Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/mazenrashed/RxPagination
Implement pagination in just few lines with RxPagination
https://github.com/mazenrashed/RxPagination
pagination recyclerview rx rxandroid rxjava rxjava2 rxkotlin rxpagination rxrelay
Last synced: 3 months ago
JSON representation
Implement pagination in just few lines with RxPagination
- Host: GitHub
- URL: https://github.com/mazenrashed/RxPagination
- Owner: mazenrashed
- License: apache-2.0
- Created: 2020-03-16T18:44:10.000Z (almost 5 years ago)
- Default Branch: master
- Last Pushed: 2020-05-07T00:55:27.000Z (over 4 years ago)
- Last Synced: 2024-07-03T04:06:49.237Z (7 months ago)
- Topics: pagination, recyclerview, rx, rxandroid, rxjava, rxjava2, rxkotlin, rxpagination, rxrelay
- Language: Kotlin
- Size: 155 KB
- Stars: 20
- Watchers: 4
- Forks: 1
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE.md
Awesome Lists containing this project
- awesome-jordan - RxPagination - Implement the pagination in a few lines. (Android)
README
# RxPagination
[](https://jitpack.io/#mazenrashed/RxPagination)
Implement the pagination in a few lines,
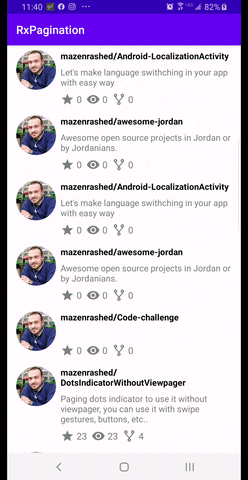
### Add the JitPack repository to your build file
```groovy
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
```
### Add dependency
```groovy
dependencies {
implementation 'com.github.mazenrashed:RxPagination:${LAST_VERSION}'
}
```
# Usage
We will use the car example,
## In Your repository
Jest implement ListRepository\
```kotlin
class CarRepository : ListRepository{
...
}
```Then, you need to override getDataList(page: Int, pageSize : Int) inside the repository
```kotlin
class CarRepository : ListRepository {
override fun getDataList(page: Int, pageSize : Int): Single> {
return endPoints.getCars(pageSize, page) //For Example (You need to return your data source here)
}
}
```## In The View Model
Inehet from RxPagination\
```kotlin
class CarsViewModel(carsRepository: CarRepository) :
RxPagination(carsRepository, PAGE_SIZE, FIRST_PAGE) {
init {
loadDataList()
}
}
```
Then, you need to override onFetchDataListSubscribed,
onFetchDataListError and onFetchDataListSuccess
```kotlin
class CarsViewModel(carsRepository: CarRepository) :
RxPagination(carsRepository, PAGE_SIZE, FIRST_PAGE) {
init {
loadDataList()
}
override fun onFetchDataListSubscribed() {
//Start fetching data
}
override fun onFetchDataListError(throwable: Throwable) {
}
override fun onFetchDataListSuccess(lastLoadedList: ArrayList) {
//You don't need to handle the data, but if you need it, it's available
//This lastLoadedList is the new loaded part.
}
}
```
## In the UI (Activity or Fragment)
To observe the data list, use viewModel.dataList,
dataList is a BehaviorRelay\\>
```kotlin
viewModel
.dataList
.observeOn(AndroidSchedulers.mainThread())
.subscribe { dataList ->
// notify your list adapter here
}
```
## Load and reload data
```kotlin
viewModel.loadDataList() //To load the more dataviewModel.reloadDataList() //To reload the data
```## Access page informations
To access cerrent page
```kotlin
viewModel.page.value //Int
```
To check if you reach the last page
```kotlin
viewModel.isLastPage.value //Boolean
```## Contributing
We welcome contributions !
* ⇄ Pull requests and ★ Stars are always welcome.