Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/pdfme/pdfme
Open-source PDF generation library built with TypeScript and React. Features a WYSIWYG template designer, PDF viewer, and powerful generation capabilities. Create custom PDFs effortlessly in both browser and Node.js environments.
https://github.com/pdfme/pdfme
pdf pdf-designer pdf-generation pdf-generator pdf-lib pdf-library pdf-viewer react typescript
Last synced: 5 days ago
JSON representation
Open-source PDF generation library built with TypeScript and React. Features a WYSIWYG template designer, PDF viewer, and powerful generation capabilities. Create custom PDFs effortlessly in both browser and Node.js environments.
- Host: GitHub
- URL: https://github.com/pdfme/pdfme
- Owner: pdfme
- License: mit
- Created: 2021-08-22T08:45:56.000Z (over 3 years ago)
- Default Branch: main
- Last Pushed: 2024-10-26T07:41:05.000Z (3 months ago)
- Last Synced: 2024-10-29T11:58:56.189Z (3 months ago)
- Topics: pdf, pdf-designer, pdf-generation, pdf-generator, pdf-lib, pdf-library, pdf-viewer, react, typescript
- Language: TypeScript
- Homepage: http://pdfme.com/
- Size: 154 MB
- Stars: 2,606
- Watchers: 18
- Forks: 239
- Open Issues: 52
-
Metadata Files:
- Readme: README.md
- Funding: .github/FUNDING.yml
- License: LICENSE.md
Awesome Lists containing this project
- awesome-github-star - pdfme
README
# PDFME
![]()
Website |
pdfme Cloud |
DiscordTypeScript-based PDF generator and React-based UI. Open source, developed by the community, and completely free to use under the MIT license!
## Features
| Fast PDF Generator | Easy PDF Template Design | Simple JSON Template |
| ---------------------------------------------------------------------------------------------- | ----------------------------------------------------- | -------------------------------------------------------------- |
| Works on Node and browser. Use templates to generate PDFs; complex operations are not needed. | Anyone can easily create templates with the designer. | Templates are JSON data that is easy to understand and handle. |---
## Documentation
**For the complete documentation of pdfme, please refer to [Getting Started](https://pdfme.com/docs/getting-started).**
## Examples Using pdfme
If you are looking for code examples using pdfme to get started, please check out the [pdfme-playground website](https://playground.pdfme.com/) and the [playground source code](https://github.com/pdfme/pdfme/tree/main/playground). Setting these up is covered in the [DEVELOPMENT.md](DEVELOPMENT.md) file.
## Cloud Service Option
While pdfme is a powerful open-source library, we understand that some users might prefer a managed solution. For those looking for a ready-to-use, scalable PDF generation service without the need for setup and maintenance, we offer pdfme Cloud.
**[Try pdfme Cloud - Hassle-free PDF Generation](https://app.pdfme.com?utm_source=github&utm_content=readme-cloud)**
pdfme Cloud provides all the features of the open-source library, plus:
- PDF generation at scale without infrastructure management
- Hosted WYSIWYG template designer
- Simple API integration
- Automatic updates and maintenance\*pdfme is and will always remain open-source. The cloud service is an optional offering for those who prefer a managed solution.
## Sponsors
Support this project by becoming a sponsor. Your logo will show up here with a link to your website.
| [](https://github.com/ProgressLabIT) | [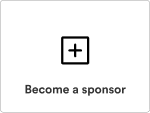](https://github.com/sponsors/pdfme) |
| :------------------------------------------------------------------------------------------------------------: | :-----------------------------------------------------------------------------------------------------------------------------------------------: |
| [ProgressLab](https://github.com/ProgressLabIT) | [New Sponsor](https://github.com/sponsors/pdfme) |## Special Thanks
- [pdf-lib](https://pdf-lib.js.org/): Used in PDF generation.
- [fontkit](https://github.com/foliojs/fontkit): Used in font rendering.
- [PDF.js](https://mozilla.github.io/pdf.js/): Used in PDF viewing.
- [React](https://reactjs.org/): Used in building the UI.
- [form-render](https://xrender.fun/form-render): Used in building the UI.
- [antd](https://ant.design/): Used in building the UI.
- [react-moveable](https://daybrush.com/moveable/), [react-selecto](https://github.com/daybrush/selecto), [@scena/react-guides](https://daybrush.com/guides/): Used in Designer UI.
- [dnd-kit](https://github.com/clauderic/dnd-kit): Used in Designer UI.
- [Lucide](https://lucide.dev/): Used in Designer UI and Schema's icon.I definitely could not have created pdfme without these libraries. I am grateful to the developers of these libraries.
If you want to contribute to pdfme, please check the [Development Guide](https://pdfme.com/docs/development-guide) page.
We look forward to your contribution!