Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/matinghanbari/telegramasdatabase
This .NET-based project integrates with Telegram's free cloud storage services to provide a database solution. It leverages Telegram's cloud infrastructure to store and manage data efficiently, allowing users to access and update the database via a Telegram bot interface.
https://github.com/matinghanbari/telegramasdatabase
data database dotnet dotnet-core dotnet8 json telegram telegram-bot
Last synced: 1 day ago
JSON representation
This .NET-based project integrates with Telegram's free cloud storage services to provide a database solution. It leverages Telegram's cloud infrastructure to store and manage data efficiently, allowing users to access and update the database via a Telegram bot interface.
- Host: GitHub
- URL: https://github.com/matinghanbari/telegramasdatabase
- Owner: MatinGhanbari
- License: mit
- Created: 2024-12-17T10:30:50.000Z (12 days ago)
- Default Branch: main
- Last Pushed: 2024-12-24T15:20:31.000Z (4 days ago)
- Last Synced: 2024-12-24T16:25:28.850Z (4 days ago)
- Topics: data, database, dotnet, dotnet-core, dotnet8, json, telegram, telegram-bot
- Language: C#
- Homepage: https://nuget.org/packages/TelegramAsDatabase
- Size: 208 KB
- Stars: 7
- Watchers: 1
- Forks: 2
- Open Issues: 24
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# TDB: Telegram as database service
This project is a .NET application that utilizes Telegram's free cloud storage to provide a simple, scalable database solution. It enables data storage and management through a Telegram bot, allowing users to interact with the database directly via Telegram. By leveraging Telegram's cloud infrastructure, the project eliminates the need for traditional database setups, offering a lightweight and efficient alternative for small to medium-scale data storage needs.### Now telegram as database is availabe on nuget.org: [click here](https://www.nuget.org/packages/TelegramAsDatabase)
## Features
1. **Asynchronous Operations:** All methods are asynchronous (using `Task`) to avoid blocking the calling thread.
1. **Transaction Support:** Offers the ability to begin a transaction for atomic operations.
1. **Generic Interface:** The interface is generic (`ITDB`) allowing it to work with various data types.
1. **Data Model:** Uses a generic data model (`TDBData`) to encapsulate data and metadata.
1. **FluentResults Integration:** Leverages the FluentResults library for robust error handling and reporting.
1. **Cancellation Support:** All methods accept an optional `CancellationToken` for graceful cancellation.
1. **Key-Value Storage:** Primarily designed for key-value based data storage and retrieval.
1. **Basic CRUD Operations:** Supports common operations like Get, Set, Update, Delete, and Exists.
1. **Bulk Operations:** Includes methods for saving and deleting multiple items in bulk.
1. **Key Retrieval:** Provides a method to retrieve all keys currently stored.
1. **Retry Policy:** Implements a retry policy to enhance resilience against transient failures. This policy can be configured to retry operations a specified number of times with optional backoff strategies, improving the overall reliability and availability of the service.# Getting start
1. Create a bot using bot father in telegram (Important: **Dont Change the bot description at all**)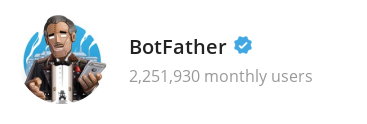
2. Create a channel as a database source in telegram
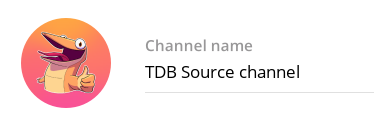
3. Now add your bot that created in the first step to the channel as an administrator and give all premissions to it.
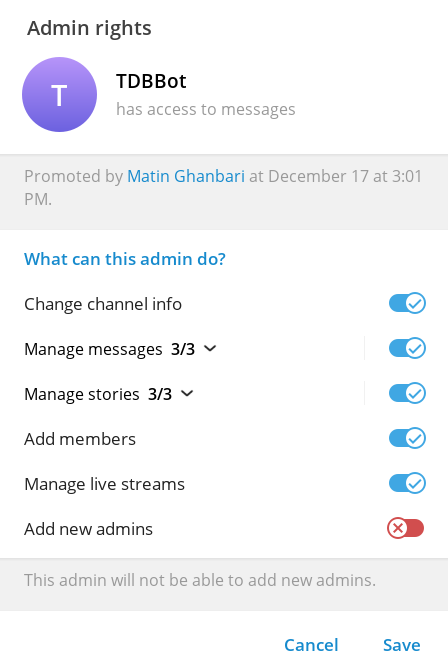
4. Goto your appsettings and add TDB config to it.:
- The api key is key that you get from BotFather in the first step
- The Channel Id is the id of the channel you can get it by forwarding a message of the channel to @userinfobot bot.
```json
"TDBConfig": {
"ApiKey": "7753344678:AAFf4MIQSShxa4djp172DjhDe2_jqRsyOeU",
"ChannelId": "-1002378130994"
}
```
5. Now Add Tdb to your service container:```csharp
var configurations = builder.Configuration;
builder.Services.AddTDB(configurations);
```6. Done! now you can use the telegram free database
# How to use
The example code of using the TDB:
```csharp
public class MyCustomService
{
private ITDB _tdbService;public MyCustomService(ITDB tdb)
{
_tdbService = tdb;
}public async Task MyCustomMethod(CancellationToken cancellationToken)
{
// [--------- GetAllKeysAsync ---------]
var allKeys = await _tdbService.GetAllKeysAsync(cancellationToken);// [------------ SaveAsync ------------]
var saveResult = await _tdbService.SaveAsync(new TDBData()
{
Key = "item-key",
Value = new MyTestModel()
{
Name = "FirstTest",
Description = "FirstDescription",
Type = "FuncTest"
}
}, cancellationToken);// [--------- UpdateAsync ---------]
var updateResult = await _tdbService.UpdateAsync("item-key", new TDBData()
{
Key = "item-key",
Value = new MyTestModel()
{
Name = "FirstTest2",
Description = "FirstDescription2",
Type = "FuncTest2"
}
}, cancellationToken);// [--------- DeleteAsync ---------]
var deleteResult = await _tdbService.DeleteAsync("item-key", cancellationToken);
}
}
```