Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/mizrael/blazorex
Blazorex is an HTML Canvas wrapper library for Blazor.
https://github.com/mizrael/blazorex
blazor blazor-webassembly canvas canvas2d game-development gamedev hacktoberfest
Last synced: about 22 hours ago
JSON representation
Blazorex is an HTML Canvas wrapper library for Blazor.
- Host: GitHub
- URL: https://github.com/mizrael/blazorex
- Owner: mizrael
- License: mit
- Created: 2022-09-19T01:51:38.000Z (over 2 years ago)
- Default Branch: master
- Last Pushed: 2025-01-20T15:19:59.000Z (6 days ago)
- Last Synced: 2025-01-20T16:19:58.605Z (6 days ago)
- Topics: blazor, blazor-webassembly, canvas, canvas2d, game-development, gamedev, hacktoberfest
- Language: C#
- Homepage: https://www.nuget.org/packages/Blazorex/
- Size: 2.19 MB
- Stars: 58
- Watchers: 3
- Forks: 8
- Open Issues: 0
-
Metadata Files:
- Readme: readme.md
- License: LICENSE
Awesome Lists containing this project
README
# Blazorex
[](https://www.nuget.org/packages/Blazorex/)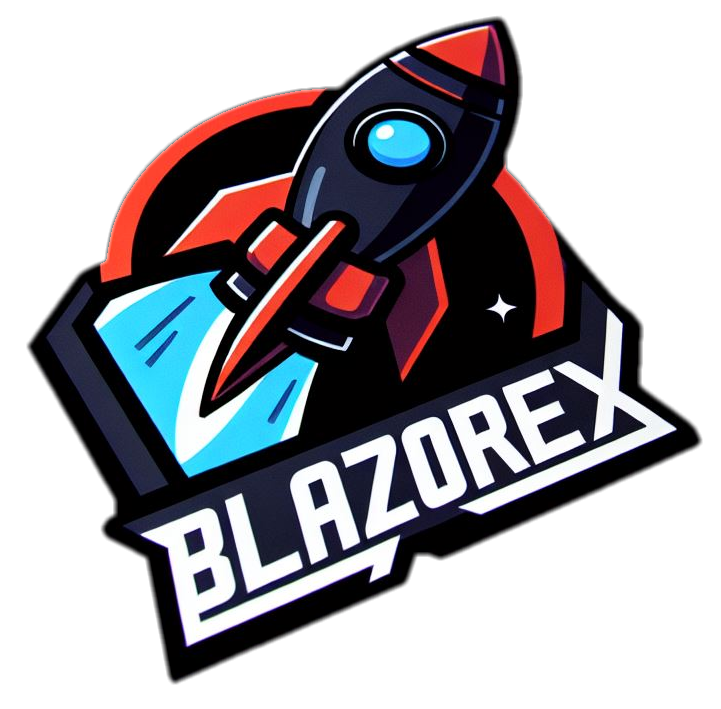
## Description
Blazorex is an HTML Canvas wrapper library for Blazor.
It has some interesting functionalities like:
- multiple canvases
- background rendering
- image rendering
- procedural image generation (yes, the fire on the background is fully procedural!
Thanks [filipedeschamps](https://github.com/filipedeschamps) for the awesome repository showing how to render the [Doom fire](https://github.com/filipedeschamps/doom-fire-algorithm)! )## Installation
Blazorex can be installed as Nuget package: https://www.nuget.org/packages/Blazorex/## Usage
### Simple scenario
Just add the `Canvas` Component to your Razor page and register to the `OnCanvasReady` to receive the `CanvasBase` instance.
Then use `OnFrameReady` to define your update/render logic:
```csharp
@code{
CanvasBase _canvas;private void OnCanvasReady(CanvasBase canvas)
{
_canvas = canvas;
}private void OnFrameReady(float timeStamp)
{
// your render logic goes here
}
}```
You might also need to update your `index.html` to include the library's CSS:
```html
```
### Multiple Canvases
In case you want to have multiple canvases on the same page, you can use the `CanvasManager` component instead:```csharp
@code{
CanvasManager _canvasManager;
protected override async Task OnAfterRenderAsync(bool firstRender)
{
if (!firstRender)
return;
_canvasManager.CreateCanvas("myCanvas", new CanvasCreationOptions()
{
Width = 800,
Height = 600,
Hidden = false,
OnCanvasReady = this.OnMyCanvasReady,
OnFrameReady = this.OnMyCanvasFrameReady,
});
}
}
```You simply have to get a reference to the `CanvasManager` and then call the `CreateCanvas` passing an instance of `CanvasCreationOptions` with the desired parameters.
For a complete list of options for Canvas initialization, see [here](https://github.com/mizrael/Blazorex/blob/master/src/Blazorex/CanvasCreationOptions.cs).
The [./samples](./samples) folder contains some examples of how to setup the canvas and draw some cool stuff :)
A sample game can be found here: [Blazeroids](https://github.com/mizrael/Blazeroids)