https://github.com/npm-dom/domquery
jQuery-like handy DOM manipulation library composed from small modules in NPM.
https://github.com/npm-dom/domquery
Last synced: 7 months ago
JSON representation
jQuery-like handy DOM manipulation library composed from small modules in NPM.
- Host: GitHub
- URL: https://github.com/npm-dom/domquery
- Owner: npm-dom
- Created: 2013-06-16T14:46:17.000Z (about 12 years ago)
- Default Branch: master
- Last Pushed: 2016-04-09T04:18:54.000Z (about 9 years ago)
- Last Synced: 2024-08-11T19:28:53.768Z (11 months ago)
- Language: JavaScript
- Homepage: http://npm.im/domquery
- Size: 65.4 KB
- Stars: 84
- Watchers: 6
- Forks: 8
- Open Issues: 5
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
## DomQuery
jQuery-like handy DOM manipulation library composed from small modules.
Example:
```js
var dom = require('domquery')dom('ul.songs:last-child')
.add('
.show()
```
## Install
```bash
$ npm install domquery
```
## Usage
Recommended to use [browserify](http://github.com/substack/node-browserify) for bundling for client-side. Sorry, it does not work in Node.
### Selecting
```js
var dom = require('domquery')
dom('body .foo .bar')
// => [array, of, elements]
dom('.foo', '.bar', '.qux').select('*')
// [all children of .foo, .bar, .qux]
dom('.foo', '.bar', '.qux').parent()
// [parent elements of .foo, .bar, .qux]
dom('.foo', '.bar', '.qux').siblings('button.tweet')
// [all siblings that matches "button.tweet"]
```
Details: [dom-select](https://github.com/npm-dom/dom-select), [siblings](http://github.com/npm-dom/siblings), [closest](https://github.com/component/closest)
### Changing Style
```js
var dom = require('domquery')
dom('body .foo .bar')
.style('background-color', 'red')
// OR
.style({
'padding': '10px',
'margin': '10px'
})
```
Other available Methods:
* show
* hide
Details: [dom-style](https://github.com/npm-dom/dom-style)
### Adding and Removing Elements
domquery embeds [dom-tree](http://github.com/npm-dom) to provide following methods;
#### .insert(parent element)
Insert an element to a parent element.
```js
var dom = require('domquery')
dom('
{title}
.insert('body')
```
#### .add(child)
Add a new element to specified parent element.
```js
dom('body > ul')
.add('
```
Or;
```js
var row = dom('
dom('body > ul').add(row)
```
* `child` can be an element, array, selector or HTML.
#### .addBefore(child, reference)
Adds `child` before `reference`
```js
dom('ul.songs')
.addBefore('
```
* `child` can be an element, array, selector or HTML.
* `reference` can be an element, array or selector.
#### .addAfter(child, reference)
Adds `child` after `reference`
```js
dom('ul.songs')
.addAfter('
```
* `child` can be an element, array, selector or HTML.
* `reference` can be an element, array or selector.
#### .replace(target, replacement)
Replaces `target` with `replacement`
```js
dom('ul.songs')
.replace('li:first-child', document.createElement('textarea'))
```
or:
```js
dom('ul.songs')
.replace('li:first-child', '
```
#### .remove(element)
```js
dom('ul .songs').remove('li:first-child')
```
### Inline CSS
Methods: addClass, hasClass, removeClass, toggleClass
Example:
```js
var dom = require('domquery')
dom('body').addClass('foobar')
dom('body').hasClass('foobar')
// => true
dom('body').removeClass('foobar')
dom('body').hasClass('foobar')
// => false
dom('body').toggleClass('foobar')
dom('body').hasClass('foobar')
// => true
```
Other Available Methods:
* addClass
* hasClass
* removeClass
* toggleClass
Details: [dom-classes](https://github.com/npm-dom/dom-classes)
### Events
domquery embeds [dom-event](http://github.com/npm-dom/dom-event), [key-event](http://github.com/npm-dom/key-event) and [delegate-dom](http://github.com/npm-dom/delegate-dom) modules to provide following methods;
#### .on(event, callback)
Add a new event
```js
var dom = require('domquery')
dom('body').on('click', function (event) {
console.log('clicked body')
})
```
Shortcuts:
```js
dom('ul li').click(function (event) {
console.log('clicked a "li"')
})
```
* change
* click
* keydown
* keyup
* keypress
* mousedown
* mouseover
* mouseup
* resize
##### .off(event, callback)
Remove the event listener;
```js
dom('body').off('click', fn)
```
#### .on(event, selector, callback)
[Delegate event](http://github.com/npm-dom/delegate-dom) handler function for `selector`:
```js
dom('body ul').on('click', 'li', function (event) {
console.log('clicked a list item!')
})
```
#### .onKey(event, callback)
Adds a [keyboard event](http://github.com/npm-dom/key-event):
```js
dom('input').onKey('alt a', function (event) {
console.log('user pressed alt + a')
})
```
#### .offKey(event, callback)
Removes a [keyboard event](http://github.com/npm-dom/key-event):
```js
dom('input').onKey('alt a', altA)
dom('input').offKey('alt a', altA)
function altA (event) {
console.log('user pressed alt + a')
}
```
### Attributes
```js
var dom = require('domquery')
dom('a.my-link').attr('href')
// => http://foobar.com
dom('a').attr('href', 'http://foobar.com')
```
### Content
Reading:
```js
dom('.foo').html() // equivalent of `innerHTML`
dom('input.my-input').val() // equivalent of `value`
```
Setting:
```js
dom('.foo').html('
dom('input.my-input').val('new value')
```
More info about it is at [dom-value](http://github.com/npm-dom/dom-value)
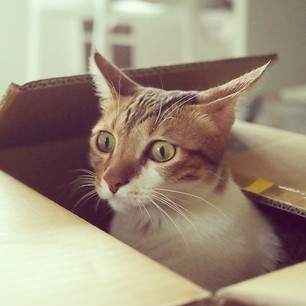