Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/mucsi96/react-use-api
React hook for fetching data.
https://github.com/mucsi96/react-use-api
Last synced: 5 days ago
JSON representation
React hook for fetching data.
- Host: GitHub
- URL: https://github.com/mucsi96/react-use-api
- Owner: mucsi96
- Created: 2020-06-14T20:14:05.000Z (over 4 years ago)
- Default Branch: master
- Last Pushed: 2021-05-11T19:52:04.000Z (over 3 years ago)
- Last Synced: 2024-10-12T03:12:40.076Z (about 1 month ago)
- Language: TypeScript
- Homepage:
- Size: 1.38 MB
- Stars: 0
- Watchers: 2
- Forks: 0
- Open Issues: 5
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# react-use-api
React hook for fetching data.
## Usage with ErrorBoundary
```typescript
import { useApi } from "./useApi";function useSearchByName(name: string) {
return useApi({
method: "GET",
url: `/api/search/${name}`
});
}const [names, search, loading] = useSearchByName(searchString);
```## Usage without ErrorBoundary
```typescript
import { useApi } from "./useApi";function useSearchByName(name: string) {
return useApi({
method: "GET",
url: `/api/search/${name}`,
noErrorPropagationBoundary: true
});
}const [names, search, loading, error] = useSearchByName(searchString);
```## Usage of ApiContextProvider
```typescript
export default function App() {
const apiContext = useMemo(
() => ({
enhanceRequest(request) {
request.headers.append(
"x-this-header",
"was-added-on-application-level"
);
return Promise.resolve(request);
},
postFetch(response, error) {
console.log("this log was made on application level", {
response,
error,
});
return Promise.resolve();
},
}),
[]
);
return (
);
}
```## Features
- Fetching data using fetchAPI
- Returning data, load function, loading state, error state
- Error handling for server and network errors
- Support for error boundaries
- Request cancelling on component unmount
- Request cancelling on prop change
- Request enhacing on application / context level. For example adding some headers
- Response processing on application / context level. For example logging errors.## Fetching data using fetchAPI
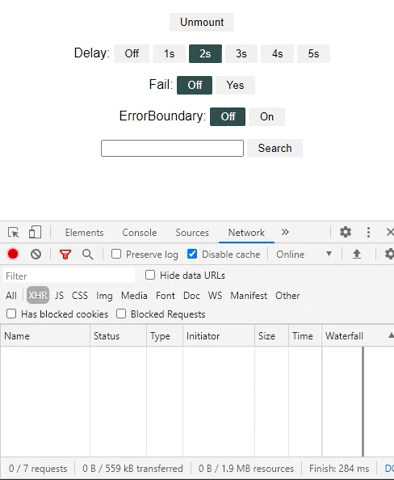
## Error handling for server errors
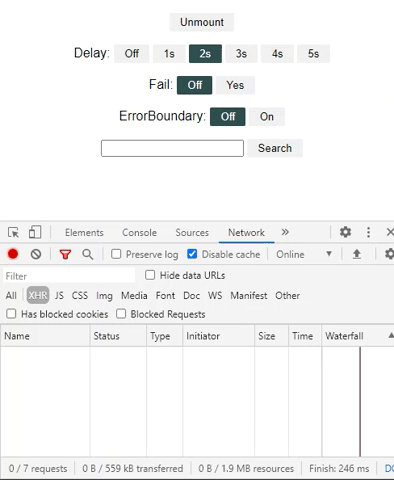
## Error handling for network errors
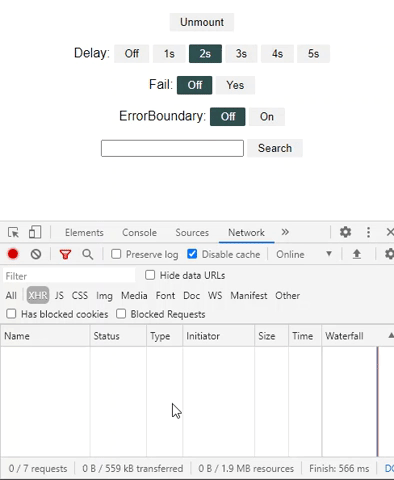
## Support for error boundaries
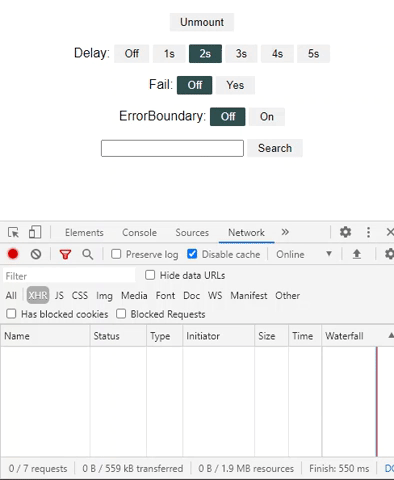
## Request cancelling on component unmount
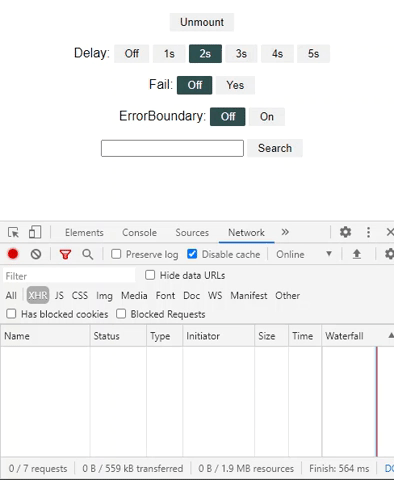
## Request cancelling on prop change
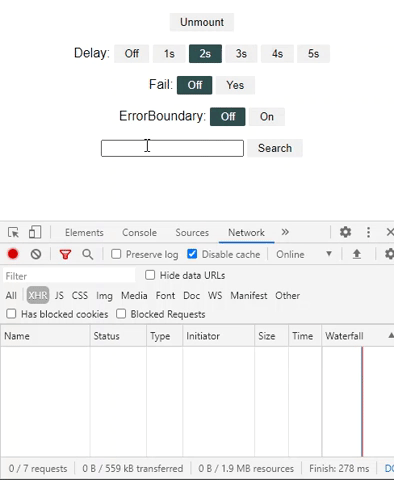
## Request enhacing on application / context level
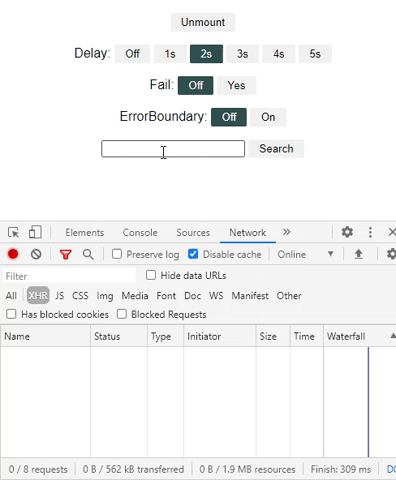
## Response processing on application / context level
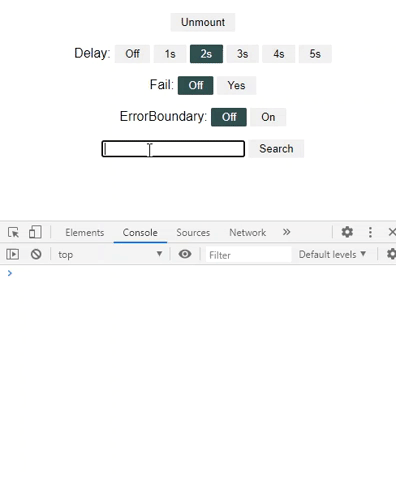