https://github.com/mini-software/MiniWord
.NET Word(docx) exporting template engine without COM+ & interop (support Linux and Mac)
https://github.com/mini-software/MiniWord
csharp dotnet office word
Last synced: 26 days ago
JSON representation
.NET Word(docx) exporting template engine without COM+ & interop (support Linux and Mac)
- Host: GitHub
- URL: https://github.com/mini-software/MiniWord
- Owner: mini-software
- License: apache-2.0
- Created: 2022-09-11T15:37:27.000Z (over 2 years ago)
- Default Branch: main
- Last Pushed: 2025-02-04T08:34:17.000Z (3 months ago)
- Last Synced: 2025-04-18T04:57:50.317Z (27 days ago)
- Topics: csharp, dotnet, office, word
- Language: C#
- Homepage:
- Size: 1.08 MB
- Stars: 670
- Watchers: 8
- Forks: 86
- Open Issues: 44
-
Metadata Files:
- Readme: README.md
- Funding: .github/FUNDING.yml
- License: LICENSE
Awesome Lists containing this project
README
---
---
---
## Introduction
MiniWord is an easy and effective .NET Word Template library.
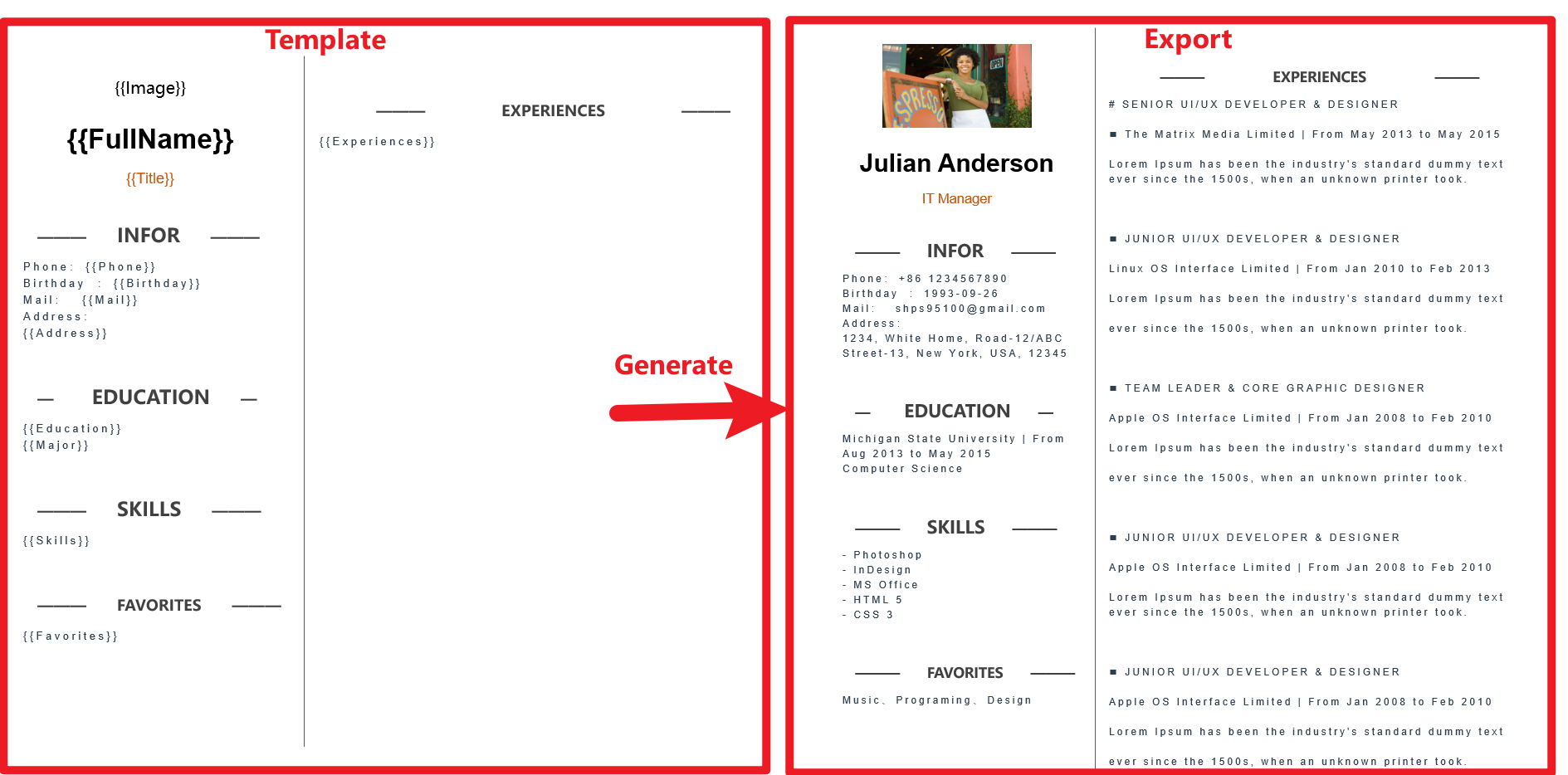
## Getting Started
### Installation
- nuget link : https://www.nuget.org/packages/MiniWord
### Quick Start
Template follow "WHAT you see is what you get" design,and the template tag styles are completely preserved.
```csharp
var value = new Dictionary(){["title"] = "Hello MiniWord"};
MiniSoftware.MiniWord.SaveAsByTemplate(outputPath, templatePath, value);
```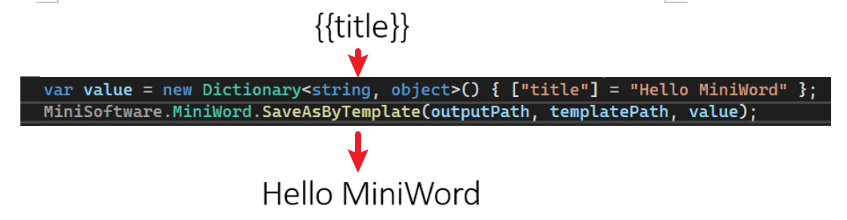
### Input, Output
- Input support file path, byte[]
- Output support file path, byte[], stream```csharp
SaveAsByTemplate(string path, string templatePath, Dictionary value)
SaveAsByTemplate(string path, byte[] templateBytes, Dictionary value)
SaveAsByTemplate(this Stream stream, string templatePath, Dictionary value)
SaveAsByTemplate(this Stream stream, byte[] templateBytes, Dictionary value)
```## Tags
MiniWord template format string like Vue, React `{{tag}}`,users only need to make sure tag and value parameter key same then system will replace them automatically.
### Text
```csharp
{{tag}}
```##### Example
```csharp
var value = new Dictionary()
{
["Name"] = "Jack",
["Department"] = "IT Department",
["Purpose"] = "Shanghai site needs a new system to control HR system.",
["StartDate"] = DateTime.Parse("2022-09-07 08:30:00"),
["EndDate"] = DateTime.Parse("2022-09-15 15:30:00"),
["Approved"] = true,
["Total_Amount"] = 123456,
};
MiniWord.SaveAsByTemplate(path, templatePath, value);
```##### Template

##### Result

### Image
Value type is `MiniWordPicture`
##### Example
```csharp
var value = new Dictionary()
{
["Logo"] = new MiniWordPicture() { Path= PathHelper.GetFile("DemoLogo.png"), Width= 180, Height= 180 }
};
MiniWord.SaveAsByTemplate(path, templatePath, value);
```##### Template
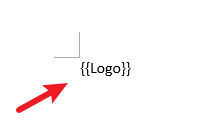
##### Result
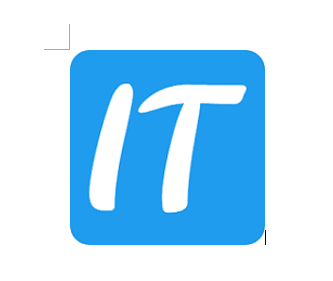
### List
tag value is `string[]` or `IList` type
##### Example
```csharp
var value = new Dictionary()
{
["managers"] = new[] { "Jack" ,"Alan"},
["employees"] = new[] { "Mike" ,"Henry"},
};
MiniWord.SaveAsByTemplate(path, templatePath, value);
```Template
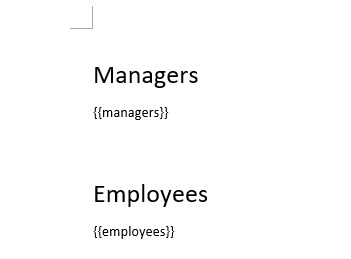
##### Result
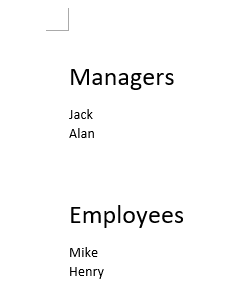
### Table
Tag value is `IEmerable>` type
##### Example
```csharp
var value = new Dictionary()
{
["TripHs"] = new List>
{
new Dictionary
{
{ "sDate",DateTime.Parse("2022-09-08 08:30:00")},
{ "eDate",DateTime.Parse("2022-09-08 15:00:00")},
{ "How","Discussion requirement part1"},
{ "Photo",new MiniWordPicture() { Path = PathHelper.GetFile("DemoExpenseMeeting02.png"), Width = 160, Height = 90 }},
},
new Dictionary
{
{ "sDate",DateTime.Parse("2022-09-09 08:30:00")},
{ "eDate",DateTime.Parse("2022-09-09 17:00:00")},
{ "How","Discussion requirement part2 and development"},
{ "Photo",new MiniWordPicture() { Path = PathHelper.GetFile("DemoExpenseMeeting01.png"), Width = 160, Height = 90 }},
},
}
};
MiniWord.SaveAsByTemplate(path, templatePath, value);
```##### Template

##### Result
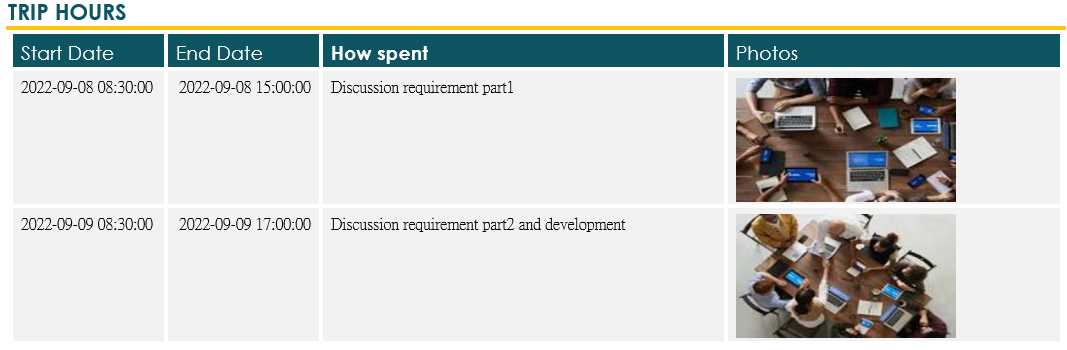
### List inside list
Tag value is `IEnumerable` type. Adding `{{foreach` and `endforeach}}` tags to template is required.
##### Example
```csharp
var value = new Dictionary()
{
["TripHs"] = new List>
{
new Dictionary
{
{ "sDate", DateTime.Parse("2022-09-08 08:30:00") },
{ "eDate", DateTime.Parse("2022-09-08 15:00:00") },
{ "How", "Discussion requirement part1" },
{
"Details", new List()
{
new MiniWordForeach()
{
Value = new Dictionary()
{
{"Text", "Air"},
{"Value", "Airplane"}
},
Separator = " | "
},
new MiniWordForeach()
{
Value = new Dictionary()
{
{"Text", "Parking"},
{"Value", "Car"}
},
Separator = " / "
}
}
}
}
}
};
MiniWord.SaveAsByTemplate(path, templatePath, value);
```##### Template
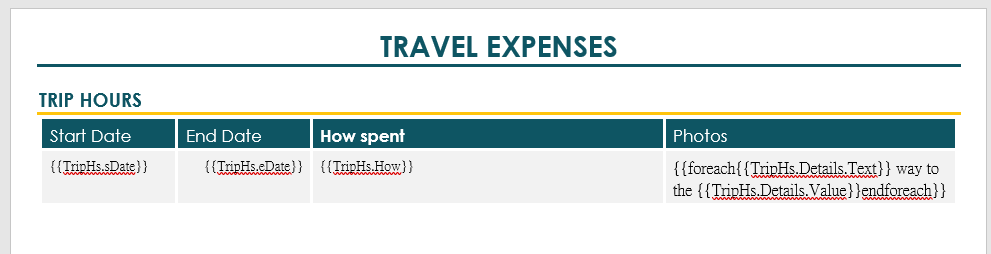
##### Result
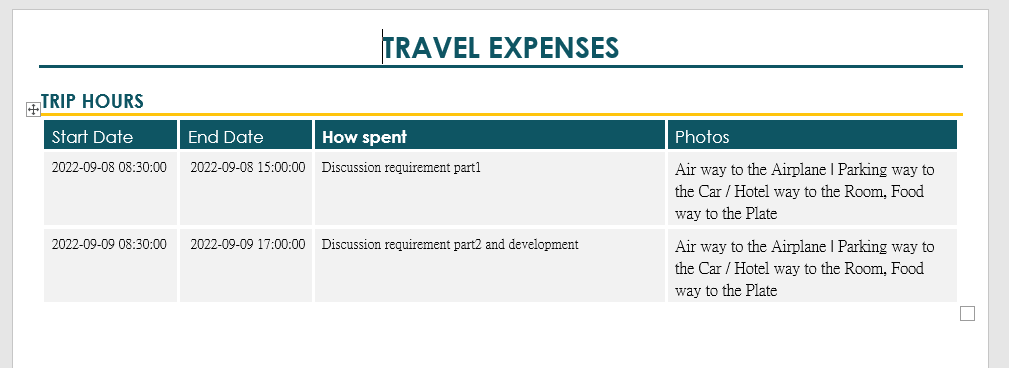
### If statement inside template
For multip paragraph, use @if and @endif tags.
For single paragraph and inside foreach, use `{{if` and `endif}}` tags to template is required.##### Example
```csharp
var value = new Dictionary()
{
["Name"] = new List(){
new MiniWordHyperLink(){
Url = "https://google.com",
Text = "測試連結22!!"
},
new MiniWordHyperLink(){
Url = "https://google1.com",
Text = "測試連結11!!"
}
},
["Company_Name"] = "MiniSofteware",
["CreateDate"] = new DateTime(2021, 01, 01),
["VIP"] = true,
["Points"] = 123,
["APP"] = "Demo APP",
};
MiniWord.SaveAsByTemplate(path, templatePath, value);
```##### Template For Multi Paragraph
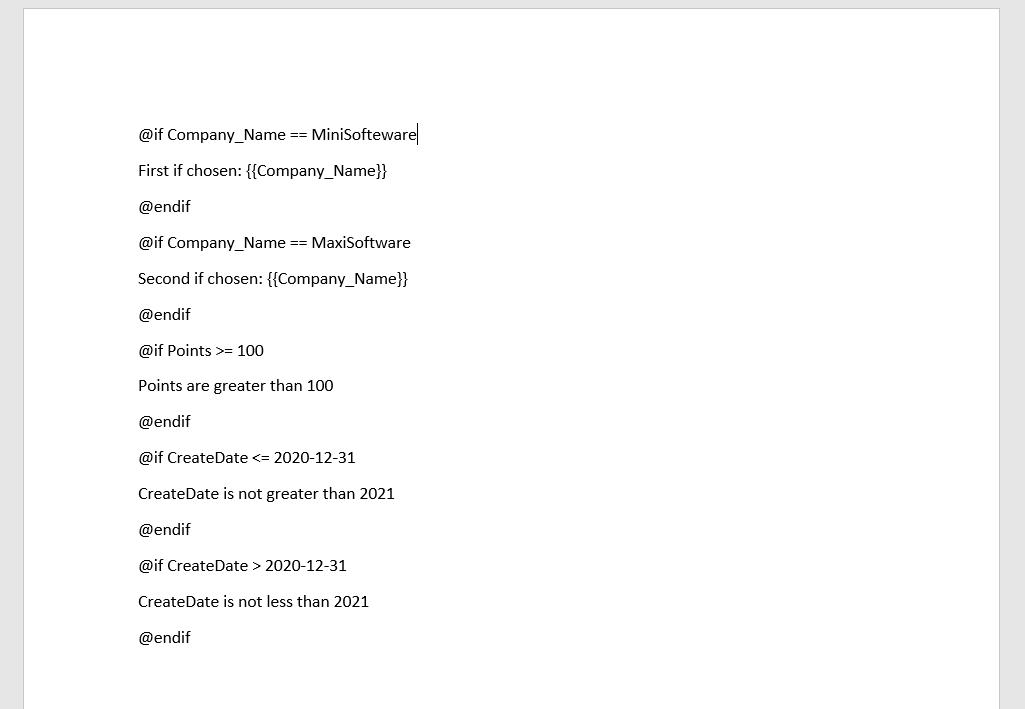
##### Result Of Multi Paragraph
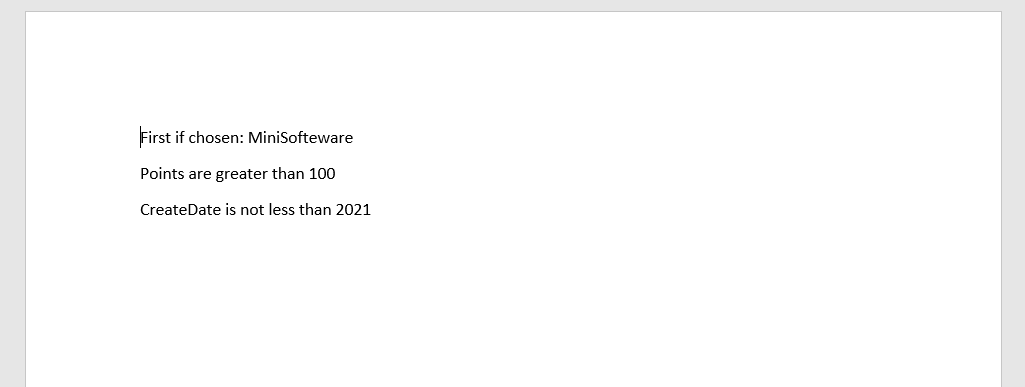
##### Template For Single Paragraph
##### Result Of Single Paragraph
### ColorText
##### Example
```csharp
var value = new
{
Company_Name = new MiniWordColorText { Text = "MiniSofteware", FontColor = "#eb70AB", },
Name = new[] {
new MiniWordColorText { Text = "Ja", HighlightColor = "#eb70AB" },
new MiniWordColorText { Text = "ck", HighlightColor = "#a56abe" }
},
CreateDate = new MiniWordColorText
{
Text = new DateTime(2021, 01, 01).ToString(),
HighlightColor = "#eb70AB",
FontColor = "#ffffff",
},
VIP = true,
Points = 123,
APP = "Demo APP",
};
MiniWord.SaveAsByTemplate(path, templatePath, value);
```## Other
### POCO or dynamic parameter
v0.5.0 support POCO or dynamic parameter
```csharp
var value = new { title = "Hello MiniWord" };
MiniWord.SaveAsByTemplate(outputPath, templatePath, value);
```### FontColor and HighlightColor
```csharp
var value = new
{
Company_Name = new MiniWordColorText { Text = "MiniSofteware", FontColor = "#eb70AB" },
Name = new MiniWordColorText { Text = "Jack", HighlightColor = "#eb70AB" },
CreateDate = new MiniWordColorText { Text = new DateTime(2021, 01, 01).ToString(), HighlightColor = "#eb70AB", FontColor = "#ffffff" },
VIP = true,
Points = 123,
APP = "Demo APP",
};
```### HyperLink
If value type is `MiniWordHyperLink` system will replace template string by hyperlink.
* Url: HyperLink URI target path
* Text:Description```csharp
var value = new
{
["Name"] = new MiniWordHyperLink(){
Url = "https://google.com",
Text = "Test Link!!"
},
["Company_Name"] = "MiniSofteware",
["CreateDate"] = new DateTime(2021, 01, 01),
["VIP"] = true,
["Points"] = 123,
["APP"] = "Demo APP",
};
MiniWord.SaveAsByTemplate(path, templatePath, value);
```## Examples
#### ASP.NET Core 3.1 API Export
```cs
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using System;
using System.Collections.Generic;
using System.IO;
using System.Net;
using MiniSoftware;public class Program
{
public static void Main(string[] args) => CreateHostBuilder(args).Build().Run();public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args).ConfigureWebHostDefaults(webBuilder => webBuilder.UseStartup());
}public class Startup
{
public void ConfigureServices(IServiceCollection services) => services.AddMvc();
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseStaticFiles();
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=api}/{action=Index}/{id?}");
});
}
}public class ApiController : Controller
{
public IActionResult Index()
{
return new ContentResult
{
ContentType = "text/html",
StatusCode = (int)HttpStatusCode.OK,
Content = @"
DownloadWordFromTemplatePath
DownloadWordFromTemplateBytes
"
};
}static Dictionary defaultValue = new Dictionary()
{
["title"] = "FooCompany",
["managers"] = new List> {
new Dictionary{{"name","Jack"},{ "department", "HR" } },
new Dictionary {{ "name", "Loan"},{ "department", "IT" } }
},
["employees"] = new List> {
new Dictionary{{ "name", "Wade" },{ "department", "HR" } },
new Dictionary {{ "name", "Felix" },{ "department", "HR" } },
new Dictionary{{ "name", "Eric" },{ "department", "IT" } },
new Dictionary {{ "name", "Keaton" },{ "department", "IT" } }
}
};public IActionResult DownloadWordFromTemplatePath()
{
string templatePath = "TestTemplateComplex.docx";Dictionary value = defaultValue;
MemoryStream memoryStream = new MemoryStream();
MiniWord.SaveAsByTemplate(memoryStream, templatePath, value);
memoryStream.Seek(0, SeekOrigin.Begin);
return new FileStreamResult(memoryStream, "application/vnd.openxmlformats-officedocument.wordprocessingml.document")
{
FileDownloadName = "demo.docx"
};
}private static Dictionary TemplateBytesCache = new Dictionary();
static ApiController()
{
string templatePath = "TestTemplateComplex.docx";
byte[] bytes = System.IO.File.ReadAllBytes(templatePath);
TemplateBytesCache.Add(templatePath, bytes);
}public IActionResult DownloadWordFromTemplateBytes()
{
byte[] bytes = TemplateBytesCache["TestTemplateComplex.docx"];Dictionary value = defaultValue;
MemoryStream memoryStream = new MemoryStream();
MiniWord.SaveAsByTemplate(memoryStream, bytes, value);
memoryStream.Seek(0, SeekOrigin.Begin);
return new FileStreamResult(memoryStream, "application/vnd.openxmlformats-officedocument.wordprocessingml.document")
{
FileDownloadName = "demo.docx"
};
}
}
```## Support : [Donate Link](https://miniexcel.github.io/)