https://github.com/nitin42/react-handy-renderer
✏️ Draw 2D primitives in sketchy style with React
https://github.com/nitin42/react-handy-renderer
canvas colors primitives react shapes sketch
Last synced: 3 months ago
JSON representation
✏️ Draw 2D primitives in sketchy style with React
- Host: GitHub
- URL: https://github.com/nitin42/react-handy-renderer
- Owner: nitin42
- Created: 2018-02-12T08:26:01.000Z (over 7 years ago)
- Default Branch: master
- Last Pushed: 2018-02-12T12:09:03.000Z (over 7 years ago)
- Last Synced: 2025-03-17T23:02:53.360Z (3 months ago)
- Topics: canvas, colors, primitives, react, shapes, sketch
- Language: JavaScript
- Size: 52.7 KB
- Stars: 60
- Watchers: 4
- Forks: 3
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# react-handy-renderer
> ***✏️ Draw 2D primitives in sketchy style with React***
![]()
## Motivation
[Theory on design and effects of sketchy rendering](http://openaccess.city.ac.uk/1274/)
## Demo
You can find the demo [here](https://codesandbox.io/s/nrpq071nwm) on CodeSandbox (switch on "module view" on the top right and go to /demo)
## Install
```
npm install react-handy-renderer
```## Usage
`react-handy-renderer` exposes a set of **five** primitives:
* **Rectangle**
* **RoundedRect**
* **Line**
* **Curve**
* **Ellipse**and one wrapper component called **Paper** which serves as a canvas on which these primitives are drawn.
Here is a basic example -
```js
import React from 'react';
import { render, Paper, Ellipse } from 'react-handy-renderer';function Sketch() {
return (
);
}render(, document.getElementById('canvas-container'));
```## Examples
### RoundedRect

```js
```
### Rectangle
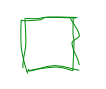
```js
```
### Ellipse
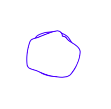
```js
```
### Line

```js
```
### Curve
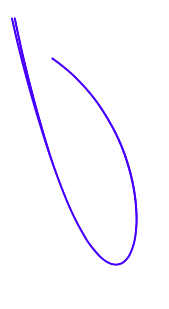
```js
```
## Usage with ReactDOM renderer
You can also render the primitives when working with ReactDOM as this just renders to a canvas node.
Example -
```js
import React, { Component } from 'react'
import {
render as create,
Paper,
Rectangle,
Main,
Ellipse,
Line,
RoundedRect,
Curve,
} from 'react-handy-renderer'function Sketch() {
return (
)
}// Renders the canvas node
create(, document.getElementById('root'))// create function has already rendered the canvas node.
// So now you can continue to use React with ReactDOM as you used to.
// Make changes directly to the component.
class App extends Component {
render() {
return (
REACT HANDY RENDERER
)
}
}export default App
```## API
### Paper
Wrapper component for creating the canvas.
**Props**
* `width` - width of the canvas
* `height` - height of the canvas
* `roughness` - Number value for changing the roughness of shapes
* `bowing` - Number value for changing the bowing of lines
* `maxOffset` - Number value for giving coordinates an offset,
[Example](#usage)
### Curve
**Props**
* `c1` - First control point's `x` and `y` coordinate values
* `c2` - Second control point's `x` and `y` coordinate values
* `c3` - Third control point's `x` and `y` coordinate values
* `c4` - Fourth control point's `x` and `y` coordinate values
* `roughness` - Number value for changing the roughness
* `bowing` - Number value for changing the bowing of lines
* `maxOffset` - Number value for giving coordinates an offset,
* `color` - String value for color
[Example](#curve)
### Ellipse
**Props**
* `position` - It is an object that takes `x` and `y` coordinate values.
* `width` - defines width
* `height` - defines height
* `color` - sets the color for stroke
* `weight` - sets the stroke thickness
* `roughness` - Number value for changing the roughness
* `bowing` - Number value for changing the bowing of lines
* `maxOffset` - Number value for giving coordinates an offset,
* `fill` - sets the fill color for filling the ellipse
[Example](#ellipse)
### Rectangle
**Props**
* `position` - It is an object that takes `x` and `y` coordinate values.
* `width` - defines width
* `height` - defines height
* `color` - sets the color for stroke
* `weight` - sets the stroke thickness
* `roughness` - Number value for changing the roughness
* `bowing` - Number value for changing the bowing of lines
* `maxOffset` - Number value for giving coordinates an offset,
[Example](#rectangle)
### RoundedRect
**Props**
* `position` - It is an object that takes `x` and `y` coordinate values.
* `width` - defines width
* `height` - defines height
* `radius` - sets the radius
* `color` - sets the color for stroke
* `weight` - sets the stroke thickness
* `roughness` - Number value for changing the roughness
* `bowing` - Number value for changing the bowing of lines
* `maxOffset` - Number value for giving coordinates an offset,
[Example](roundedrect)
### Line
**props**
* `from` - An object that takes `x1` and `y1` coordinate value.
* `to` - An object that takes `x2` and `y2` value
* `color` - sets the color for stroke
* `weight` - sets the stroke thickness
* `roughness` - Number value for changing the roughness
* `bowing` - Number value for changing the bowing of lines
* `maxOffset` - Number value for giving coordinates an offset,
[Example](#line)
## Todo
- [ ] Define hachures
- [ ] More examples