https://github.com/optuna/optuna
A hyperparameter optimization framework
https://github.com/optuna/optuna
distributed hacktoberfest hyperparameter-optimization machine-learning parallel python
Last synced: 6 days ago
JSON representation
A hyperparameter optimization framework
- Host: GitHub
- URL: https://github.com/optuna/optuna
- Owner: optuna
- License: mit
- Created: 2018-02-21T06:12:56.000Z (about 7 years ago)
- Default Branch: master
- Last Pushed: 2024-10-29T09:40:45.000Z (6 months ago)
- Last Synced: 2024-10-29T11:46:59.589Z (6 months ago)
- Topics: distributed, hacktoberfest, hyperparameter-optimization, machine-learning, parallel, python
- Language: Python
- Homepage: https://optuna.org
- Size: 18.8 MB
- Stars: 10,795
- Watchers: 117
- Forks: 1,027
- Open Issues: 68
-
Metadata Files:
- Readme: README.md
- Contributing: CONTRIBUTING.md
- Funding: .github/FUNDING.yml
- License: LICENSE
- Code of conduct: CODE_OF_CONDUCT.md
Awesome Lists containing this project
- my-awesome-starred - optuna/optuna - A hyperparameter optimization framework (Python)
- awesome-llmops - Optuna - square) | (AutoML / Profiling)
- StarryDivineSky - optuna/optuna
- my-awesome - optuna/optuna - optimization,machine-learning,parallel,python pushed_at:2025-04 star:11.7k fork:1.1k A hyperparameter optimization framework (Python)
- awesome-sciml - optuna/optuna: A hyperparameter optimization framework
- awesome-list - Optuna - An automatic hyperparameter optimization software framework, particularly designed for machine learning. (Machine Learning Framework / Hyperparameter Search & Gradient-Free Optimization)
- awesome-production-machine-learning - Optuna - Optuna is an automatic hyperparameter optimisation software framework, particularly designed for machine learning. (AutoML)
- awesome-python-machine-learning-resources - GitHub - 7% open · ⏱️ 26.08.2022): (超参数优化和AutoML)
- awesomeLibrary - optuna - A hyperparameter optimization framework (语言资源库 / python)
README
# Optuna: A hyperparameter optimization framework
[](https://www.python.org)
[](https://pypi.python.org/pypi/optuna)
[](https://anaconda.org/conda-forge/optuna)
[](https://github.com/optuna/optuna)
[](https://optuna.readthedocs.io/en/stable/)
[](https://codecov.io/gh/optuna/optuna):link: [**Website**](https://optuna.org/)
| :page_with_curl: [**Docs**](https://optuna.readthedocs.io/en/stable/)
| :gear: [**Install Guide**](https://optuna.readthedocs.io/en/stable/installation.html)
| :pencil: [**Tutorial**](https://optuna.readthedocs.io/en/stable/tutorial/index.html)
| :bulb: [**Examples**](https://github.com/optuna/optuna-examples)
| [**Twitter**](https://twitter.com/OptunaAutoML)
| [**LinkedIn**](https://www.linkedin.com/showcase/optuna/)
| [**Medium**](https://medium.com/optuna)*Optuna* is an automatic hyperparameter optimization software framework, particularly designed
for machine learning. It features an imperative, *define-by-run* style user API. Thanks to our
*define-by-run* API, the code written with Optuna enjoys high modularity, and the user of
Optuna can dynamically construct the search spaces for the hyperparameters.## :loudspeaker: News
* **Mar 24, 2025**: A new article [Distributed Optimization in Optuna and gRPC Storage Proxy](https://medium.com/optuna/distributed-optimization-in-optuna-and-grpc-storage-proxy-08db83f1d608) has been published.
* **Mar 11, 2025**: A new article [[Optuna v4.2] Gaussian Process-Based Sampler Can Now Handle Inequality Constraints](https://medium.com/optuna/optuna-v4-2-gaussian-process-based-sampler-can-now-handle-inequality-constraints-a4f68e8ee810) has been published.
* **Feb 17, 2025**: A new article [SMAC3 Registered on OptunaHub](https://medium.com/optuna/smac3-registered-on-optunahub-4fb9e90855cb) has been published.
* **Jan 22, 2025**: A new article [OptunaHub Benchmarks: A New Feature to Use/Register Various Benchmark Problems](https://medium.com/optuna/optunahub-benchmarks-a-new-feature-to-use-register-various-benchmark-problems-694401524ce0) has been published.
* **Jan 20, 2025**: Optuna 4.2.0 and OptunaHub 0.2.0 are out! Try the newest Optuna and OptunaHub! Check out [the release note](https://github.com/optuna/optuna/releases/tag/v4.2.0) for details.
* **Jan 16, 2025**: A new article [Overview of Python Free Threading (v3.13t) Support in Optuna](https://medium.com/optuna/overview-of-python-free-threading-v3-13t-support-in-optuna-ad9ab62a11ba) has been published.## :fire: Key Features
Optuna has modern functionalities as follows:
- [Lightweight, versatile, and platform agnostic architecture](https://optuna.readthedocs.io/en/stable/tutorial/10_key_features/001_first.html)
- Handle a wide variety of tasks with a simple installation that has few requirements.
- [Pythonic search spaces](https://optuna.readthedocs.io/en/stable/tutorial/10_key_features/002_configurations.html)
- Define search spaces using familiar Python syntax including conditionals and loops.
- [Efficient optimization algorithms](https://optuna.readthedocs.io/en/stable/tutorial/10_key_features/003_efficient_optimization_algorithms.html)
- Adopt state-of-the-art algorithms for sampling hyperparameters and efficiently pruning unpromising trials.
- [Easy parallelization](https://optuna.readthedocs.io/en/stable/tutorial/10_key_features/004_distributed.html)
- Scale studies to tens or hundreds of workers with little or no changes to the code.
- [Quick visualization](https://optuna.readthedocs.io/en/stable/tutorial/10_key_features/005_visualization.html)
- Inspect optimization histories from a variety of plotting functions.## Basic Concepts
We use the terms *study* and *trial* as follows:
- Study: optimization based on an objective function
- Trial: a single execution of the objective functionPlease refer to the sample code below. The goal of a *study* is to find out the optimal set of
hyperparameter values (e.g., `regressor` and `svr_c`) through multiple *trials* (e.g.,
`n_trials=100`). Optuna is a framework designed for automation and acceleration of
optimization *studies*.Sample code with scikit-learn
[](http://colab.research.google.com/github/optuna/optuna-examples/blob/main/quickstart.ipynb)
```python
import ...# Define an objective function to be minimized.
def objective(trial):# Invoke suggest methods of a Trial object to generate hyperparameters.
regressor_name = trial.suggest_categorical('regressor', ['SVR', 'RandomForest'])
if regressor_name == 'SVR':
svr_c = trial.suggest_float('svr_c', 1e-10, 1e10, log=True)
regressor_obj = sklearn.svm.SVR(C=svr_c)
else:
rf_max_depth = trial.suggest_int('rf_max_depth', 2, 32)
regressor_obj = sklearn.ensemble.RandomForestRegressor(max_depth=rf_max_depth)X, y = sklearn.datasets.fetch_california_housing(return_X_y=True)
X_train, X_val, y_train, y_val = sklearn.model_selection.train_test_split(X, y, random_state=0)regressor_obj.fit(X_train, y_train)
y_pred = regressor_obj.predict(X_val)error = sklearn.metrics.mean_squared_error(y_val, y_pred)
return error # An objective value linked with the Trial object.
study = optuna.create_study() # Create a new study.
study.optimize(objective, n_trials=100) # Invoke optimization of the objective function.
```> [!NOTE]
> More examples can be found in [optuna/optuna-examples](https://github.com/optuna/optuna-examples).
>
> The examples cover diverse problem setups such as multi-objective optimization, constrained optimization, pruning, and distributed optimization.## Installation
Optuna is available at [the Python Package Index](https://pypi.org/project/optuna/) and on [Anaconda Cloud](https://anaconda.org/conda-forge/optuna).
```bash
# PyPI
$ pip install optuna
``````bash
# Anaconda Cloud
$ conda install -c conda-forge optuna
```> [!IMPORTANT]
> Optuna supports Python 3.8 or newer.
>
> Also, we provide Optuna docker images on [DockerHub](https://hub.docker.com/r/optuna/optuna).## Integrations
Optuna has integration features with various third-party libraries. Integrations can be found in [optuna/optuna-integration](https://github.com/optuna/optuna-integration) and the document is available [here](https://optuna-integration.readthedocs.io/en/stable/index.html).
Supported integration libraries
* [Catboost](https://github.com/optuna/optuna-examples/tree/main/catboost/catboost_pruning.py)
* [Dask](https://github.com/optuna/optuna-examples/tree/main/dask/dask_simple.py)
* [fastai](https://github.com/optuna/optuna-examples/tree/main/fastai/fastai_simple.py)
* [Keras](https://github.com/optuna/optuna-examples/tree/main/keras/keras_integration.py)
* [LightGBM](https://github.com/optuna/optuna-examples/tree/main/lightgbm/lightgbm_integration.py)
* [MLflow](https://github.com/optuna/optuna-examples/tree/main/mlflow/keras_mlflow.py)
* [PyTorch](https://github.com/optuna/optuna-examples/tree/main/pytorch/pytorch_simple.py)
* [PyTorch Ignite](https://github.com/optuna/optuna-examples/tree/main/pytorch/pytorch_ignite_simple.py)
* [PyTorch Lightning](https://github.com/optuna/optuna-examples/tree/main/pytorch/pytorch_lightning_simple.py)
* [TensorBoard](https://github.com/optuna/optuna-examples/tree/main/tensorboard/tensorboard_simple.py)
* [TensorFlow](https://github.com/optuna/optuna-examples/tree/main/tensorflow/tensorflow_estimator_integration.py)
* [tf.keras](https://github.com/optuna/optuna-examples/tree/main/tfkeras/tfkeras_integration.py)
* [Weights & Biases](https://github.com/optuna/optuna-examples/tree/main/wandb/wandb_integration.py)
* [XGBoost](https://github.com/optuna/optuna-examples/tree/main/xgboost/xgboost_integration.py)## Web Dashboard
[Optuna Dashboard](https://github.com/optuna/optuna-dashboard) is a real-time web dashboard for Optuna.
You can check the optimization history, hyperparameter importance, etc. in graphs and tables.
You don't need to create a Python script to call [Optuna's visualization](https://optuna.readthedocs.io/en/stable/reference/visualization/index.html) functions.
Feature requests and bug reports are welcome!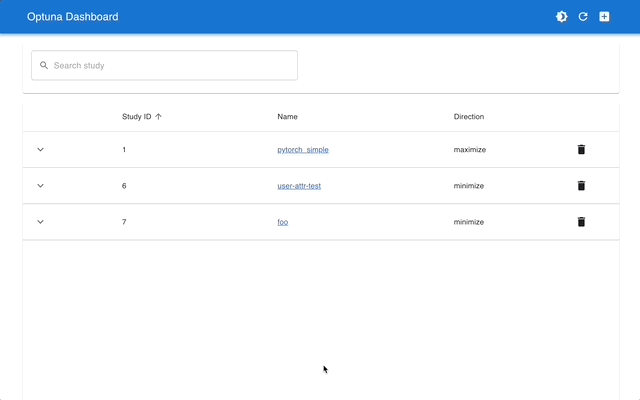
`optuna-dashboard` can be installed via pip:
```shell
$ pip install optuna-dashboard
```> [!TIP]
> Please check out the convenience of Optuna Dashboard using the sample code below.Sample code to launch Optuna Dashboard
Save the following code as `optimize_toy.py`.
```python
import optunadef objective(trial):
x1 = trial.suggest_float("x1", -100, 100)
x2 = trial.suggest_float("x2", -100, 100)
return x1 ** 2 + 0.01 * x2 ** 2study = optuna.create_study(storage="sqlite:///db.sqlite3") # Create a new study with database.
study.optimize(objective, n_trials=100)
```Then try the commands below:
```shell
# Run the study specified above
$ python optimize_toy.py# Launch the dashboard based on the storage `sqlite:///db.sqlite3`
$ optuna-dashboard sqlite:///db.sqlite3
...
Listening on http://localhost:8080/
Hit Ctrl-C to quit.
```## OptunaHub
[OptunaHub](https://hub.optuna.org/) is a feature-sharing platform for Optuna.
You can use the registered features and publish your packages.### Use registered features
`optunahub` can be installed via pip:
```shell
$ pip install optunahub
# Install AutoSampler dependencies (CPU only is sufficient for PyTorch)
$ pip install cmaes scipy torch --extra-index-url https://download.pytorch.org/whl/cpu
```You can load registered module with `optunahub.load_module`.
```python
import optuna
import optunahubdef objective(trial: optuna.Trial) -> float:
x = trial.suggest_float("x", -5, 5)
y = trial.suggest_float("y", -5, 5)
return x**2 + y**2module = optunahub.load_module(package="samplers/auto_sampler")
study = optuna.create_study(sampler=module.AutoSampler())
study.optimize(objective, n_trials=10)print(study.best_trial.value, study.best_trial.params)
```For more details, please refer to [the optunahub documentation](https://optuna.github.io/optunahub/).
### Publish your packages
You can publish your package via [optunahub-registry](https://github.com/optuna/optunahub-registry).
See the [OptunaHub tutorial](https://optuna.github.io/optunahub-registry/index.html).## Communication
- [GitHub Discussions] for questions.
- [GitHub Issues] for bug reports and feature requests.[GitHub Discussions]: https://github.com/optuna/optuna/discussions
[GitHub issues]: https://github.com/optuna/optuna/issues## Contribution
Any contributions to Optuna are more than welcome!
If you are new to Optuna, please check the [good first issues](https://github.com/optuna/optuna/labels/good%20first%20issue). They are relatively simple, well-defined, and often good starting points for you to get familiar with the contribution workflow and other developers.
If you already have contributed to Optuna, we recommend the other [contribution-welcome issues](https://github.com/optuna/optuna/labels/contribution-welcome).
For general guidelines on how to contribute to the project, take a look at [CONTRIBUTING.md](./CONTRIBUTING.md).
## Reference
If you use Optuna in one of your research projects, please cite [our KDD paper](https://doi.org/10.1145/3292500.3330701) "Optuna: A Next-generation Hyperparameter Optimization Framework":
BibTeX
```bibtex
@inproceedings{akiba2019optuna,
title={{O}ptuna: A Next-Generation Hyperparameter Optimization Framework},
author={Akiba, Takuya and Sano, Shotaro and Yanase, Toshihiko and Ohta, Takeru and Koyama, Masanori},
booktitle={The 25th ACM SIGKDD International Conference on Knowledge Discovery \& Data Mining},
pages={2623--2631},
year={2019}
}
```## License
MIT License (see [LICENSE](./LICENSE)).
Optuna uses the codes from SciPy and fdlibm projects (see [LICENSE_THIRD_PARTY](./LICENSE_THIRD_PARTY)).