors using the prop `renderError`. This prop must be a function that receives the error object and returns what should be rendered when parsing fails:
```jsx
ReactDOM.render(
{
return Fail: {error.name};
}}
/>,
document.getElementById('math')
);// The code above will render 'Fail: ParseError' because it's the value returned from `renderError`.
```This will render `Fail: ParseError`:
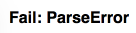
#### Custom wrapper component
You can change the wrapper component (block math uses `div` and inline uses `span`) by whatever you want via `props.as` attribute.
```jsx
ReactDOM.render(
,
document.getElementById('math')
);
```### Escaping expressions
In addition to using the `math` property, you can also quote as a child allowing the use of `{ }` in your expression.
```jsx
ReactDOM.render(
{'\\frac{\\text{m}}{\\text{s}^2}'},
document.getElementById('math')
);
```Or Multiline
```jsx
ReactDOM.render(
{`\\frac{\\text{m}}
{\\text{s}^2}`},
document.getElementById('math')
);
```However, it can be annoying to escape backslashes. This can be circumvented with the `String.raw` tag on a template literal when using ES6.
```jsx
ReactDOM.render(
{String.raw`\frac{\text{m}}{\text{s}^2}`},
document.getElementById('math')
);
```Backticks must be escaped with a backslash but would be passed to KaTeX as \\\`. A tag can be created to replace \\\` with \`
```jsx
const latex = (...a) => String.raw(...a).replace('\\`', '`');
ReactDOM.render({latex`\``}, document.getElementById('math'));
```You can even do variable substitution
```jsx
const top = 'm';
const bottom = 's';
ReactDOM.render(
{String.raw`\frac{\text{${top}}}{\text{${bottom}}^2}`},
document.getElementById('math')
);
```### Configuring KaTeX
You can change directly [all KaTeX options](https://katex.org/docs/options.html) via `props.settings`:
**Example of adding a custom macro:**
```jsx
ReactDOM.render(
y = k * x + c
);
```Result:
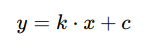