https://github.com/meshpro/dmsh
:spider_web: Simple mesh generator inspired by distmesh.
https://github.com/meshpro/dmsh
finite-elements mathematics mesh mesh-generation python python3
Last synced: about 2 months ago
JSON representation
:spider_web: Simple mesh generator inspired by distmesh.
- Host: GitHub
- URL: https://github.com/meshpro/dmsh
- Owner: meshpro
- License: gpl-3.0
- Created: 2018-07-15T20:08:22.000Z (almost 7 years ago)
- Default Branch: main
- Last Pushed: 2023-03-13T11:05:12.000Z (over 2 years ago)
- Last Synced: 2025-04-17T06:53:15.361Z (2 months ago)
- Topics: finite-elements, mathematics, mesh, mesh-generation, python, python3
- Homepage:
- Size: 1.79 MB
- Stars: 218
- Watchers: 10
- Forks: 27
- Open Issues: 5
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
- awesome-scientific-computing - dmsh - Simple generator for unstructured triangular meshes, inspired by distmesh. (Meshing / Triangular and tetrahedral meshing)
README
The worst mesh generator you'll ever use.
[](https://pypi.org/project/dmsh/)
[](https://pypi.org/project/dmsh/)
[](https://github.com/nschloe/dmsh)
[](https://pypistats.org/packages/dmsh)[](https://discord.gg/PBCCvwHqpv)
Inspired by [distmesh](http://persson.berkeley.edu/distmesh/), dmsh can be slow,
requires a lot of memory, and isn't terribly robust either.On the plus side,
- it's got a user-friendly interface,
- is pure Python (and hence easily installable on any system), and
- it produces pretty high-quality meshes.Combined with [optimesh](https://github.com/nschloe/optimesh), dmsh produces the
highest-quality 2D meshes in the west.### Examples
#### Primitives
|
|
|
|
| :--------------------------------------------------------------------------------------------------------: | :-----------------------------------------------------------------------------------------------------------: | :---------------------------------------------------------------------------------------------------------: |```python
import dmsh
import meshio
import optimeshgeo = dmsh.Circle([0.0, 0.0], 1.0)
X, cells = dmsh.generate(geo, 0.1)# optionally optimize the mesh
X, cells = optimesh.optimize_points_cells(X, cells, "CVT (full)", 1.0e-10, 100)# visualize the mesh
dmsh.show(X, cells, geo)# and write it to a file
meshio.Mesh(X, {"triangle": cells}).write("circle.vtk")
``````python
import dmshgeo = dmsh.Rectangle(-1.0, +2.0, -1.0, +1.0)
X, cells = dmsh.generate(geo, 0.1)
``````python
import dmshgeo = dmsh.Polygon(
[
[0.0, 0.0],
[1.1, 0.0],
[1.2, 0.5],
[0.7, 0.6],
[2.0, 1.0],
[1.0, 2.0],
[0.5, 1.5],
]
)
X, cells = dmsh.generate(geo, 0.1)
```#### Combinations
##### Difference
|
|
|
|
| :-----------------------------------------------------------------------------------------: | :-------------------------------------------------------------------------------------------: | :--------------------------------------------------------------------------------------------------------------: |```python
import dmshgeo = dmsh.Circle([-0.5, 0.0], 1.0) - dmsh.Circle([+0.5, 0.0], 1.0)
X, cells = dmsh.generate(geo, 0.1)
``````python
import dmshgeo = dmsh.Circle([0.0, 0.0], 1.0) - dmsh.Polygon([[0.0, 0.0], [1.5, 0.4], [1.5, -0.4]])
X, cells = dmsh.generate(geo, 0.1, tol=1.0e-10)
```The following example uses a nonconstant edge length; it depends on the distance to the
circle `c`.```python
import dmsh
import numpy as npr = dmsh.Rectangle(-1.0, +1.0, -1.0, +1.0)
c = dmsh.Circle([0.0, 0.0], 0.3)
geo = r - cX, cells = dmsh.generate(geo, lambda pts: np.abs(c.dist(pts)) / 5 + 0.05, tol=1.0e-10)
```##### Union
|
|
|
|
| :--------------------------------------------------------------------------------------------------: | :-----------------------------------------------------------------------------------------------------: | :--------------------------------------------------------------------------------------------------------: |```python
import dmshgeo = dmsh.Circle([-0.5, 0.0], 1.0) + dmsh.Circle([+0.5, 0.0], 1.0)
X, cells = dmsh.generate(geo, 0.15)
``````python
import dmshgeo = dmsh.Rectangle(-1.0, +0.5, -1.0, +0.5) + dmsh.Rectangle(-0.5, +1.0, -0.5, +1.0)
X, cells = dmsh.generate(geo, 0.15)
``````python
import dmsh
import numpy as npangles = np.pi * np.array([3.0 / 6.0, 7.0 / 6.0, 11.0 / 6.0])
geo = dmsh.Union(
[
dmsh.Circle([np.cos(angles[0]), np.sin(angles[0])], 1.0),
dmsh.Circle([np.cos(angles[1]), np.sin(angles[1])], 1.0),
dmsh.Circle([np.cos(angles[2]), np.sin(angles[2])], 1.0),
]
)
X, cells = dmsh.generate(geo, 0.15)
```#### Intersection
|
|
|
|
| :---------------------------------------------------------------------------------------------------------: | :---------------------------------------------------------------------------------------------------------------: | :------------------------------------------------------------------------------------------------------------------: |```python
import dmshgeo = dmsh.Circle([0.0, -0.5], 1.0) & dmsh.Circle([0.0, +0.5], 1.0)
X, cells = dmsh.generate(geo, 0.1, tol=1.0e-10)
``````python
import dmsh
import numpy as npangles = np.pi * np.array([3.0 / 6.0, 7.0 / 6.0, 11.0 / 6.0])
geo = dmsh.Intersection(
[
dmsh.Circle([np.cos(angles[0]), np.sin(angles[0])], 1.5),
dmsh.Circle([np.cos(angles[1]), np.sin(angles[1])], 1.5),
dmsh.Circle([np.cos(angles[2]), np.sin(angles[2])], 1.5),
]
)
X, cells = dmsh.generate(geo, 0.1, tol=1.0e-10)
```The following uses the `HalfSpace` primtive for cutting off a circle.
```python
import dmshgeo = dmsh.HalfSpace([1.0, 1.0]) & dmsh.Circle([0.0, 0.0], 1.0)
X, cells = dmsh.generate(geo, 0.1)
```### Rotation, translation, scaling
|
|
|
| :---------------------------------------------------------------------------------------------: | :--------------------------------------------------------------------------------------------: |```python
import dmsh
import numpy as npgeo = dmsh.Rotation(dmsh.Rectangle(-1.0, +2.0, -1.0, +1.0), 0.1 * np.pi)
X, cells = dmsh.generate(geo, 0.1, tol=1.0e-10)
``````python
import dmshgeo = dmsh.Rectangle(-1.0, +2.0, -1.0, +1.0) + [1.0, 1.0]
X, cells = dmsh.generate(geo, 0.1)
``````python
import dmshgeo = dmsh.Rectangle(-1.0, +2.0, -1.0, +1.0) * 2.0
X, cells = dmsh.generate(geo, 0.1, tol=1.0e-5)
```### Local refinement
All objects can be used to refine the mesh according to the distance to the object;
e.g. a `Path`:```python
import dmshgeo = dmsh.Rectangle(0.0, 1.0, 0.0, 1.0)
p1 = dmsh.Path([[0.4, 0.6], [0.6, 0.4]])
def target_edge_length(x):
return 0.03 + 0.1 * p1.dist(x)X, cells = dmsh.generate(geo, target_edge_length, tol=1.0e-10)
```### Custom shapes
It is also possible to define your own geometry. Simply create a class derived from
`dmsh.Geometry` that contains a `dist` method and a method to project points onto the
boundary.```python
import dmsh
import numpy as npclass MyDisk(dmsh.Geometry):
def __init__(self):
self.r = 1.0
self.x0 = [0.0, 0.0]
bounding_box = [-1.0, 1.0, -1.0, 1.0]
feature_points = np.array([[], []]).T
super().__init__(bounding_box, feature_points)def dist(self, x):
assert x.shape[0] == 2
y = (x.T - self.x0).T
return np.sqrt(np.einsum("i...,i...->...", y, y)) - self.rdef boundary_step(self, x):
# project onto the circle
y = (x.T - self.x0).T
r = np.sqrt(np.einsum("ij,ij->j", y, y))
return ((y / r * self.r).T + self.x0).Tgeo = MyDisk()
X, cells = dmsh.generate(geo, 0.1)
```### Debugging
| 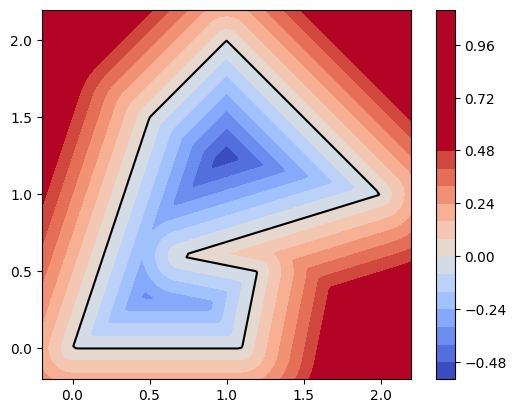 | 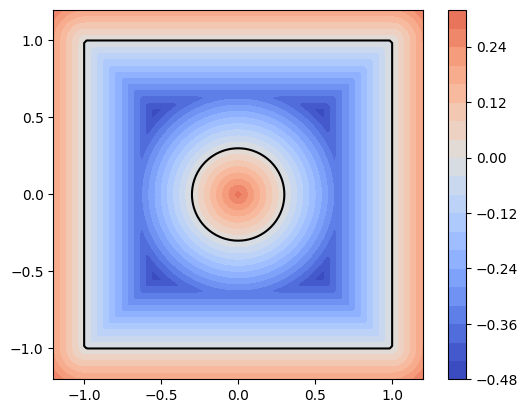 |
| :-----------------------------------------------------------------------------------------------: | :------------------------------------------------------------------------------------------------------: |dmsh is rather fragile, but sometimes the break-downs are due to an incorrectly defined
geometry. Use```
geo.show()
```to inspect the level set function of your domain. (It must be negative inside the
domain and positive outside. The 0-level set forms the domain boundary.)### Installation
dmsh is [available from the Python Package
Index](https://pypi.org/project/dmsh/), so simply type```
pip install dmsh
```to install.
### Testing
To run the dmsh unit tests, check out this repository and type
```
tox
```