https://github.com/nielspilgaard/pilgaard.backgroundjobs
A dotnet library for running background jobs in a scalable and performant manner. The jobs can trigger based on cron expressions, intervals or absolute datetime.
https://github.com/nielspilgaard/pilgaard.backgroundjobs
cronjob cronjob-schedule csharp dotnet dotnet-core dotnet-farmework dotnet-standard nuget recurring scheduled-tasks scheduling
Last synced: about 1 month ago
JSON representation
A dotnet library for running background jobs in a scalable and performant manner. The jobs can trigger based on cron expressions, intervals or absolute datetime.
- Host: GitHub
- URL: https://github.com/nielspilgaard/pilgaard.backgroundjobs
- Owner: NielsPilgaard
- License: apache-2.0
- Created: 2022-04-21T17:43:32.000Z (about 3 years ago)
- Default Branch: master
- Last Pushed: 2025-04-02T16:50:36.000Z (about 1 month ago)
- Last Synced: 2025-04-05T06:07:37.844Z (about 1 month ago)
- Topics: cronjob, cronjob-schedule, csharp, dotnet, dotnet-core, dotnet-farmework, dotnet-standard, nuget, recurring, scheduled-tasks, scheduling
- Language: C#
- Homepage:
- Size: 471 KB
- Stars: 54
- Watchers: 2
- Forks: 1
- Open Issues: 1
-
Metadata Files:
- Readme: README.md
- Contributing: .github/CONTRIBUTING.md
- Funding: .github/FUNDING.yml
- License: LICENSE
- Code of conduct: .github/CODE_OF_CONDUCT.md
Awesome Lists containing this project
README
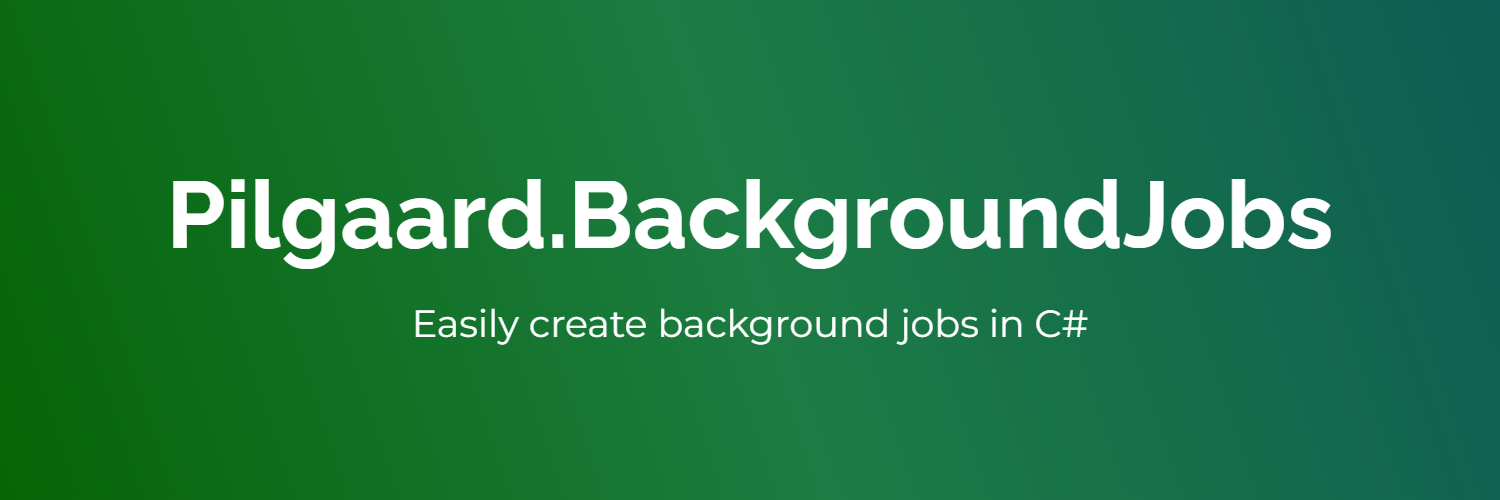
[](https://github.com/NielsPilgaard/Pilgaard.BackgroundJobs/actions/workflows/backgroundjobs_ci.yml)
[](https://www.nuget.org/packages/Pilgaard.BackgroundJobs)
[](https://www.nuget.org/packages/Pilgaard.BackgroundJobs)
A dotnet library for running background jobs in a scalable and performant manner.## Features
- Implement background jobs through interfaces
- Centralized host to manage and run jobs, keeping memory and thread usage low.
- Dependency Injection support
- Read and update job schedules at runtime through `IConfiguration` or `IOptionsMonitor`
- Monitoring jobs using logs and metrics, both compatible with OpenTelemetry## Scheduling Methods
- Cron expressions using `ICronJob`
- Recurringly at a set interval using `IRecurringJob`
- Recurringly at a set interval after an initial delay using `IRecurringJobWithInitialDelay`
- Once at an absolute time using `IOneTimeJob`## Use Case examples
- Sending emails
- Processing data
- Enforcing data retention# Getting Started
Make BackgroundJobs by implementing one of these interfaces:```csharp
public class CronJob : ICronJob
{
public Task RunJobAsync(CancellationToken cancellationToken = default)
{
Console.WriteLine("Time to backup your databases!");return Task.CompletedTask;
}
public CronExpression CronExpression => CronExpression.Parse("0 3 * * *");
}
```
```csharp
public class RecurringJob : IRecurringJob
{
public Task RunJobAsync(CancellationToken cancellationToken = default)
{
Console.WriteLine("This is your hourly reminder to stay hydrated.");return Task.CompletedTask;
}
public TimeSpan Interval => TimeSpan.FromHours(1);
}
```
```csharp
public class RecurringJobWithInitialDelay : IRecurringJobWithInitialDelay
{
public Task RunJobAsync(CancellationToken cancellationToken = default)
{
Console.WriteLine("This is your hourly reminder to stay hydrated.");return Task.CompletedTask;
}
public TimeSpan Interval => TimeSpan.FromHours(1);
public TimeSpan InitialDelay => TimeSpan.Zero;
}
```
```csharp
public class OneTimeJob : IOneTimeJob
{
public Task RunJobAsync(CancellationToken cancellationToken = default)
{
Console.WriteLine("Happy New Year!");return Task.CompletedTask;
}
public DateTime ScheduledTimeUtc => new(year: 2023, month: 12, day: 31, hour: 23, minute: 59, second: 59);
}
```# Registration
Call `AddBackgroundJobs()` on an `IServiceCollection`, and then add jobs:
```csharp
builder.Services.AddBackgroundJobs()
.AddJob()
.AddJob()
.AddJob()
.AddJob();
```You can also register jobs in-line for simple use cases:
```csharp
builder.Services.AddBackgroundJobs()
.AddJob(
name: "basic-cronjob",
job: () => {},
cronExpression: CronExpression.Parse("* * * * *"))
.AddJob(
name: "basic-recurringjob",
job: () => {},
interval: TimeSpan.FromSeconds(3))
.AddJob(
name: "basic-recurringjob-withinitialdelay",
job: () => {},
interval: TimeSpan.FromSeconds(3),
initialDelay: TimeSpan.Zero)
.AddJob(
name: "basic-onetimejob",
job: () => {},
scheduledTimeUtc: DateTime.UtcNow.AddHours(1))
.AddAsyncJob(
name: "async-cronjob",
job: cancellationToken => Task.CompletedTask,
cronExpression: CronExpression.Parse("* * * * *"))
.AddAsyncJob(
name: "async-recurringjob",
job: cancellationToken => Task.CompletedTask,
interval: TimeSpan.FromSeconds(3))
.AddAsyncJob(
name: "async-recurringjob-withinitialdelay",
job: cancellationToken => Task.CompletedTask,
interval: TimeSpan.FromSeconds(3),
initialDelay: TimeSpan.Zero)
.AddAsyncJob(
name: "async-onetimejob",
job: cancellationToken => Task.CompletedTask,
scheduledTimeUtc: DateTime.UtcNow.AddHours(1));
```# Samples
| Sample 🔗 | Tags |
| -- | -- |
| [BackgroundJobs.Configuration](https://github.com/NielsPilgaard/Pilgaard.BackgroundJobs/tree/master/samples/BackgroundJobs.Configuration) | ASP.NET, Reloading, Configuration
| [BackgroundJobs.MinimalAPI](https://github.com/NielsPilgaard/Pilgaard.BackgroundJobs/tree/master/samples/BackgroundJobs.MinimalAPI) | ASP.NET, MinimalAPI
| [BackgroundJobs.OpenTelemetry](https://github.com/NielsPilgaard/Pilgaard.BackgroundJobs/tree/master/samples/BackgroundJobs.OpenTelemetry) | ASP.NET, Open Telemetry, Metrics, Logs
| [BackgroundJobs.WorkerService](https://github.com/NielsPilgaard/Pilgaard.BackgroundJobs/tree/master/samples/BackgroundJobs.WorkerService) | Console, Worker Service---
# Open Telemetry Compatibility
Each project exposes histogram metrics, which allow monitoring the duration and count of jobs.
The meter names match the project names.
The [Open Telemetry Sample](https://github.com/NielsPilgaard/Pilgaard.BackgroundJobs/tree/master/samples/BackgroundJobs.OpenTelemetry) shows how to collect CronJob metrics using the Prometheus Open Telemetry exporter.
---
## Roadmap
- ~~Replace Assembly Scanning with registration similar to that of HealthChecks~~
- A separate UI project to help visualize when jobs trigger
- Utilize dotnet 8's new TimeProvider instead of `DateTime.UtcNow`
- More samples
- Using Blazor Server
- ~~Using a Worker Service~~
- Using IConfiguration to reload job schedule
- Using OneTimeJobs to control feature flags
- Using RecurringJobs to manage data retention---
## Thanks to
- The developers of [Cronos](https://github.com/HangfireIO/Cronos) for their excellent Cron expression library.
- JetBrains for providing me with a free license to their products, through their [Open Source Support program](https://jb.gg/OpenSourceSupport).