https://github.com/nikialeksey/jood
Object oriented jdbc wrapper
https://github.com/nikialeksey/jood
database elegant-objects elegantobjects eo-principles java java-8 java-library java8 jdbc library migrations object-oriented sql sql-database sql-migrations sql-queries
Last synced: 6 months ago
JSON representation
Object oriented jdbc wrapper
- Host: GitHub
- URL: https://github.com/nikialeksey/jood
- Owner: nikialeksey
- License: mit
- Created: 2019-06-16T06:42:01.000Z (about 6 years ago)
- Default Branch: master
- Last Pushed: 2022-08-30T11:09:38.000Z (almost 3 years ago)
- Last Synced: 2024-11-17T13:08:24.692Z (8 months ago)
- Topics: database, elegant-objects, elegantobjects, eo-principles, java, java-8, java-library, java8, jdbc, library, migrations, object-oriented, sql, sql-database, sql-migrations, sql-queries
- Language: Java
- Size: 129 KB
- Stars: 4
- Watchers: 4
- Forks: 1
- Open Issues: 1
-
Metadata Files:
- Readme: readme.md
- License: LICENSE
Awesome Lists containing this project
README
# Jood





[](https://maven-badges.herokuapp.com/maven-central/com.nikialeksey/jood)
[](https://travis-ci.org/nikialeksey/jood)
[](https://codecov.io/gh/nikialeksey/jood)[](https://github.com/nikialeksey/jood/blob/master/LICENSE)
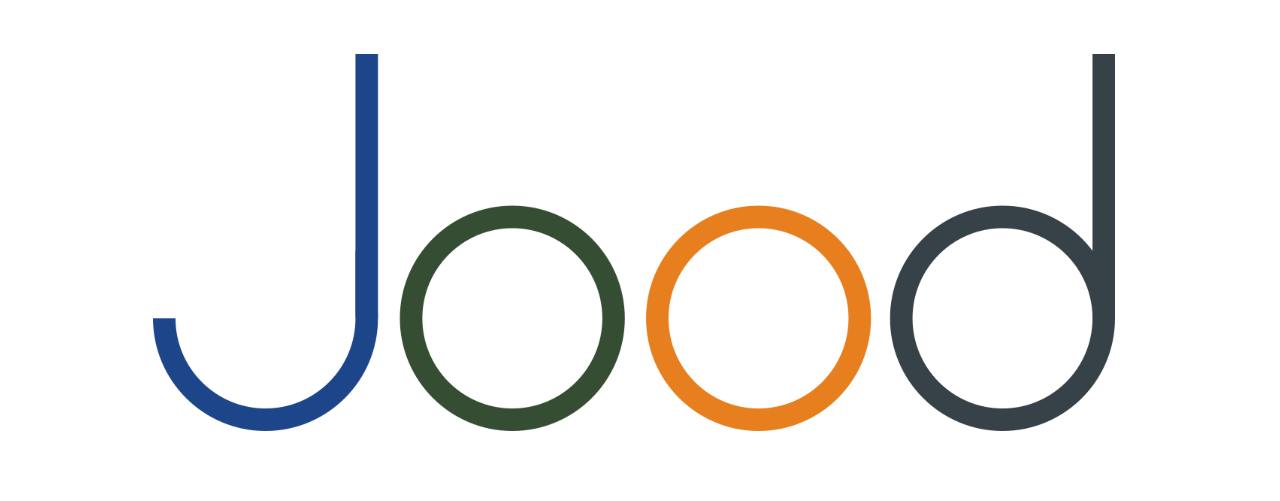
## What is it?
**Jood** is object oriented sql-database library written in Java.## Getting started
#### Gradle
```groovy
implementation 'com.nikialeksey:jood:x.y.z'
```#### Maven
```xmlcom.nikialeksey
jood
x.y.z```
Where `x.y.z` actual version from
[](https://maven-badges.herokuapp.com/maven-central/com.nikialeksey/jood)### Connection
```java
Connection connection = DriverManager.getConnection(...);
Db db = new JdDb(() -> connection);
```For example, connect to `sqlite` in-memory:
```java
Connection connection = DriverManager.getConnection(
"jdbc:sqlite::memory:"
);
Db db = new JdDb(() -> connection);
```### Data source
```java
DataSource ds = ...;
Db db = new JdDb(ds);
```For example, connect to `h2` in-memory through the `c3p0` pooled data source:
```java
ComboPooledDataSource ds = new ComboPooledDataSource();
ds.setJdbcUrl("jdbc:h2:mem:db_name");
Db db = new JdDb(ds);
```### Simple queries
```java
Db db = ...;
db.write(new JdSql("CREATE TABLE a (n INTEGER NOT NULL)"));
db.write(new JdSql("INSERT INTO a(n) VALUES(5)"));
try (
QueryResult qr = db.read(new JdSql("SELECT * FROM a"))
) {
ResultSet rs = qr.rs();
while (rs.next()) {
String n = rs.getString("n");
}
}
```### Query arguments
```java
db.write(
new JdSql(
"INSERT INTO a(n) VALUES(?)",
new IntArg(5)
)
);
```### Transactions
```java
Db db = ...;
db.run(() -> { // all changes inside will be commited after successfull execution
db.run(() -> { // transactions could be inner
for (int i = 0; i < 1000; i++) {
db.write(new JdSql("INSERT INTO a(n) VALUES(1)"));
}
});
for (int i = 0; i < 3000; i++) {
db.write(new JdSql("INSERT INTO a(n) VALUES(2)"));
}
});
```### Migrations
```java
Db origin = ...;
Db db = new MigrationsDb(
origin,
new JdMigrations(
new Migration() {
@Override
public int number() {
return 0; // migration index
}@Override
public void execute(final Db db) throws JbException {
db.write(
new JdSql(
"CREATE TABLE user (name TEXT NOT NULL)"
)
);
}
},
new Migration() {
@Override
public int number() {
return 1; // migration index
}@Override
public void execute(final Db db) throws JbException {
db.write(
new JdSql(
"ALTER TABLE user " +
"ADD lastname TEXT NOT NULL DEFAULT ''"
)
);
}
}
),
2 // db version number
)
```