https://github.com/ph-fritsche/liform
Transform Symfony Forms into JSON Schema
https://github.com/ph-fritsche/liform
forms json-schema symfony-bundle symfony-form
Last synced: 2 months ago
JSON representation
Transform Symfony Forms into JSON Schema
- Host: GitHub
- URL: https://github.com/ph-fritsche/liform
- Owner: ph-fritsche
- License: mit
- Created: 2020-05-04T09:23:25.000Z (about 5 years ago)
- Default Branch: master
- Last Pushed: 2021-07-21T10:00:22.000Z (almost 4 years ago)
- Last Synced: 2025-03-25T06:41:32.539Z (3 months ago)
- Topics: forms, json-schema, symfony-bundle, symfony-form
- Language: PHP
- Homepage: https://ph-fritsche.github.io/liform
- Size: 536 KB
- Stars: 3
- Watchers: 0
- Forks: 0
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
[](https://codecov.io/gh/ph-fritsche/liform)
# 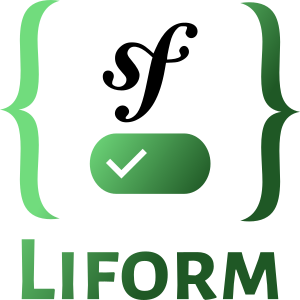
Library for transforming [Symfony Form Views](https://symfony.com/doc/current/components/form.html) into JSON.
It is developed to be used with [liform-react-final](https://www.npmjs.com/package/liform-react-final), but can be used with any form rendering supporting [JSON schema](http://json-schema.org/).
## Installation
Install per [Composer](https://getcomposer.org/) from [Packagist](https://packagist.org/packages/pitch/liform).
```
composer require pitch/liform
```If you execute this inside a Symfony application with [Symfony Flex](https://symfony.com/doc/current/setup/flex.html),
`LiformInterface` will be available per [Dependency Injection](https://symfony.com/doc/current/service_container.html#injecting-services-config-into-a-service) right away.## Basic usage
```php
$form = \Symfony\Component\Form\Forms::createFormFactory()->create();
$form->add('foo', \Symfony\Component\Form\Extension\Core\Type\TextType::class);
$form->add('bar', \Symfony\Component\Form\Extension\Core\Type\NumberType::class);/* ... handle the request ... */
$resolver = new \Pitch\Liform\Resolver();
$resolver->setTransformer('text', new \Pitch\Liform\Transformer\StringTransformer());
$resolver->setTransformer('number', new \Pitch\Liform\Transformer\NumberTransformer());
/* ... */$liform = new \Pitch\Liform\Liform($resolver);
$liform->addExtension(new \Pitch\Liform\Extension\ValueExtension());
$liform->addExtension(new \Pitch\Liform\Extension\LabelExtension());
/* ... */return $liform->transform($form->createView());
```### Inside a Symfony application
```php
namespace App\Controller;/* use statements */
class MyFormController extends Symfony\Bundle\FrameworkBundle\Controller\AbstractController
{
protected LiformInterface $liform;/* Let the service container inject the service */
public function __construct(LiformInterface $liform)
{
$this->liform = $liform;
}public function __invoke(Request $request)
{
$form = $this->createForm(MyFormType::class);
$form->handleRequest($request);
if ($form->isSubmitted() && $form->isValid()) {
/* ... do something ... */
} else {
return new Response($this->render('my_form.html.twig', [
'liform' => $this->liform->transform($form->createView()),
]), $form->isSubmitted() ? 400 : 200);
}
}
}
```### The result
The `TransformResult` is an object that when passed through `json_encode()` will produce something like:
```js
{
"schema": {
"title": "form",
"type": "object",
"properties": {
"foo": {
"title": "foo",
"type": "string"
},
"bar": {
"title": "bar",
"type": "number",
}
},
"required": [
"foo",
"bar"
]
},
"meta": {
"errors": {
"foo": ["This is required."]
}
},
"values": {
"bar": 42
}
}
```## Acknowledgements
This library is based on [Limenius/Liform](https://github.com/Limenius/Liform).
Its technique for transforming forms using resolvers and reducers is inspired by [Symfony Console Form](https://github.com/matthiasnoback/symfony-console-form).