https://github.com/nabind47/fastapi
https://github.com/nabind47/fastapi
Last synced: 4 months ago
JSON representation
- Host: GitHub
- URL: https://github.com/nabind47/fastapi
- Owner: nabind47
- Created: 2023-08-21T12:26:19.000Z (almost 2 years ago)
- Default Branch: main
- Last Pushed: 2023-08-21T12:27:57.000Z (almost 2 years ago)
- Last Synced: 2025-01-17T20:13:30.110Z (6 months ago)
- Language: Python
- Size: 4.88 KB
- Stars: 0
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
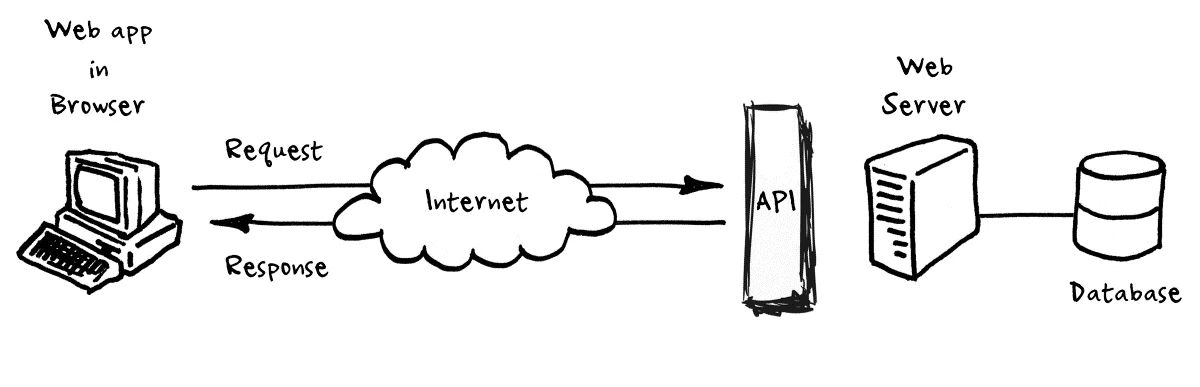
```sh
python -m venv venv
source venv/bin/activate
``````sh
pip install fastapi uvicornpip freeze > requirements.txt
pip install -r requirements.txt
``````py
from fastapi import FastAPIapp = FastAPI()
@app.get("/")
async def root():
return {"message": "Hello World"}
``````sh
uvicorn main:app --reload
```---
```py
from typing import Optional
from fastapi import FastAPI
from pydantic import BaseModelclass Item(BaseModel):
name: str
description: Optional[str] = None
price: float
tax: Optional[float] = Noneapp = FastAPI()
@app.post("/items/")
async def create_item(item: Item):
item_dict = item.dict()
if item.tax:
price_with_tax = item.price + item.tax
item_dict.update({"price_with_tax": price_with_tax})
return item_dict
``````py
from typing import Optional
from fastapi import FastAPI
from pydantic import BaseModelclass Item(BaseModel):
name: str
description: Optional[str] = None
price: float
tax: Optional[float] = Noneapp = FastAPI()
@app.put("/items/{item_id}")
async def create_item(item_id: int, item: Item):
return {"item_id": item_id, **item.dict()}```
```py
import pymongo
import json# Connect to MongoDB
client = pymongo.MongoClient("mongodb://localhost:27017/")
db = client["courses"]
collection = db["courses"]# Read courses from courses.json
with open("courses.json", "r") as f:
courses = json.load(f)# Create index for efficient retrieval
collection.create_index("name")# add rating field to each course
for course in courses:
course['rating'] = {'total': 0, 'count': 0}# add rating field to each chapter
for course in courses:
for chapter in course['chapters']:
chapter['rating'] = {'total': 0, 'count': 0}# Add courses to collection
for course in courses:
collection.insert_one(course)# Close MongoDB connection
client.close()
```> [**Documentation**](https://fastapi.tiangolo.com/tutorial/first-steps/)