https://github.com/phenax/plasmajs
An isomorphic nodeJS framework based on react
https://github.com/phenax/plasmajs
es2015 isomorphic-nodejs-framework nodejs react
Last synced: 8 months ago
JSON representation
An isomorphic nodeJS framework based on react
- Host: GitHub
- URL: https://github.com/phenax/plasmajs
- Owner: phenax
- License: apache-2.0
- Archived: true
- Created: 2016-11-05T11:57:19.000Z (over 8 years ago)
- Default Branch: master
- Last Pushed: 2020-09-04T00:55:00.000Z (almost 5 years ago)
- Last Synced: 2024-11-14T21:52:31.847Z (8 months ago)
- Topics: es2015, isomorphic-nodejs-framework, nodejs, react
- Language: JavaScript
- Size: 490 KB
- Stars: 134
- Watchers: 6
- Forks: 12
- Open Issues: 14
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# PlasmaJS
[](https://travis-ci.org/phenax/plasmajs)
[](https://www.npmjs.com/package/plasmajs)
[](https://github.com/phenax/plasmajs/blob/master/LICENSE)
[](https://david-dm.org/phenax/plasmajs)
[](https://discord.gg/b9Z4b6r)[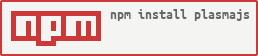](https://www.npmjs.com/package/plasmajs)
An isomorphic NodeJS framework powered with React for building web apps.
**[Use the starter-kit to get up and running with PlasmaJS](https://github.com/phenax/plasmajs-starter-kit) [OUTDATED]**
**[Join us on discord](https://discord.gg/b9Z4b6r)**
[Getting Started](https://github.com/ManuelPortero/plasmajs/blob/ManuelPortero-patch-1/docs/gettingStarted.md)
[Middlewares](https://github.com/ManuelPortero/plasmajs/blob/ManuelPortero-patch-1/docs/middlewares.md)
[Routes](https://github.com/ManuelPortero/plasmajs/blob/ManuelPortero-patch-1/docs/routes.md)
## Features
* Declarative syntax
* Isomorphic routing
* Isolated routing for API endpoints
* Middlewares that are maintanable
* ES6 syntax with babel
## Installation
* Install with npm `npm i --save plasmajs`(you can also install it globally with `npm i -g plasmajs`)
* To run the server, `plasmajs path/to/server.js`(Add it to your package.json scripts for local install)
## Guide
### Import it to your app.js
```javascript
import { Server, Route, Router, NodeHistoryAPI } from 'plasmajs';
```### Writing A Server
```javascript
const HeadLayout = props => (
{props.title}
);
const WrapperLayout = props => {props.children};
const HomeLayout = props =>Hello World;
const ErrorLayout = props =>404 Not Found;export default class App extends React.Component {
// Port number (Default=8080)
static port = 8080;render() {
const history = new NodeHistoryAPI(this.props.request, this.props.response);
return (
);
}
}
```
### Middlewares
#### Writing custom Middlewares
In MyMiddleWare.jsx...
```javascript
import { MiddleWare } from 'plasmajs';export default class MyMiddleWare extends MiddleWare {
onRequest(req, res) {
// Do your magic here
// Run this.terminate() to stop the render and take over
}
}
```And in App's render method...
```html
// ...
```
#### Logger Middleware
It logs information about the request made to the server out to the console.
````javascript
import {Logger} from 'plasmajs' // and add it in the server but after the router declaration.
````
```html// ...
```
* Props
* `color` (boolean) - Adds color to the logs if true
#### StaticContentRouter Middleware
Allows you to host a static content directory for public files
````javascript
import {StaticContentRouter} from 'plasmajs'
````
```html
// ...
```
* Props
* `dir` (string) - The name of the static content folder to host
* `hasPrefix` (boolean) - If set to false, will route it as http://example.com/file instead of http://example.com/public/file
* `compress` (boolean) - If true, will enable gzip compression on all static content if the client supports it
#### APIRoute Middleware
Allows you to declare isolated routes for requests to api hooks
```javascript
import {APIRoute} from 'plasmajs'
//...
// API request handler for api routes
_apiRequestHandler() {// Return a promise
return new Promise((resolve, reject) => {resolve({
wow: "cool cool"
});
});
}render() {
return (
//...
);
}
```* Props
* `method` (string) - The http request method
* `path` (string or regex) - The path to match
* `controller` (function) - The request handler
### Routing
Isomorphic routing which renders the content on the server-side and then lets the javascript kick in and take over the interactions. (The server side rendering only works for Push State routing on the client side, not Hash routing).
`NOTE: Its better to isolate the route definitions to its own file so that the client-side and the server-side can share the components`
#### History API
There are 3 types of routing available - Backend routing(`new NodeHistoryAPI(request, response)`), Push State routing(`new HistoryAPI(options)`), Hash routing(`new HashHistoryAPI(options)`)(NOTE: The naming is just for consistency)
#### The Router
```html
{allRouteDeclarations}
```
* Props
* `history` (object) - It's the history api instance you pass in depending on the kind of routing you require.
* `wrapper` (React component class) - It is a wrapper for the routed contents
#### Declaring a route
If `Homepage` is a react component class and `/` is the url.
```html
```
* Props
* `path` (string or regex) - The url to route the request to
* `component` (React component class) - The component to be rendered when the route is triggered
* `statusCode` (integer) - The status code for the response
* `caseInsensitive` (boolean) - Set to true if you want the url to be case insensitive
* `errorHandler` (boolean) - Set to true to define a 404 error handler## Want to help?
PRs are welcome. You can also join us on [discord](https://discord.gg/b9Z4b6r) for discussions about plasmajs.