Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/mhmtszr/pipeline
Golang pipeline solution with generics.
https://github.com/mhmtszr/pipeline
chainofresponsibility go golang pipeline
Last synced: 2 months ago
JSON representation
Golang pipeline solution with generics.
- Host: GitHub
- URL: https://github.com/mhmtszr/pipeline
- Owner: mhmtszr
- License: mit
- Created: 2023-03-17T05:30:03.000Z (almost 2 years ago)
- Default Branch: master
- Last Pushed: 2024-07-09T11:27:00.000Z (6 months ago)
- Last Synced: 2024-10-17T00:13:48.260Z (3 months ago)
- Topics: chainofresponsibility, go, golang, pipeline
- Language: Go
- Homepage:
- Size: 23.4 KB
- Stars: 33
- Watchers: 1
- Forks: 1
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# Pipeline [![GoDoc][doc-img]][doc] [![Build Status][ci-img]][ci] [![Coverage Status][cov-img]][cov] [![Go Report Card][go-report-img]][go-report]
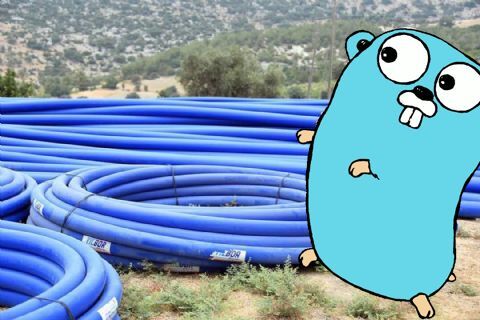
Go pipeline solution that can be used in many different combinations for chaining pipeline steps.
Inspired by [@bilal-kilic](https://github.com/bilal-kilic)'s Kotlin implementation [boru](https://github.com/Trendyol/boru).
### Usage
Supports 1.18+ Go versions because of Go Generics
```
go get github.com/mhmtszr/pipeline
```
### Examples
#### Basic Pipeline
``` go
package mainimport (
"fmt"
"github.com/mhmtszr/pipeline"
)type Square struct{}
type Add struct{}func (s Square) Execute(context int, next func(context int)) error {
context = context * context
println(fmt.Sprintf("After first chain context: %d", context))
return next(context)
}func (a Add) Execute(context int, next func(context int)) {
context = context + context
println(fmt.Sprintf("After second chain context: %d", context))
return next(context)
}func main() {
p, _ := pipeline.Builder[int]{}.UsePipelineStep(Square{}).UsePipelineStep(Add{}).Build()
p.Execute(3)
}
// After first chain context: 9
// After second chain context: 18```
#### Conditional Pipeline
``` go
p := pipeline.Builder[*int]{}.
UseConditionalStepBuilder(
pipeline.NewConditionalStepBuilder[*int]().
Condition(func(context *int) bool {
return *context == 3
}).
IfTrue(Square{}).
IfFalse(Add{}),
).UsePipelineStep(Add{}).Build()
nm := 3
_ = p.Execute(&nm)// nm 18
nm = 4
_ = p.Execute(&nm)// nm 16
```
[doc-img]: https://godoc.org/github.com/mhmtszr/pipeline?status.svg
[doc]: https://godoc.org/github.com/mhmtszr/pipeline
[ci-img]: https://github.com/mhmtszr/pipeline/actions/workflows/build-test.yml/badge.svg
[ci]: https://github.com/mhmtszr/pipeline/actions/workflows/build-test.yml
[cov-img]: https://codecov.io/gh/mhmtszr/pipeline/branch/master/graph/badge.svg
[cov]: https://codecov.io/gh/mhmtszr/pipeline
[go-report-img]: https://goreportcard.com/badge/github.com/mhmtszr/pipeline
[go-report]: https://goreportcard.com/report/github.com/mhmtszr/pipeline