Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/obsfx/dungrain
BSP based procedural dungeon generation package.
https://github.com/obsfx/dungrain
dungeon generation procedural procedural-generation rogue roguelike typescript
Last synced: 2 months ago
JSON representation
BSP based procedural dungeon generation package.
- Host: GitHub
- URL: https://github.com/obsfx/dungrain
- Owner: obsfx
- License: wtfpl
- Created: 2020-01-15T18:31:58.000Z (almost 5 years ago)
- Default Branch: master
- Last Pushed: 2022-06-22T23:35:04.000Z (over 2 years ago)
- Last Synced: 2024-10-02T08:51:07.213Z (3 months ago)
- Topics: dungeon, generation, procedural, procedural-generation, rogue, roguelike, typescript
- Language: JavaScript
- Homepage: https://github.com/obsfx/dungrain
- Size: 4.28 MB
- Stars: 4
- Watchers: 3
- Forks: 0
- Open Issues: 4
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
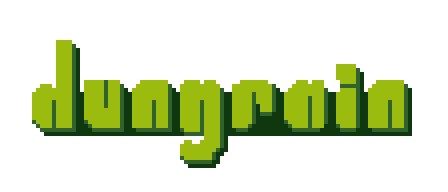
# dungrain [](https://badge.fury.io/js/dungrain)
dungrain is a procedural dungeon generation library that was built on binary space partitioning method. It takes some arguments as an object and creates 2D number array that contains types of tiles that placed based on `indexMap` (`Wall`, `Path`, `Room`, `Empty`) values.
## installation
You can directly use `dungrain.js` or `dungrain.min.js` file with script tag. They can be found in build folder at github repo.
```html
let dungeon = new dungrain({
iterationCount: 20,
column: 100,
row: 40,
indexMap: {
Wall: 3,
Path: 2,
Room: 1,
Empty: 0
}
});```
or you can install with npm.
```
npm install dungrain
``````javascript
const dungrain = require('dungrain');let dungeon = new dungrain({
iterationCount: 20,
column: 100,
row: 40,
indexMap: {
Wall: 3,
Path: 2,
Room: 1,
Empty: 0
}
});
```## usage
##### arguments
All arguments have to be passed into class as an object.
```
{
iterationCount: number,
column: number,
row: number,
indexMap: {
Wall: number,
Path: number,
Room: number,
Empty: number
}
seed: string (optional) (default: it will be generated randomly),
minimumWHRatio: number (optional) (default: 0.5),
maximumWHRatio: number (optional) (default: 2.0),
minimumChunkWidth: number (optional) (default: 8),
minimumChunkHeight: number (optional) (default: 8)
}
```##### methods
```javascript
getMap() // (returns generated dungeon that formed into 2D number array)
getAllFloors() // (returns all available floors included rooms and paths)
getRooms() // (returns all room objects that contains their topleftcorner point and all available floors array)
getPaths() // (returns all path objects that contains their start point, all available floors array data, direction data (0: VERTICAL, 1: HORIZONTAL) and width value (represents the length of the path))
getSeed() // (returns the seed of the generated dungeon)
```## example
##### code
```html
let canvas = document.querySelector('.canvas');
let ctx = canvas.getContext('2d');
let dungeon = new dungrain({
seed: 'example',
iterationCount: 6,
column: 100,
row: 50,
indexMap: {
Wall: 3,
Path: 2,
Room: 1,
Empty: 0
},
minimumWHRatio: 0.8,
maximumWHRatio: 1.6,
minimumChunkWidth: 2,
minimumChunkHeight: 2
});let map = dungeon.getMap();
for (let i = 0; i < map.length; i++) {
for (let j = 0; j < map[i].length; j++) {
let tile = map[i][j];if (tile == dungeon.indexMap.Wall) {
ctx.fillStyle = 'black';
} else if (tile == dungeon.indexMap.Path) {
ctx.fillStyle = 'blue';
} else if (tile == dungeon.indexMap.Room) {
ctx.fillStyle = 'green';
} else {
ctx.fillStyle = 'white';
}ctx.fillRect(j * 10, i * 10, 10, 10);
}
}```
##### output
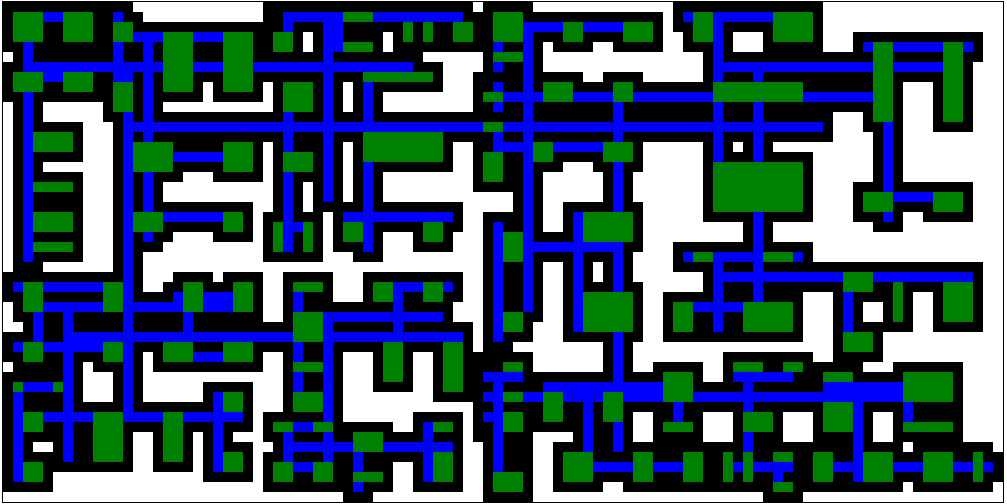
##### see in action @codepen
i made a codepen visualization demo with early version of this. You can play with that from here: https://codepen.io/omercanbalandi/pen/JjoeKrQ
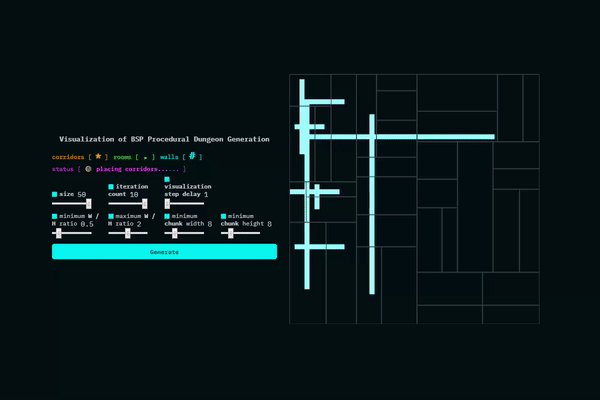