Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/nelson-gon/pyautocv
(Semi) Automated Image Processing
https://github.com/nelson-gon/pyautocv
computer-vision edge-detection filters image-analysis image-classification image-clustering image-processing image-recognition image-segmentation imagedata laplace object-detection pypi python-package python3 segmentation sobel-filter thresholded-binary thresholding
Last synced: about 2 months ago
JSON representation
(Semi) Automated Image Processing
- Host: GitHub
- URL: https://github.com/nelson-gon/pyautocv
- Owner: Nelson-Gon
- License: mit
- Created: 2020-03-22T07:52:51.000Z (almost 5 years ago)
- Default Branch: master
- Last Pushed: 2022-08-10T20:08:31.000Z (over 2 years ago)
- Last Synced: 2024-10-08T05:21:51.267Z (3 months ago)
- Topics: computer-vision, edge-detection, filters, image-analysis, image-classification, image-clustering, image-processing, image-recognition, image-segmentation, imagedata, laplace, object-detection, pypi, python-package, python3, segmentation, sobel-filter, thresholded-binary, thresholding
- Language: Python
- Homepage: https://pyautocv.readthedocs.io/en/latest/
- Size: 34.4 MB
- Stars: 3
- Watchers: 2
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- Changelog: changelog.md
- Contributing: .github/CONTRIBUTING.md
- License: LICENSE
- Code of conduct: .github/CODE_OF_CONDUCT.md
Awesome Lists containing this project
README
# (Semi) Automated Image Processing with pyautocv
[](https://pypi.python.org/pypi/pyautocv/)
[](https://doi.org/10.5281/zenodo.3766956)


[](https://codecov.io/gh/Nelson-Gon/pyautocv?branch=master)
[](https://github.com/Nelson-Gon/pyautocv/blob/master/LICENSE)
[](https://pyautocv.readthedocs.io/en/latest/?badge=latest)
[](https://pypi.python.org/pypi/pyautocv/)
[](https://pepy.tech/project/pyautocv)
[](https://pepy.tech/project/pyautocv)
[](https://pepy.tech/project/pyautocv)
[](https://GitHub.com/Nelson-Gon/pyautocv/graphs/commit-activity)
[](https://github.com/Nelson-Gon/pyautocv/commits/master)
[](https://GitHub.com/Nelson-Gon/pyautocv/issues/)
[](https://GitHub.com/Nelson-Gon/pyautocv/issues?q=is%3Aissue+is%3Aclosed)**Project Aims**
The goal of `pyautocv` is to provide a simple computer vision(cv) workflow that enables one to automate
or at least reduce the time spent in image (pre)-processing.**Installing the package**
From PyPI
```shell
pip install pyautocv
```
From GitHub```shell
pip install git+https://github.com/Nelson-Gon/pyautocv.git
#or
# clone the repo
git clone https://www.github.com/Nelson-Gon/pyautocv.git
cd pyautocv
python3 setup.py install```
**Example Usage**
**Note**: Although these methods can be run via this script, the script is less flexible and might be useful for quick
exploration but not extended analysis.To run the script at the commandline, we can do the following
```shell
python -m pyautocv -d "images/cats" -s "png" -m "thresh_to_zero" -o "threshold" -mt 200 -t 100
```
Sample Result

To perform edge detection
```shell
python -m pyautocv -d "images/biology" -s "jpg" -o "detect_edges" -m "sobel_vertical" -k 3
```
To smooth images
```shell
python -m pyautocv -d "images/houses" -s "jpg" -o "smooth" -m "gaussian" -k 5 5 --sigma 0.7
```
To get help
```shell
python -m pyautocv -h
```
Further exploration is left to the user.
---
The following section shows how to use the more flexible class/methods approach
* Image Gra(e)ying
To grey an image directory
```python
from pyautocv.segmentation import Segmentation, gray_images, show_imagesimages_list=Segmentation("images/cats")
show_images(gray_images(images_list.read_images()), images_list.read_images(), number=2)```
* Smoothing
To smooth a directory of images, we can use `EdgeDetection`'s `smooth` method as
follows```python
images_list=Segmentation("images/cats")
show_images(images_list.smooth(), images_list.read_images(),number=2)```
This will give us

The above uses default parameters including an `rgb` color mode. For biological images which are often in
grayscale, one can set `color_mode` to gray as shown below. All other operations will remain the same.```python
images_list_gray_mode=Segmentation("images/dic", image_suffix ="tif", color_mode = "gray")
show_images(images_list_gray_mode.read_images(), images_list_gray_mode.threshold_images(), number = 4)
```Result

To use a different filter
```python
images_list = Segmentation("images/cats")
show_images(images_list.read_images(), images_list.smooth(mask="median", kernel_shape=(7, 7)))```

* Edge Detection
To detect edges in a directory of images, we can use `Segmentation`'s `detect_edges`.
```python
show_images(images_list.read_images(), images_list.detect_edges(operator="roberts", mask="gaussian", sigma=0.8))
```
The above will give us the following result

To use a different filter e.g Laplace,
```python
show_images(images_list.read_images(), images_list.detect_edges(operator="laplace", mask="gaussian", sigma=0))
```
This results in

* Thresholding
To perform thresholding, we can use the method `threshold_images`.
```python
to_threshold = Segmentation("images/biology")
show_images(to_threshold.read_images(),to_threshold.threshold_images())```

To use a different thresholding method.
```python
show_images(to_threshold.read_images(),to_threshold.threshold_images(threshold_method="otsu"))
```
The above gives us:

For cat lovers, here's thresholding with inverse binary.
```python
show_images(images_list.read_images(),images_list.threshold_images(threshold_method="binary_inverse"))
```
Result:
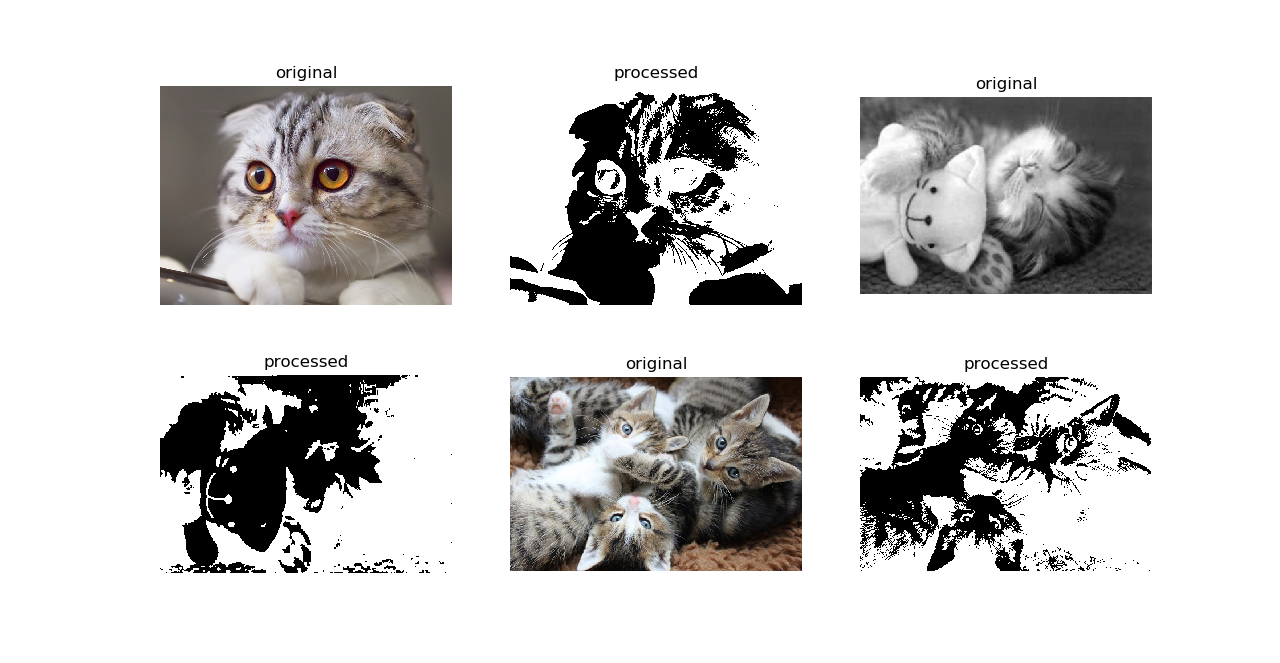
Thresholding applied to images of houses.
```python
images_list=Segmentation("images/houses")
show_images(images_list.read_images(), images_list.threshold_images(threshold_method="thresh_to_zero"))
```
```python
images_list=Segmentation("images/potholes")
show_images(images_list.read_images(), images_list.threshold_images("binary"))
```
These and more examples are available in [example.py](https://github.com/Nelson-Gon/pyautocv/blob/1bc67af448ea0bab00ea7223354619f7e9a5d42c/examples/example.py). Image sources are
shown in `sources.md`. If you feel attribution was not made, please file an issue and cite the violating image.**Citation**
Nelson Gonzabato(2021) pyautocv: (Semi) Automated Image Processing, https://github.com/Nelson-Gon/pyautocv.
```shell
@misc {Gonzabato2021,
author = {Gonzabato, N},
title = {pyautocv: (Semi) Automated Image Processing},
year = {2021},
publisher = {GitHub},
journal = {GitHub repository},
howpublished = {\url{https://github.com/Nelson-Gon/pyautocv}},
commit = {7fe2e0f7894e8be4588a22758e8097c247cd1cd9}```
>Thank you very much
> “A language that doesn't affect the way you think about programming is not worth knowing.”
― Alan J. Perlis---
**References**
* [Bebis](https://www.cse.unr.edu/~bebis/CS791E/Notes/EdgeDetection.pdf)
* [Standford, author unknown](https://ai.stanford.edu/~syyeung/cvweb/tutorial3.html)
* [Funkhouser et al.,2013](https://www.cs.princeton.edu/courses/archive/fall13/cos429/lectures/05-segmentation1)